


How do you implement logging and monitoring in a distributed system built with Go?
Implementing logging and monitoring in a distributed system built with Go involves several steps that ensure the system's health and performance can be effectively tracked and managed. Here is a detailed approach to implementing these practices:
-
Logging:
- Centralized Logging: In a distributed system, it's crucial to centralize logs from all nodes to a single place for easier analysis and troubleshooting. Go offers several libraries that support centralized logging such as Logrus or Zap. These libraries can output logs to formats compatible with centralized logging systems like ELK Stack (Elasticsearch, Logstash, Kibana) or Splunk.
-
Structured Logging: Implement structured logging where logs are formatted as JSON. This allows for easier parsing and analysis. Go's standard
log
package can be extended with libraries like Logrus to support structured logging. - Logging Levels: Use different logging levels (debug, info, warning, error, fatal) to filter logs based on importance. Libraries like Logrus provide straightforward methods to implement this.
-
Monitoring:
- Metrics Collection: Use libraries like Prometheus to collect metrics from your Go services. Prometheus can scrape HTTP endpoints to gather data on system health, performance, and other custom metrics.
- Health Checks: Implement health check endpoints in your Go applications to allow monitoring systems to check the status of each service. These can be simple HTTP endpoints that return the health status of the service.
- Tracing: Utilize distributed tracing tools like Jaeger or Zipkin to monitor requests as they flow through the system. Libraries like OpenTracing or OpenTelemetry can be integrated with Go applications to implement tracing.
-
Alerting:
- Set up alerting based on the collected logs and metrics. Tools like Prometheus can be configured with Alertmanager to send alerts to various platforms like email, Slack, or PagerDuty when certain thresholds are breached.
By following these practices, a distributed system built with Go can be effectively monitored and logged, ensuring that it remains reliable and performant.
What are the best practices for setting up logging in a Go-based distributed system?
Setting up logging in a Go-based distributed system effectively involves adhering to several best practices:
-
Use Structured Logging:
- Employ JSON or another structured format for logs. This facilitates easier parsing and analysis. Libraries like Logrus or Zap can help achieve this in Go.
-
Implement Log Levels:
- Use different log levels such as debug, info, warning, error, and fatal to categorize logs by severity. This helps in filtering logs based on the current need, whether it's debugging or monitoring production issues.
-
Centralize Logs:
- Centralize logs from all nodes of the distributed system to a single platform. This can be achieved using tools like ELK Stack or Splunk. Ensure that your Go applications can output logs in a format compatible with these systems.
-
Include Contextual Information:
- Logs should include contextual information such as timestamps, service names, and request IDs. This helps in correlating logs across different services and understanding the flow of requests.
-
Asynchronous Logging:
- Implement asynchronous logging to prevent logging from becoming a bottleneck in your application. Libraries like Zap support asynchronous logging out of the box.
-
Log Rotation and Retention:
- Set up log rotation to manage log file sizes and retention policies to ensure that logs do not consume too much storage over time. Tools like logrotate can be used for this purpose.
-
Security and Compliance:
- Ensure that logs do not contain sensitive information. Implement proper access controls and encryption for logs, especially when they are transmitted over the network or stored.
By following these best practices, you can set up a robust logging system in your Go-based distributed environment that aids in troubleshooting and maintaining system health.
How can you effectively monitor the performance of a distributed system using Go?
Monitoring the performance of a distributed system using Go involves several key strategies and tools:
-
Metrics Collection:
- Use a metrics collection library like Prometheus to gather performance data from your Go services. Prometheus can scrape HTTP endpoints to collect metrics such as CPU usage, memory consumption, request latency, and custom business metrics.
-
Health Checks:
- Implement health check endpoints in your Go applications. These endpoints can be used by monitoring systems to check the status of each service. A simple HTTP endpoint that returns the health status of the service can be very effective.
-
Distributed Tracing:
- Utilize distributed tracing tools like Jaeger or Zipkin to monitor requests as they flow through the system. Libraries like OpenTracing or OpenTelemetry can be integrated with Go applications to implement tracing. This helps in understanding the performance bottlenecks and dependencies between services.
-
Real-time Monitoring:
- Use real-time monitoring tools like Grafana to visualize the metrics collected by Prometheus. Grafana can create dashboards that provide insights into the system's performance in real-time.
-
Alerting:
- Set up alerting based on the collected metrics. Tools like Prometheus can be configured with Alertmanager to send alerts to various platforms like email, Slack, or PagerDuty when certain thresholds are breached. This ensures that you are notified of performance issues promptly.
-
Custom Metrics:
- Implement custom metrics that are specific to your application's business logic. This could include metrics like the number of active users, transaction rates, or any other performance indicators relevant to your system.
By implementing these strategies, you can effectively monitor the performance of your distributed system built with Go, ensuring that it remains efficient and reliable.
What tools should be used to integrate logging and monitoring in a Go distributed environment?
To effectively integrate logging and monitoring in a Go distributed environment, several tools can be utilized:
-
Logging Tools:
- Logrus: A popular logging library for Go that supports structured logging and different log levels. It can be configured to output logs in JSON format, which is suitable for centralized logging systems.
- Zap: Another high-performance logging library for Go that supports structured, leveled logging. It is known for its speed and efficiency, making it suitable for high-throughput environments.
- ELK Stack (Elasticsearch, Logstash, Kibana): A powerful suite for centralized logging. Elasticsearch stores logs, Logstash processes them, and Kibana provides a user interface for searching and visualizing logs.
- Splunk: A comprehensive platform for searching, monitoring, and analyzing machine-generated data. It can be used to centralize and analyze logs from your Go services.
-
Monitoring Tools:
- Prometheus: An open-source monitoring and alerting toolkit that can scrape metrics from your Go services. It is widely used in distributed systems for its scalability and flexibility.
- Grafana: A tool for querying, visualizing, and alerting on metrics. It can be used in conjunction with Prometheus to create dashboards that provide real-time insights into system performance.
- Jaeger: An open-source, end-to-end distributed tracing system. It can be integrated with Go applications using libraries like OpenTracing or OpenTelemetry to monitor request flows.
- Zipkin: Another distributed tracing system that can be used to track requests across your distributed system. It is compatible with Go through libraries like OpenTracing.
-
Alerting Tools:
- Alertmanager: A component of the Prometheus ecosystem that handles alerts sent by client applications such as the Prometheus server. It can be configured to send notifications to various platforms like email, Slack, or PagerDuty.
By using these tools, you can create a comprehensive logging and monitoring solution for your Go-based distributed system, ensuring that you have the visibility and control needed to maintain its health and performance.
The above is the detailed content of How do you implement logging and monitoring in a distributed system built with Go?. For more information, please follow other related articles on the PHP Chinese website!
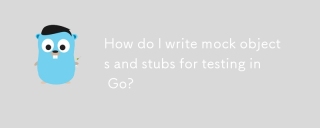
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
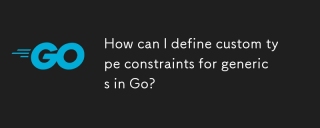
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
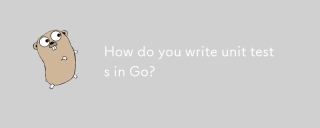
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
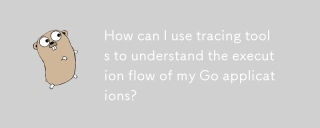
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
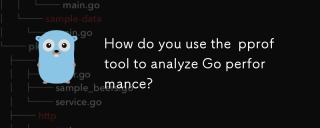
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
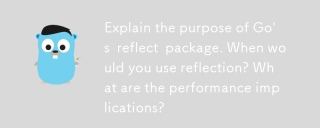
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
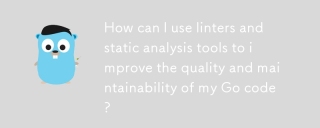
This article advocates for using linters and static analysis tools to enhance Go code quality. It details tool selection (e.g., golangci-lint, go vet), workflow integration (IDE, CI/CD), and effective interpretation of warnings/errors to improve cod
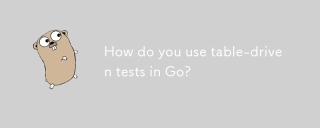
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
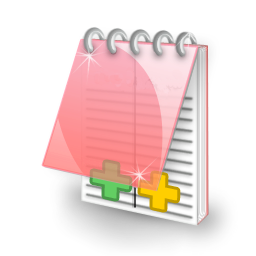
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 English version
Recommended: Win version, supports code prompts!
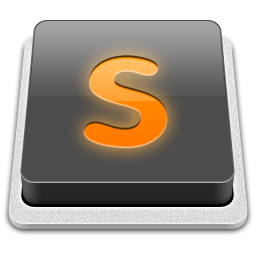
SublimeText3 Mac version
God-level code editing software (SublimeText3)
