How can you optimize Go code for specific hardware architectures?
Optimizing Go code for specific hardware architectures involves several strategies that can significantly enhance performance. Here are some key approaches:
-
Use of SIMD Instructions: Many modern CPUs support SIMD (Single Instruction, Multiple Data) instructions, which can perform the same operation on multiple data points simultaneously. Go's standard library does not directly support SIMD, but you can use assembly or external libraries like
github.com/mmcloughlin/avo
to leverage these instructions. For example, on x86 architectures, you can use SSE or AVX instructions to speed up operations on large datasets. -
Memory Alignment: Proper memory alignment can improve performance, especially on architectures that penalize misaligned memory access. Go's runtime generally handles alignment well, but for critical sections, you might need to use
unsafe
package to ensure proper alignment. - Cache Optimization: Understanding and optimizing for the CPU cache hierarchy can lead to significant performance gains. Techniques include data locality, loop tiling, and cache blocking. For instance, you can structure your data to fit within the L1 or L2 cache, reducing the need for slower memory accesses.
- Branch Prediction: Modern CPUs use branch prediction to improve performance. Writing code that is predictable can help. In Go, this might mean avoiding complex conditional statements or using techniques like loop unrolling to reduce branches.
- Compiler Optimizations: The Go compiler has various optimizations that can be enabled or tuned for specific architectures. Using compiler flags (which we'll discuss later) can help target these optimizations.
- Use of Assembly: For the most critical parts of your code, using assembly language can provide direct access to hardware-specific instructions. This is particularly useful for operations that the Go compiler might not optimize well.
By applying these techniques, you can tailor your Go code to take full advantage of the capabilities of specific hardware architectures.
What are the best practices for using Go's assembly language to enhance performance on different CPU architectures?
Using Go's assembly language to enhance performance requires careful consideration and adherence to best practices. Here are some key guidelines:
- Identify Critical Sections: Only use assembly for the most performance-critical parts of your code. The overhead of switching between Go and assembly can negate any benefits if used excessively.
- Understand the Target Architecture: Different CPU architectures have different instruction sets and optimizations. For example, x86 has SSE and AVX, while ARM has NEON. Ensure you're using the appropriate instructions for your target architecture.
-
Use Go's Assembly Syntax: Go uses a specific assembly syntax that is different from traditional assembly languages. Familiarize yourself with this syntax, which is documented in the Go wiki. For example, registers are prefixed with
$
, and labels are suffixed with:
. -
Integrate with Go Code: Use the
go:asm
directive to include assembly files in your Go project. Ensure that you correctly define the function signatures to match the Go calling convention. - Test and Benchmark: Thoroughly test and benchmark your assembly code. Use Go's built-in testing and benchmarking tools to ensure that your optimizations actually improve performance.
- Maintainability: Assembly code can be harder to maintain than Go code. Document your assembly code well and consider the long-term maintainability of your project.
-
Use Libraries: For common operations, consider using libraries that provide optimized assembly implementations, such as
github.com/minio/sha256-simd
for SHA-256 hashing.
By following these best practices, you can effectively use Go's assembly language to enhance performance on different CPU architectures.
How can profiling tools help in identifying hardware-specific optimizations for Go programs?
Profiling tools are essential for identifying areas of your Go program that can benefit from hardware-specific optimizations. Here's how they can help:
-
CPU Profiling: Tools like
pprof
can generate CPU profiles that show where your program spends most of its time. By analyzing these profiles, you can identify functions or loops that are CPU-intensive and might benefit from hardware-specific optimizations like SIMD instructions or better cache utilization. - Memory Profiling: Memory profiling can help you understand how your program uses memory. This is crucial for optimizing for cache hierarchies. By identifying memory-intensive operations, you can restructure your data to improve cache performance.
- Trace Profiling: Go's trace tool can provide a detailed view of the execution flow, including goroutine scheduling and blocking events. This can help you identify synchronization points that might be optimized for specific hardware.
-
Hardware Counters: Some profiling tools can access hardware performance counters, which provide detailed metrics on CPU events like cache misses, branch mispredictions, and instruction counts. Tools like
perf
on Linux can be used in conjunction with Go's profiling to gather these metrics. -
Benchmarking: While not strictly a profiling tool, benchmarking is crucial for measuring the impact of your optimizations. Go's
testing
package includes benchmarking capabilities that can help you quantify performance improvements.
By using these profiling tools, you can pinpoint the parts of your Go program that are most likely to benefit from hardware-specific optimizations, allowing you to focus your efforts where they will have the most impact.
Which Go compiler flags should be used to target optimizations for particular hardware architectures?
The Go compiler provides several flags that can be used to target optimizations for specific hardware architectures. Here are some of the most relevant flags:
-
-cpuprofile
: This flag generates a CPU profile that can be used to identify performance bottlenecks. While not directly an optimization flag, it's crucial for understanding where optimizations might be beneficial. -
-gcflags
: This flag allows you to pass options to the Go compiler. For example, you can use-gcflags="-l"
to disable inlining, which can be useful for debugging or when you want to manually control inlining for specific functions. -
-ldflags
: This flag allows you to pass options to the linker. For example,-ldflags="-s -w"
can strip debug information and reduce the binary size, which can be beneficial for performance on resource-constrained hardware. -
-race
: This flag enables the race detector, which can help identify data races that might affect performance on multi-core systems. -
-msan
: This flag enables memory sanitizer, which can help identify memory-related issues that might impact performance. -
-buildmode
: This flag allows you to specify the build mode. For example,-buildmode=pie
can generate position-independent executables, which can be beneficial for security and performance on some systems. -
-asmflags
: This flag allows you to pass options to the assembler. For example,-asmflags="-D GOOS_linux"
can define assembly-time constants, which can be used to conditionally include or exclude assembly code based on the target OS. -
-tags
: This flag allows you to specify build tags, which can be used to include or exclude code based on specific conditions. For example, you might use-tags=avx2
to include AVX2-specific optimizations.
By using these compiler flags, you can fine-tune the compilation process to target optimizations for particular hardware architectures, ensuring that your Go programs are as efficient as possible.
The above is the detailed content of How can you optimize Go code for specific hardware architectures?. For more information, please follow other related articles on the PHP Chinese website!
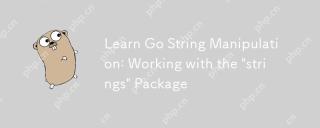
Go's "strings" package provides rich features to make string operation efficient and simple. 1) Use strings.Contains() to check substrings. 2) strings.Split() can be used to parse data, but it should be used with caution to avoid performance problems. 3) strings.Join() is suitable for formatting strings, but for small datasets, looping = is more efficient. 4) For large strings, it is more efficient to build strings using strings.Builder.
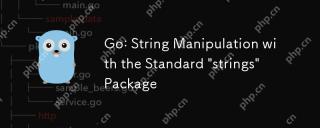
Go uses the "strings" package for string operations. 1) Use strings.Join function to splice strings. 2) Use the strings.Contains function to find substrings. 3) Use the strings.Replace function to replace strings. These functions are efficient and easy to use and are suitable for various string processing tasks.
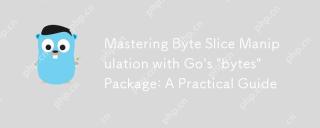
ThebytespackageinGoisessentialforefficientbyteslicemanipulation,offeringfunctionslikeContains,Index,andReplaceforsearchingandmodifyingbinarydata.Itenhancesperformanceandcodereadability,makingitavitaltoolforhandlingbinarydata,networkprotocols,andfileI
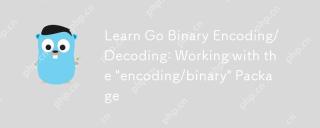
Go uses the "encoding/binary" package for binary encoding and decoding. 1) This package provides binary.Write and binary.Read functions for writing and reading data. 2) Pay attention to choosing the correct endian (such as BigEndian or LittleEndian). 3) Data alignment and error handling are also key to ensure the correctness and performance of the data.
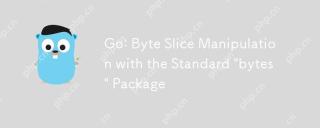
The"bytes"packageinGooffersefficientfunctionsformanipulatingbyteslices.1)Usebytes.Joinforconcatenatingslices,2)bytes.Bufferforincrementalwriting,3)bytes.Indexorbytes.IndexByteforsearching,4)bytes.Readerforreadinginchunks,and5)bytes.SplitNor
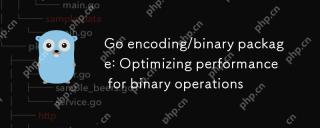
Theencoding/binarypackageinGoiseffectiveforoptimizingbinaryoperationsduetoitssupportforendiannessandefficientdatahandling.Toenhanceperformance:1)Usebinary.NativeEndianfornativeendiannesstoavoidbyteswapping.2)BatchReadandWriteoperationstoreduceI/Oover
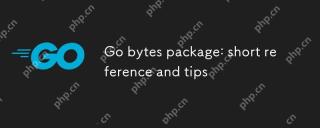
Go's bytes package is mainly used to efficiently process byte slices. 1) Using bytes.Buffer can efficiently perform string splicing to avoid unnecessary memory allocation. 2) The bytes.Equal function is used to quickly compare byte slices. 3) The bytes.Index, bytes.Split and bytes.ReplaceAll functions can be used to search and manipulate byte slices, but performance issues need to be paid attention to.
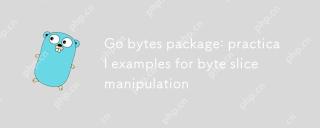
The byte package provides a variety of functions to efficiently process byte slices. 1) Use bytes.Contains to check the byte sequence. 2) Use bytes.Split to split byte slices. 3) Replace the byte sequence bytes.Replace. 4) Use bytes.Join to connect multiple byte slices. 5) Use bytes.Buffer to build data. 6) Combined bytes.Map for error processing and data verification.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
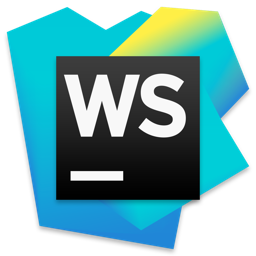
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
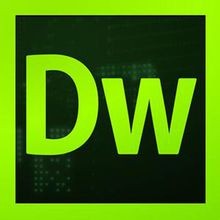
Dreamweaver CS6
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 Chinese version
Chinese version, very easy to use
