What are the best practices for writing high-performance Go code?
Writing high-performance Go code involves adhering to several best practices that can significantly enhance the efficiency and speed of your applications. Here are some key practices to consider:
- Use Appropriate Data Structures: Choose the right data structure for your use case. For example, use slices instead of arrays when the size is dynamic, and consider using maps for fast lookups. Go's built-in data structures are optimized for performance.
-
Minimize Allocations: Reducing memory allocations can lead to better performance. Use object pools to reuse memory, and prefer stack allocations over heap allocations where possible. The
sync.Pool
type can be useful for managing a pool of temporary objects. - Avoid Unnecessary Synchronization: Overuse of synchronization primitives like mutexes can lead to performance bottlenecks. Use them judiciously and consider using channels for communication between goroutines, which are more lightweight.
-
Profile and Optimize: Regularly profile your code to identify bottlenecks. Use tools like
pprof
to understand where your application spends most of its time and optimize those areas. - Leverage Go's Compiler Optimizations: The Go compiler is capable of performing various optimizations. Write idiomatic Go code to take advantage of these optimizations. For instance, inlining small functions can reduce function call overhead.
-
Use Efficient I/O Operations: I/O operations can be a major performance bottleneck. Use buffered I/O operations and consider using
io.Copy
for efficient data transfer between readers and writers. - Optimize for CPU Caches: Write code that is cache-friendly. This includes aligning data to cache line boundaries and minimizing cache misses by keeping frequently accessed data together.
- Avoid Reflection: While reflection is powerful, it comes with a performance cost. Use it sparingly and only when necessary.
By following these best practices, you can write Go code that is not only efficient but also maintainable and scalable.
What specific Go language features can enhance code performance?
Go offers several language features that can be leveraged to enhance code performance:
- Goroutines and Channels: Go's lightweight concurrency model allows for efficient parallel execution of tasks. Goroutines are cheap to create and manage, and channels provide a safe and efficient way to communicate between them.
-
Defer: The
defer
statement can be used to ensure that resources are released in a timely manner, which can help in managing system resources efficiently and avoiding memory leaks. - Interfaces: Go's interfaces allow for polymorphism without the overhead of traditional object-oriented languages. They enable writing generic code that can work with different types, reducing code duplication and improving performance.
- Slices: Slices are more flexible and efficient than arrays for managing collections of data. They allow for dynamic resizing and efficient memory usage.
- Maps: Go's built-in map type provides fast lookups and is optimized for performance. It's an excellent choice for scenarios requiring key-value storage.
- Struct Embedding: Embedding structs allows for composition and can lead to more efficient code by reducing the need for explicit delegation.
- Inlined Functions: The Go compiler can inline small functions, reducing the overhead of function calls. Writing small, focused functions can take advantage of this optimization.
- Escape Analysis: Go's escape analysis can determine whether a variable should be allocated on the stack or the heap. Writing code that encourages stack allocation can improve performance.
By understanding and utilizing these features effectively, developers can write Go code that is both performant and idiomatic.
How can concurrency be optimized in Go to improve application speed?
Optimizing concurrency in Go can significantly improve application speed by leveraging the language's built-in support for parallel execution. Here are some strategies to optimize concurrency:
-
Use Appropriate Number of Goroutines: Creating too many goroutines can lead to context switching overhead. Use a suitable number of goroutines based on the workload and available resources. The
runtime.GOMAXPROCS
function can help in setting the maximum number of CPUs that can be used simultaneously. - Minimize Goroutine Creation Overhead: Goroutines are lightweight, but creating them still incurs some overhead. Use goroutine pools or reuse goroutines where possible to minimize this overhead.
-
Efficient Use of Channels: Channels are a powerful tool for communication between goroutines. Use buffered channels to reduce blocking and improve throughput. Also, consider using
select
statements to handle multiple channels efficiently. - Avoid Unnecessary Synchronization: Overuse of synchronization primitives like mutexes can lead to performance bottlenecks. Use channels for communication where possible, as they are more lightweight and can help in avoiding deadlocks.
-
Use
sync.WaitGroup
for Coordination: Thesync.WaitGroup
type is useful for waiting for a collection of goroutines to finish executing. It's more efficient than using channels for this purpose in many cases. -
Leverage
sync.Pool
for Object Reuse: Usesync.Pool
to manage a pool of temporary objects, reducing the need for frequent allocations and garbage collection. -
Profile and Optimize Concurrency: Use profiling tools like
pprof
to identify concurrency-related bottlenecks. Optimize based on the insights gained from profiling. - Consider Work Stealing: Go's scheduler uses work stealing to balance the load across goroutines. Writing code that can take advantage of this can lead to better performance.
By applying these strategies, you can optimize concurrency in Go, leading to faster and more efficient applications.
What tools and techniques are recommended for profiling and optimizing Go code?
Profiling and optimizing Go code is crucial for improving performance. Here are some recommended tools and techniques:
-
pprof: The
pprof
tool is part of the Go standard library and is used for profiling Go programs. It can generate CPU, memory, and other types of profiles. Usego tool pprof
to analyze the profiles and identify performance bottlenecks. -
Go Benchmarking: Use Go's built-in benchmarking tools to measure the performance of specific functions or code snippets. The
go test -bench
command can run benchmarks and provide detailed performance metrics. -
Trace: The
trace
tool can help in understanding the execution flow of your program, including goroutine scheduling and blocking events. Usego tool trace
to analyze trace data and optimize concurrency-related issues. -
Memory Profiling: Use the
runtime.ReadMemStats
function to gather memory usage statistics. This can help in identifying memory leaks and optimizing memory usage. -
Flame Graphs: Flame graphs are a visual representation of stack traces and can be generated using
pprof
. They help in quickly identifying which functions are consuming the most CPU time. -
Go's Escape Analysis: Use the
-gcflags="-m"
option with thego build
command to see the escape analysis output. This can help in understanding why certain variables are allocated on the heap and how to optimize them for stack allocation. -
Third-Party Tools: Tools like
Delve
for debugging,gops
for monitoring Go processes, andgo-torch
for generating flame graphs can provide additional insights and help in optimizing Go code. - Code Review and Optimization: Regularly review your code for potential optimizations. Look for opportunities to reduce allocations, improve algorithms, and use more efficient data structures.
By using these tools and techniques, you can effectively profile and optimize your Go code, leading to significant performance improvements.
The above is the detailed content of What are the best practices for writing high-performance Go code?. For more information, please follow other related articles on the PHP Chinese website!
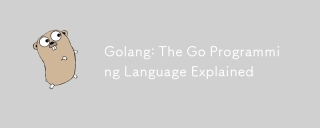
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
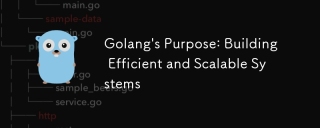
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
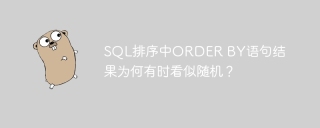
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
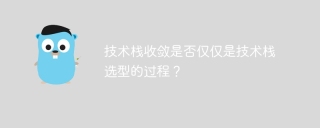
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...
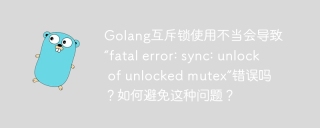
Golang ...
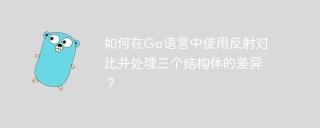
How to compare and handle three structures in Go language. In Go programming, it is sometimes necessary to compare the differences between two structures and apply these differences to the...
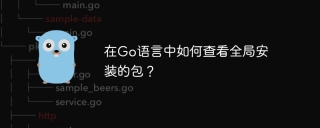
How to view globally installed packages in Go? In the process of developing with Go language, go often uses...
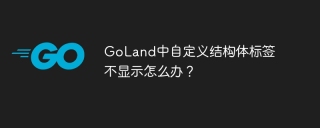
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
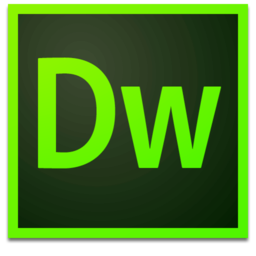
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor