The article discusses best practices for writing testable Go code, including separation of concerns, dependency injection, and using Go's built-in testing tools.
What are the best practices for writing testable Go code?
Writing testable Go code is crucial for maintaining and evolving software projects. Here are some best practices to follow:
- Separation of Concerns: Ensure that each function or method has a single responsibility. This makes it easier to test individual components without affecting others. For example, instead of having a function that both processes data and logs it, separate these into two functions.
- Dependency Injection: Use dependency injection to make your code more modular and easier to test. By injecting dependencies, you can easily mock them in your tests. For instance, instead of directly creating a database connection within a function, pass it as a parameter.
- Interface-based Programming: Define interfaces for your dependencies. This allows you to easily swap out implementations with mocks during testing. For example, if you have a function that uses a database, define an interface for the database operations and use that interface in your function.
- Avoid Global State: Global state can make your code harder to test because it can lead to unpredictable behavior. Instead, pass state as parameters to functions or methods.
- Write Small, Focused Functions: Smaller functions are easier to test because they have fewer paths of execution. Aim for functions that do one thing well.
- Use Pure Functions When Possible: Pure functions, which always produce the same output for the same input and have no side effects, are inherently easier to test.
- Test-Driven Development (TDD): Writing tests before writing the actual code can help ensure that your code is testable from the start. This approach also helps in designing better APIs.
-
Use Go's Built-in Testing Tools: Go comes with a built-in testing framework (
testing
package) and a command-line tool (go test
). Make use of these tools to write and run your tests.
By following these best practices, you can write Go code that is more testable, maintainable, and robust.
How can I structure my Go code to make it more testable?
Structuring your Go code effectively can significantly enhance its testability. Here are some strategies to consider:
- Package Organization: Organize your code into logical packages. Each package should have a clear purpose and contain related functionality. This makes it easier to test individual packages in isolation.
- Layered Architecture: Implement a layered architecture where different layers handle different responsibilities. For example, you might have a data access layer, a business logic layer, and a presentation layer. This separation makes it easier to test each layer independently.
-
Use Interfaces: Define interfaces for your dependencies and use them in your code. This allows you to easily swap out real implementations with mock objects during testing. For example:
type Database interface { GetUser(id int) (User, error) } func GetUserFromDB(db Database, id int) (User, error) { return db.GetUser(id) }
- Dependency Injection: Instead of hardcoding dependencies, inject them into your functions or structs. This makes it easier to replace them with test doubles during testing.
- Avoid Deep Nesting: Deeply nested code can be hard to test. Try to keep your code flat and use early returns to reduce nesting.
- Use Constructor Functions: Use constructor functions to create instances of your structs. This allows you to easily inject dependencies and makes your code more testable.
- Separate Concerns: Ensure that each function or method has a single responsibility. This makes it easier to test individual components without affecting others.
By structuring your Go code with these principles in mind, you can make it more modular, easier to understand, and more testable.
What tools can help improve the testability of Go code?
Several tools can help improve the testability of Go code. Here are some of the most useful ones:
-
Go Test: Go's built-in testing framework (
testing
package) and command-line tool (go test
) are essential for writing and running tests. They provide a simple and effective way to test your Go code. - GoMock: GoMock is a mocking framework for Go. It allows you to generate mock objects for your interfaces, making it easier to test code that depends on external services or databases.
- Testify: Testify is a popular testing toolkit for Go. It provides additional assertion functions and a suite of tools for writing more expressive and maintainable tests.
- Ginkgo: Ginkgo is a BDD (Behavior-Driven Development) testing framework for Go. It provides a more expressive way to write tests and can be particularly useful for writing integration tests.
- Gomega: Gomega is a matcher library that works well with Ginkgo. It provides a rich set of matchers for writing more readable and expressive assertions.
- GoCov: GoCov is a tool for collecting and reporting code coverage information. It can help you identify areas of your code that are not adequately tested.
- GoLeak: GoLeak is a tool for detecting goroutine leaks in your tests. It can help ensure that your tests are not leaving goroutines running, which can lead to false positives in test results.
- SonarQube: SonarQube is a code quality and security tool that can analyze your Go code for potential issues, including testability problems. It can help you identify areas where your code could be more testable.
By using these tools, you can enhance the testability of your Go code, making it easier to write, run, and maintain tests.
What common pitfalls should I avoid when writing testable Go code?
When writing testable Go code, it's important to be aware of common pitfalls that can make your code harder to test. Here are some to avoid:
- Tight Coupling: Avoid tightly coupling your code to specific implementations. Instead, use interfaces and dependency injection to make your code more modular and easier to test.
- Global State: Using global state can make your code harder to test because it can lead to unpredictable behavior. Instead, pass state as parameters to functions or methods.
- Complex Functions: Writing functions that do too much can make them hard to test. Aim for small, focused functions that do one thing well.
- Side Effects: Functions with side effects, such as modifying global state or making external calls, can be difficult to test. Try to use pure functions when possible.
- Hardcoded Dependencies: Hardcoding dependencies into your code can make it difficult to replace them with test doubles. Use dependency injection to make your code more testable.
- Ignoring Error Handling: Proper error handling is crucial for writing testable code. Make sure to handle errors appropriately and test error paths as well as happy paths.
- Overuse of Mocks: While mocks can be useful, overusing them can lead to brittle tests that break easily. Use mocks judiciously and consider using real implementations when possible.
- Neglecting Test Coverage: Failing to achieve adequate test coverage can leave parts of your code untested and vulnerable to bugs. Use tools like GoCov to monitor your test coverage and ensure that all critical paths are tested.
- Ignoring Integration Tests: While unit tests are important, neglecting integration tests can leave gaps in your testing strategy. Make sure to write integration tests to ensure that different components work together correctly.
By avoiding these common pitfalls, you can write Go code that is more testable, maintainable, and robust.
The above is the detailed content of What are the best practices for writing testable Go code?. For more information, please follow other related articles on the PHP Chinese website!
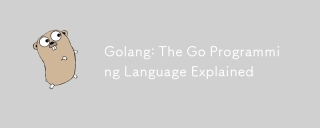
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
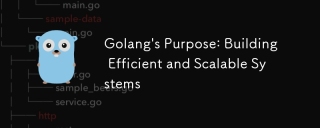
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
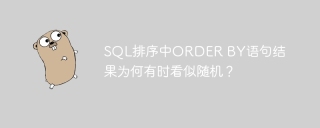
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
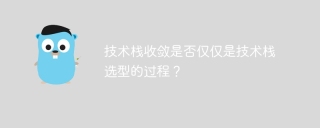
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...
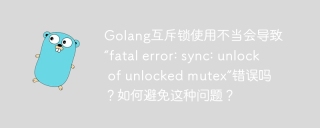
Golang ...
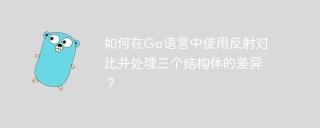
How to compare and handle three structures in Go language. In Go programming, it is sometimes necessary to compare the differences between two structures and apply these differences to the...
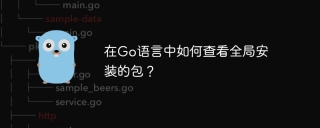
How to view globally installed packages in Go? In the process of developing with Go language, go often uses...
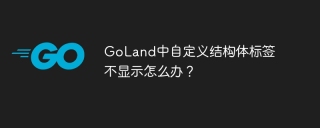
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
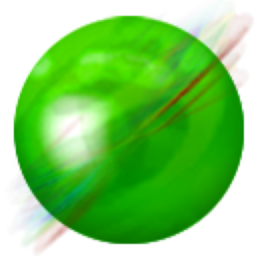
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 Linux new version
SublimeText3 Linux latest version

SublimeText3 Chinese version
Chinese version, very easy to use