How can you use WebSockets in UniApp to create real-time applications?
UniApp, a framework that allows developers to write code once and deploy it across multiple platforms, supports the use of WebSockets for creating real-time applications. WebSockets provide a full-duplex communication channel over a single TCP connection, which is ideal for applications requiring real-time data exchange, such as chat apps, live updates, and gaming.
To use WebSockets in UniApp, you can follow these steps:
-
Initialize WebSocket Connection: Use the
uni.connectSocket
API to establish a WebSocket connection. You need to specify the URL of the WebSocket server. For example:uni.connectSocket({ url: 'wss://your-websocket-server.com/socket', success: function() { console.log('WebSocket connection established.'); } });
-
Send Data: Once the connection is established, you can send data to the server using
uni.sendSocketMessage
. For instance:uni.sendSocketMessage({ data: 'Hello, WebSocket Server!', success: function() { console.log('Message sent successfully.'); } });
-
Receive Data: To handle incoming messages, use the
onSocketMessage
event listener:uni.onSocketMessage(function(res) { console.log('Received message:', res.data); });
-
Close Connection: When you need to close the connection, use
uni.closeSocket
:uni.closeSocket({ success: function() { console.log('WebSocket connection closed.'); } });
By integrating these steps into your UniApp project, you can create applications that provide real-time functionality, enhancing user experience with instant updates and interactions.
What are the best practices for implementing WebSockets in UniApp for optimal performance?
Implementing WebSockets in UniApp with optimal performance involves several best practices:
- Use Efficient Data Formats: Opt for lightweight data formats like JSON or Protocol Buffers to minimize the payload size and reduce bandwidth usage.
-
Implement Heartbeats: Use periodic heartbeat messages to keep the connection alive and detect disconnections quickly. This can be done by sending a simple ping message at regular intervals:
setInterval(() => { uni.sendSocketMessage({ data: JSON.stringify({ type: 'ping' }), }); }, 30000); // Every 30 seconds
- Optimize Message Handling: Process incoming messages efficiently. Use event-driven programming to handle different types of messages and avoid blocking the main thread.
-
Connection Management: Implement a reconnection strategy to handle temporary network issues. For example, attempt to reconnect with exponential backoff:
let reconnectAttempts = 0; function reconnect() { setTimeout(() => { uni.connectSocket({ url: 'wss://your-websocket-server.com/socket', success: () => { reconnectAttempts = 0; }, fail: () => { reconnectAttempts ; reconnect(); } }); }, Math.min(1000 * Math.pow(2, reconnectAttempts), 30000)); }
- Error Handling: Implement robust error handling to manage connection failures and unexpected errors gracefully.
- Resource Management: Ensure that WebSocket connections are closed properly when they are no longer needed to free up resources.
By following these practices, you can enhance the performance and reliability of your WebSocket-based applications in UniApp.
How can you handle WebSocket connections and disconnections effectively in UniApp?
Handling WebSocket connections and disconnections effectively in UniApp involves several key strategies:
-
Connection Establishment: Use
uni.connectSocket
to initiate a connection and handle thesuccess
andfail
callbacks to manage the initial connection state:uni.connectSocket({ url: 'wss://your-websocket-server.com/socket', success: function() { console.log('WebSocket connection established.'); }, fail: function() { console.log('Failed to establish WebSocket connection.'); } });
-
Connection Monitoring: Use
onSocketOpen
andonSocketClose
to monitor the connection status:uni.onSocketOpen(function() { console.log('WebSocket connection opened.'); }); uni.onSocketClose(function() { console.log('WebSocket connection closed.'); });
-
Reconnection Strategy: Implement a reconnection mechanism to handle temporary disconnections. Use exponential backoff to avoid overwhelming the server with reconnection attempts:
let reconnectAttempts = 0; function reconnect() { setTimeout(() => { uni.connectSocket({ url: 'wss://your-websocket-server.com/socket', success: () => { reconnectAttempts = 0; }, fail: () => { reconnectAttempts ; reconnect(); } }); }, Math.min(1000 * Math.pow(2, reconnectAttempts), 30000)); }
-
Error Handling: Use
onSocketError
to handle any errors that occur during the WebSocket communication:uni.onSocketError(function(res) { console.log('WebSocket error:', res); });
-
Graceful Disconnection: Ensure that the WebSocket connection is closed properly when the application is closed or when the user logs out:
uni.closeSocket({ success: function() { console.log('WebSocket connection closed gracefully.'); } });
By implementing these strategies, you can ensure that your UniApp application handles WebSocket connections and disconnections smoothly, providing a robust user experience.
What security measures should be considered when using WebSockets in UniApp for real-time applications?
When using WebSockets in UniApp for real-time applications, several security measures should be considered to protect your application and its users:
- Use Secure WebSocket (WSS): Always use the secure WebSocket protocol (wss://) instead of the non-secure version (ws://) to encrypt data in transit. This prevents man-in-the-middle attacks and ensures data integrity.
-
Authentication and Authorization: Implement strong authentication mechanisms to verify the identity of users before allowing them to establish a WebSocket connection. Use tokens or session IDs to authenticate WebSocket connections:
uni.connectSocket({ url: 'wss://your-websocket-server.com/socket', header: { 'Authorization': 'Bearer ' userToken } });
- Data Validation: Validate and sanitize all incoming and outgoing data to prevent injection attacks. Ensure that the data conforms to expected formats and does not contain malicious content.
- Rate Limiting: Implement rate limiting on the server-side to prevent abuse and denial-of-service (DoS) attacks. This can help manage the load and protect against malicious users attempting to flood the server with messages.
- Connection Limits: Set limits on the number of concurrent WebSocket connections per user to prevent resource exhaustion and potential abuse.
- Encryption: Use end-to-end encryption for sensitive data to ensure that even if the data is intercepted, it remains unreadable to unauthorized parties.
- Logging and Monitoring: Implement comprehensive logging and monitoring to detect and respond to security incidents promptly. Monitor for unusual patterns of activity that may indicate a security breach.
- Secure WebSocket Server: Ensure that the WebSocket server itself is secure. Keep the server software up to date, use firewalls, and follow best practices for server security.
By incorporating these security measures, you can enhance the safety and reliability of your UniApp real-time applications that use WebSockets.
The above is the detailed content of How can you use WebSockets in UniApp to create real-time applications?. For more information, please follow other related articles on the PHP Chinese website!
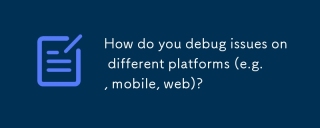
The article discusses debugging strategies for mobile and web platforms, highlighting tools like Android Studio, Xcode, and Chrome DevTools, and techniques for consistent results across OS and performance optimization.

The article discusses debugging tools and best practices for UniApp development, focusing on tools like HBuilderX, WeChat Developer Tools, and Chrome DevTools.

The article discusses end-to-end testing for UniApp applications across multiple platforms. It covers defining test scenarios, choosing tools like Appium and Cypress, setting up environments, writing and running tests, analyzing results, and integrat
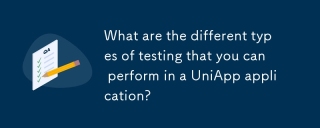
The article discusses various testing types for UniApp applications, including unit, integration, functional, UI/UX, performance, cross-platform, and security testing. It also covers ensuring cross-platform compatibility and recommends tools like Jes
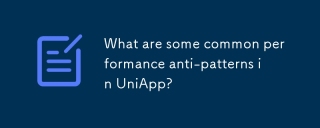
The article discusses common performance anti-patterns in UniApp development, such as excessive global data use and inefficient data binding, and offers strategies to identify and mitigate these issues for better app performance.
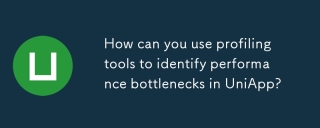
The article discusses using profiling tools to identify and resolve performance bottlenecks in UniApp, focusing on setup, data analysis, and optimization.
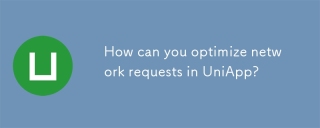
The article discusses strategies for optimizing network requests in UniApp, focusing on reducing latency, implementing caching, and using monitoring tools to enhance application performance.
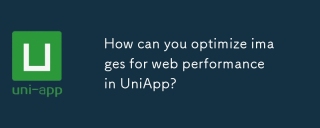
The article discusses optimizing images in UniApp for better web performance through compression, responsive design, lazy loading, caching, and using WebP format.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Notepad++7.3.1
Easy-to-use and free code editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool