


Explain the different sorting algorithms (e.g., bubble sort, insertion sort, merge sort, quicksort, heapsort). What are their time complexities?
Bubble Sort:
Bubble sort is a simple sorting algorithm that repeatedly steps through the list, compares adjacent elements, and swaps them if they are in the wrong order. The pass through the list is repeated until the list is sorted. The time complexity of bubble sort is O(n^2) in the average and worst cases, where n is the number of items being sorted. In the best case, where the list is already sorted, the time complexity is O(n).
Insertion Sort:
Insertion sort builds the final sorted array one item at a time. It is much less efficient on large lists than more advanced algorithms such as quicksort, heapsort, or merge sort. However, it performs well for small lists or nearly sorted lists. The time complexity of insertion sort is O(n^2) in the average and worst cases, and O(n) in the best case.
Merge Sort:
Merge sort is a divide-and-conquer algorithm that divides the unsorted list into n sublists, each containing one element (a list of one element is considered sorted), and repeatedly merges sublists to produce new sorted sublists until there is only one sublist remaining. The time complexity of merge sort is O(n log n) in all cases (best, average, and worst).
Quicksort:
Quicksort is also a divide-and-conquer algorithm that works by selecting a 'pivot' element from the array and partitioning the other elements into two sub-arrays, according to whether they are less than or greater than the pivot. The sub-arrays are then sorted recursively. The time complexity of quicksort is O(n log n) on average and in the best case, but it can degrade to O(n^2) in the worst case.
Heapsort:
Heapsort involves building a max-heap from the list, then repeatedly extracting the maximum element from the heap and placing it at the end of the sorted array. The time complexity of heapsort is O(n log n) in all cases (best, average, and worst).
Which sorting algorithm is most efficient for small datasets and why?
For small datasets, insertion sort is often the most efficient sorting algorithm. This is because insertion sort has a best-case time complexity of O(n), which occurs when the input is already sorted or nearly sorted. For small datasets, the overhead of more complex algorithms like quicksort or merge sort may outweigh their benefits, making insertion sort a good choice due to its simplicity and efficiency in these scenarios.
How does the choice of pivot affect the performance of quicksort?
The choice of pivot in quicksort significantly affects its performance. The pivot is used to partition the array into two sub-arrays, and the efficiency of this partitioning directly impacts the overall performance of the algorithm.
- Best Case: If the pivot chosen always divides the array into two equal halves, quicksort achieves its best-case time complexity of O(n log n). This happens when the pivot is the median of the array.
- Average Case: In practice, choosing a random pivot or the middle element often results in an average-case time complexity of O(n log n), as it tends to divide the array into roughly equal parts over multiple iterations.
- Worst Case: The worst-case scenario occurs when the pivot chosen is always the smallest or largest element in the array, leading to unbalanced partitions. This results in a time complexity of O(n^2). This can happen, for example, if the array is already sorted and the first or last element is chosen as the pivot.
Therefore, strategies like choosing a random pivot or using the median-of-three method (selecting the median of the first, middle, and last elements) can help mitigate the risk of encountering the worst-case scenario.
Can you recommend a sorting algorithm for large datasets and explain its advantages?
For large datasets, I recommend using mergesort. Mergesort has several advantages that make it suitable for sorting large datasets:
- Stable and Consistent Performance: Mergesort has a time complexity of O(n log n) in all cases (best, average, and worst), making its performance predictable and reliable regardless of the input data's initial order.
- Efficient Use of Memory: While mergesort does require additional memory for the merging process, it can be implemented in a way that minimizes memory usage, such as using an in-place merge or external sorting for extremely large datasets that do not fit in memory.
- Parallelization: Mergesort is well-suited for parallel processing, as the divide-and-conquer approach allows different parts of the array to be sorted independently before being merged. This can significantly speed up the sorting process on multi-core systems or distributed computing environments.
- Stability: Mergesort is a stable sorting algorithm, meaning that it preserves the relative order of equal elements. This can be important in applications where the order of equal elements matters.
Overall, the consistent O(n log n) time complexity, potential for parallelization, and stability make mergesort an excellent choice for sorting large datasets.
The above is the detailed content of Explain the different sorting algorithms (e.g., bubble sort, insertion sort, merge sort, quicksort, heapsort). What are their time complexities?. For more information, please follow other related articles on the PHP Chinese website!
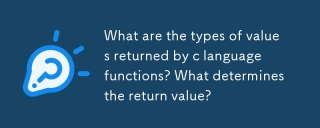
This article details C function return types, encompassing basic (int, float, char, etc.), derived (arrays, pointers, structs), and void types. The compiler determines the return type via the function declaration and the return statement, enforcing

Gulc is a high-performance C library prioritizing minimal overhead, aggressive inlining, and compiler optimization. Ideal for performance-critical applications like high-frequency trading and embedded systems, its design emphasizes simplicity, modul
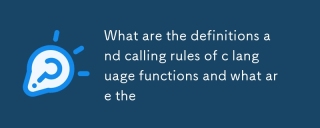
This article explains C function declaration vs. definition, argument passing (by value and by pointer), return values, and common pitfalls like memory leaks and type mismatches. It emphasizes the importance of declarations for modularity and provi

This article details C functions for string case conversion. It explains using toupper() and tolower() from ctype.h, iterating through strings, and handling null terminators. Common pitfalls like forgetting ctype.h and modifying string literals are
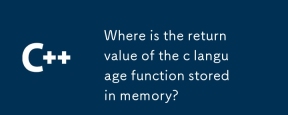
This article examines C function return value storage. Small return values are typically stored in registers for speed; larger values may use pointers to memory (stack or heap), impacting lifetime and requiring manual memory management. Directly acc
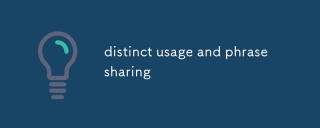
This article analyzes the multifaceted uses of the adjective "distinct," exploring its grammatical functions, common phrases (e.g., "distinct from," "distinctly different"), and nuanced application in formal vs. informal
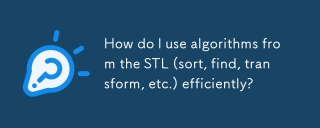
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
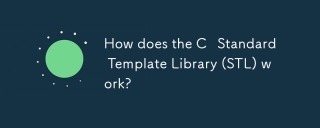
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
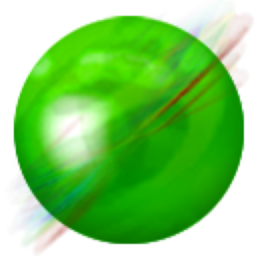
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
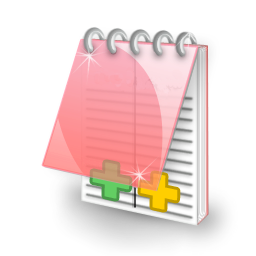
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor
