


How do you handle data persistence in UniApp (e.g., using local storage, databases)?
How do you handle data persistence in UniApp (e.g., using local storage, databases)?
In UniApp, data persistence can be managed through various methods, primarily using local storage and databases. Here's a detailed look at how you can handle data persistence in UniApp:
-
Local Storage:
UniApp provides a straightforward way to use local storage through theuni.setStorage
,uni.getStorage
, anduni.removeStorage
APIs. These APIs allow you to store data in key-value pairs, which is suitable for small amounts of data such as user preferences or session data.-
Example of setting data:
uni.setStorage({ key: 'userInfo', data: { name: 'John Doe', age: 30 }, success: function () { console.log('Data stored successfully'); } });
-
Example of retrieving data:
uni.getStorage({ key: 'userInfo', success: function (res) { console.log('Data retrieved:', res.data); } });
-
-
Databases:
For more complex data management, UniApp supports integration with databases. You can use SQLite for local storage or connect to remote databases like MySQL or MongoDB through APIs or third-party plugins.-
SQLite Example:
UniApp supports SQLite through plugins likeuni-sqlite
. You can install it via npm and use it to create and manage databases locally.const sqlite = require('@dcloudio/uni-sqlite'); const db = new sqlite.Database('myDatabase.db'); db.run('CREATE TABLE IF NOT EXISTS users (id INTEGER PRIMARY KEY, name TEXT, age INTEGER)'); db.run('INSERT INTO users (name, age) VALUES (?, ?)', ['John Doe', 30]); db.all('SELECT * FROM users', [], (err, rows) => { if (err) { console.error(err); } else { console.log('Users:', rows); } });
-
Remote Database Example:
You can use UniApp'suni.request
API to interact with remote databases. For instance, you can send a POST request to a server to store data in a MySQL database.uni.request({ url: 'https://your-server.com/api/users', method: 'POST', data: { name: 'John Doe', age: 30 }, success: function (res) { console.log('Data sent to server:', res.data); } });
-
By using these methods, you can effectively manage data persistence in UniApp, choosing the appropriate method based on your application's needs.
What are the best practices for managing local storage in UniApp to ensure data security?
To ensure data security when managing local storage in UniApp, follow these best practices:
-
Encryption:
Always encrypt sensitive data before storing it locally. UniApp does not provide built-in encryption, but you can use third-party libraries likecrypto-js
to encrypt data before storing it withuni.setStorage
.const CryptoJS = require('crypto-js'); const encryptedData = CryptoJS.AES.encrypt(JSON.stringify({ name: 'John Doe', age: 30 }), 'secret key').toString(); uni.setStorage({ key: 'userInfo', data: encryptedData, success: function () { console.log('Encrypted data stored successfully'); } });
-
Data Minimization:
Store only the necessary data in local storage. Avoid storing sensitive information like passwords or credit card numbers. If such data must be stored, ensure it is encrypted and stored for the shortest possible time. -
Secure Storage:
Use secure storage mechanisms provided by the device's operating system when possible. For example, on iOS, you can use the Keychain, and on Android, you can use the Android Keystore system. -
Regular Data Cleanup:
Implement mechanisms to regularly clean up or update data stored in local storage. This helps in reducing the risk of data breaches and ensures that outdated or unnecessary data is removed. -
Access Control:
Implement strict access controls to ensure that only authorized parts of your application can access the stored data. Use UniApp's built-in security features and consider implementing additional checks. -
Data Integrity:
Use checksums or digital signatures to ensure the integrity of the data stored in local storage. This helps in detecting any unauthorized modifications to the data.
By following these best practices, you can enhance the security of data stored in local storage within UniApp.
Can you recommend a suitable database solution for UniApp that supports offline data syncing?
For UniApp applications that require offline data syncing, a suitable database solution would be PouchDB. PouchDB is a JavaScript database that can be used both in the browser and on the server, making it ideal for UniApp's cross-platform nature. It supports seamless synchronization with CouchDB, which can be used as a backend database.
Here's why PouchDB is recommended:
-
Offline First:
PouchDB is designed to work offline first, allowing your UniApp to function without an internet connection. Once the connection is restored, it automatically syncs data with the remote CouchDB server. -
Easy Integration:
PouchDB can be easily integrated into UniApp using npm. You can install it with:npm install pouchdb-browser
-
Synchronization:
PouchDB provides robust synchronization capabilities. You can set up a local PouchDB instance in your UniApp and sync it with a remote CouchDB server.const PouchDB = require('pouchdb-browser'); const localDB = new PouchDB('myLocalDB'); const remoteDB = new PouchDB('http://your-couchdb-server.com/your-database'); localDB.sync(remoteDB, { live: true, retry: true }).on('change', function (change) { console.log('Data synced:', change); }).on('error', function (err) { console.error('Sync error:', err); });
-
Cross-Platform Compatibility:
PouchDB works across different platforms supported by UniApp, including iOS, Android, and web browsers. -
Flexible Data Model:
PouchDB uses a flexible JSON-based data model, which is suitable for various types of applications and data structures.
By using PouchDB, you can ensure that your UniApp can handle offline data syncing efficiently and securely.
How does UniApp handle data migration when switching between different persistence methods?
UniApp does not provide a built-in mechanism for data migration between different persistence methods. However, you can implement a custom solution to handle data migration. Here's a step-by-step approach to manage data migration in UniApp:
-
Assess Current Data:
First, assess the data currently stored in the old persistence method. Identify the structure and format of the data. -
Plan Migration Strategy:
Plan how you will migrate the data to the new persistence method. Consider the differences in data formats and structures between the old and new methods. -
Implement Migration Logic:
Write code to read data from the old persistence method and write it to the new one. This may involve transforming the data to fit the new format.Example of migrating from local storage to SQLite:
const sqlite = require('@dcloudio/uni-sqlite'); const db = new sqlite.Database('myNewDatabase.db'); db.run('CREATE TABLE IF NOT EXISTS users (id INTEGER PRIMARY KEY, name TEXT, age INTEGER)'); uni.getStorage({ key: 'userInfo', success: function (res) { const userData = JSON.parse(res.data); db.run('INSERT INTO users (name, age) VALUES (?, ?)', [userData.name, userData.age], function(err) { if (err) { console.error('Migration error:', err); } else { console.log('Data migrated successfully'); // Remove old data uni.removeStorage({ key: 'userInfo', success: function () { console.log('Old data removed'); } }); } }); } });
-
Test Migration:
Thoroughly test the migration process to ensure that all data is correctly transferred and that no data is lost or corrupted. -
Rollback Plan:
Have a rollback plan in case the migration fails. This could involve keeping the old data intact until the migration is confirmed successful. -
User Notification:
Inform users about the migration process, especially if it involves downtime or data access changes. Provide clear instructions on what to expect during and after the migration.
By following these steps, you can effectively manage data migration in UniApp when switching between different persistence methods.
The above is the detailed content of How do you handle data persistence in UniApp (e.g., using local storage, databases)?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
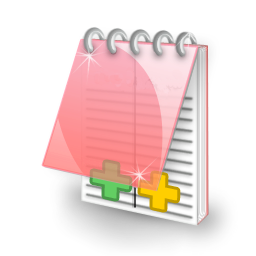
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 English version
Recommended: Win version, supports code prompts!

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
