Recently a friend asked me how to dynamically change one HTML element to another in a Vue template block. For example, according to certain conditions<div> Switch element to<code><p> element. The key is how to do this without relying on a series of <code>v-if
and v-else
codes. At first I didn't care much because I couldn't see a strong reason for doing so; this was not common. However, later the same day, he contacted me again and told me that he had learned how to change the element type. He excitedly pointed out that Vue has a built-in component that can be used as a dynamic element, just what he needs.
This small feature keeps the template code simple and neat. It reduces the redundant code of v-if
and v-else
, making the code easier to understand and maintain. This allows us to create well-written and more complex conditions in a script block using methods or computed properties. These things should be placed in scripts, not in template blocks.
The main reason I wrote this article is that this function is used in many places in the design system we work for. Admittedly, this is not a big feature and is hardly mentioned in the documentation, at least as far as I can tell. However, it has the potential to help render specific HTML elements in components.
Vue's built-in<component></component>
element
There are several features in Vue that can easily change views dynamically. One of the features is built-in<component></component>
element, which allows components to switch dynamically. There is a short description of using this element with an HTML element in the Vue 2 and Vue 3 documentation; this is the part we are going to explore now.
The concept is to use<component></component>
This characteristic of an element replaces commonly used HTML elements that are similar in nature but have different functions, semantics or visual effects. The following basic examples will demonstrate the potential of this element in keeping Vue components simple and neat.
Button or link?
Buttons and links are often used interchangeably, but their functions, semantics and even visuals vary greatly. Generally speaking, the button (<button></button>
) is used for internal operations in the current view and is bound to JavaScript code. On the other hand, link (<a></a>
) is intended to point to another resource, whether it is a resource on the host server or an external resource; in most cases it is a web page. Single-page applications tend to rely more on buttons than links, but both are needed.
Links are often visually designed to look like buttons, just like the buttons created by Bootstrap's .btn
class. With this in mind, we can easily create a component that switches between these two elements according to a single prop. By default, the component will be a button, but if https://www.php.cn/link/ed2ddd0dcd323046d0f9a51e5cc51c60
prop is applied, it will render as a link.
This is in the template<component></component>
:
<component :https: :is="element"><slot></slot></component>
This binding property points to a computed property named element
, while the binding https://www.php.cn/link/ed2ddd0dcd323046d0f9a51e5cc51c60
property uses the appropriately named https://www.php.cn/link/ed2ddd0dcd323046d0f9a51e5cc51c60
prop. This takes advantage of Vue's normal behavior, that is, if prop has no value, the bound properties will not appear in the rendered HTML element. Whether the final element is a button or a link, slot provides internal content.
Calculating properties is simple:
element() { return this.https://www.php.cn/link/ed2ddd0dcd323046d0f9a51e5cc51c60 ? 'a' : 'button'; }
If there is https://www.php.cn/link/ed2ddd0dcd323046d0f9a51e5cc51c60
prop, then the application<a></a>
element; otherwise we get one<button></button>
.
<my-button> This is a button</my-button> <my-button https:> This is a link</my-button>
HTML is presented as follows:
<button>This is a button</button> <a https:>This is a link</a>
In this case one might expect the two elements to be visually similar, but they are obviously different for semantic and accessible needs. That is, the elements of the two outputs do not have to have the same style. You can use an element with the selector div.my-button
in a style block, or create a dynamic class that changes according to the element.
The overall goal is to simplify things by allowing a component to render as two different HTML elements as needed - no v-if
or v-else
!
Ordered or unordered list?
Similar to the button example above, we can create a component that outputs different list elements. Since both unordered and ordered lists use the same list item (<li>
) element as child elements, so this is easy to implement; we just need to exchange<ul></ul>
and<ol></ol>
. Even if we want to choose to use the description list<dl></dl>
, this is also easy to implement, because the content is just an acceptable one<dt></dt>
/<dd></dd>
Combined slot. The template code is very similar to the button example:
<component :is="element"><slot> No list items!</slot></component>
Please note the default content inside the slot element, which I will talk about later.
There is a prop that specifies the list type to use, defaulting to ul
:
props: { listType: { type: String, default: 'ul' } }
Similarly, there is a computed property called element
:
element() { if (this.$slots.default) { return this.listType; } else { return 'div'; } }
In this case, we are testing whether the default slot exists, which means it has content to render. If present, use the list type passed through listType
prop. Otherwise, the element will become<div> , this will display the "No list items!" message inside the slot element. This way, if there is no list item, the HTML will not render as a list with only one item declared without items. It's up to you, though, but it's nice to consider the semantics of a list without obviously valid items. Another thing to consider is that the auxiliary tools can be confused as to think that this is a list with only one project declaration without projects. Just like the button example above, you can also style each list in different styles. This can locate elements with the class name <code>ul.my-list
based on the selector. Another option is to dynamically change the class name based on the selected element.
This example follows a BEM-like class naming structure:
<component :class="`my-list ${element}`" :is="element"><slot> No list items!</slot></component>
The usage is as simple as the previous button example:
<my-list><li> list item 1</li></my-list> <my-list list-type="ol"><li>list item 1</li></my-list> <my-list list-type="dl"><dt>Item 1</dt> <dd> This is item one.</dd></my-list> <my-list></my-list>
Each instance renders the specified list element. However, the last instance will generate a Even if these two examples are essentially changing the root element component, consider going deeper into the component. For example, the title may need to be based on certain conditions from If you find yourself having to use ternary operators to control what's more than a few properties, I recommend sticking with When creating components, if there is a simple Consider everything we’ve covered so far and apply it to a flexible card component. This card component example allows three different types of cards to be placed in a specific part of the article layout: Think of these as following the design system, and components control HTML for semantics and styling. In the example above, you can see the hero card at the top, followed by a series of call to action cards, and then—scroll down a little—you will see the message card on the right. Here is the template code for the card component: There are three "component" elements in the card. Each element represents a specific element within the card, but will change according to the card type. Each component calls There may be many ways to deal with this, but you have to move in a direction that suits your component needs. In this case, there is an object that tracks the HTML element tags for each part of each card type. The method then returns the required HTML element based on the current card type and the parameters passed in. For styles, I inserted a class on the root element of the card that is based on the type of the card. This makes it very easy to create each type of CSS as required. You can also create CSS based on the HTML element itself, but I prefer classes. Future changes to card components may change the HTML structure and are unlikely to change the logic of creating the class. The card also supports background images on the title of the hero card. This is done by placing a simple computed attribute If the image URL is provided in Here is the hero card in this demo: You will see that each section of the card has its own content slot. And, for simplicity, text is the only thing expected in the slot. The card component processes the required HTML elements according to the card type. Making components expect only text makes using components quite simple. It replaces the need to make decisions about HTML structure, thus simplifying the implementation of cards. For comparison, here are two other types used in the demo: Again, note that each slot only expects text, as each card type generates its own HTML element according to the definition of Weirdly named in Vue component Here is a new technology example shared by a friend, which has become an amazingly useful tool in the Vue feature I have used. I hope this post will pass on ideas and information about this little feature to you and let you explore how to take advantage of it in your own Vue project.<ol></ol>
To show the sort order, and then switch back when finished? Now we are controlling the elements
<h1></h1>
Change to<h3></h3>
.
v-if
. Writing more code to handle properties, classes, and properties only makes the code more complex than v-if
. In these cases, v-if
will make the code simpler in the long run, while simpler code is easier to read and maintain.v-if
to switch between elements, consider this small aspect of Vue's main functionality. Extended ideas, flexible card system
<li> Hero Card: This is expected to be used at the top of the page and is more eye-catching than other cards.
<li> Call to Action Card: Used as a series of user actions before or within the article.
<li> Information card: used for excerpts.
<component :class="`card ${type}`" :is="elements('root')">
<component :style="bg()" :is="elements('header')">
<slot name="header"></slot>
</component>
<div>
<slot name="content"></slot>
</div>
<component :is="elements('footer')">
<slot name="footer"></slot>
</component>
</component>
elements()
method with a parameter that identifies which part of the call card.elements()
method is: elements(which) {
const tags = {
hero: { root: 'section', header: 'h1', footer: 'date' },
cta: { root: 'section', header: 'h2', footer: 'div' },
info: { root: 'aside', header: 'h3', footer: 'small' }
}
return tags[this.type][which];
}
bg
on the title element. Here is the computed attribute: bg() {
return this.background ? `background-image: url(${this.background})` : null;
}
background
prop, the computed property returns a string for inline styles that apply the image as the background image. A fairly simple solution that can easily make it more powerful. For example, it can support custom colors, gradients, or default colors (if no image is provided). This example does not involve a lot of possibilities, as each card type may have its own optional prop for developers to take advantage of.<custom-card background="https://picsum.photos/id/237/800/200" type="hero">
<template v-slot:header>Article Title</template>
<template v-slot:content>Lorem ipsum...</template>
<template v-slot:footer>January 1, 2011</template>
</custom-card>
<custom-card type="cta">
<template v-slot:header>CTA Title One</template>
<template v-slot:content>Lorem ipsum dolor sit amet, consistetur apiscing elit.</template>
<template v-slot:footer>footer</template>
</custom-card>
<custom-card type="info">
<template v-slot:header>Here's a Quote</template>
<template v-slot:content>“Maecenas ... quis.”</template>
<template v-slot:footer>who said it</template>
</custom-card>
elements()
method. If you think in the future that different HTML elements should be used, you just need to update the component. Building accessibility features is another potential future update. Interaction functionality can even be expanded based on card type. The power of components in components
<component></component>
Elements were originally designed for one thing, but as often happens, it has a small side effect that makes it very useful in other ways.<component></component>
Elements are designed to dynamically switch Vue components within another component as needed. A basic idea about this might be a tab system for switching between components that act as pages; this is actually explained in the Vue documentation. But it supports doing the same for HTML elements.
The above is the detailed content of Dynamically Switching From One HTML Element to Another in Vue. For more information, please follow other related articles on the PHP Chinese website!
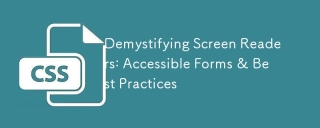
This is the 3rd post in a small series we did on form accessibility. If you missed the second post, check out "Managing User Focus with :focus-visible". In
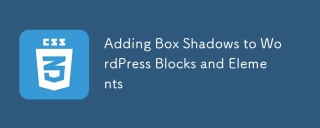
The CSS box-shadow and outline properties gained theme.json support in WordPress 6.1. Let's look at a few examples of how it works in real themes, and what options we have to apply these styles to WordPress blocks and elements.
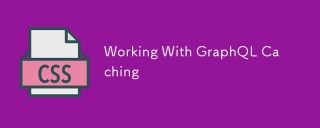
If you’ve recently started working with GraphQL, or reviewed its pros and cons, you’ve no doubt heard things like “GraphQL doesn’t support caching” or
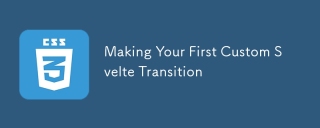
The Svelte transition API provides a way to animate components when they enter or leave the document, including custom Svelte transitions.
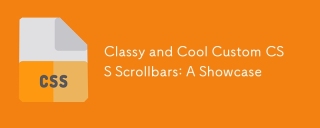
In this article we will be diving into the world of scrollbars. I know, it doesn’t sound too glamorous, but trust me, a well-designed page goes hand-in-hand
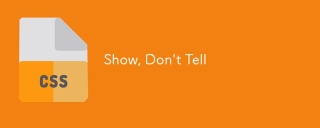
How much time do you spend designing the content presentation for your websites? When you write a new blog post or create a new page, are you thinking about
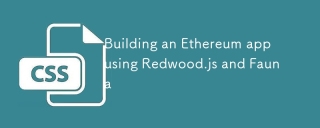
With the recent climb of Bitcoin’s price over 20k $USD, and to it recently breaking 30k, I thought it’s worth taking a deep dive back into creating Ethereum
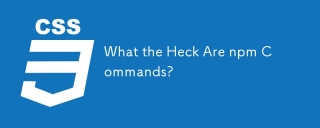
npm commands run various tasks for you, either as a one-off or a continuously running process for things like starting a server or compiling code.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Notepad++7.3.1
Easy-to-use and free code editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),