


What are mutexes (mutual exclusion locks)? How do they prevent race conditions?
What are mutexes (mutual exclusion locks)? How do they prevent race conditions?
Mutexes, short for mutual exclusion locks, are synchronization primitives that allow only one thread to access a critical section of code at a time. This ensures that shared resources are used in a controlled manner, preventing data corruption and other concurrency-related issues.
Mutexes prevent race conditions by enforcing an order of access to shared resources. When a thread wants to enter a critical section, it must first acquire the mutex. If the mutex is already locked by another thread, the requesting thread will be blocked until the mutex is released. Once a thread successfully acquires the mutex, it can safely execute the critical section knowing that no other thread can interfere. After completing the operations, the thread releases the mutex, allowing other waiting threads to acquire it and access the shared resource.
In what scenarios are mutexes most effectively used to manage concurrent access?
Mutexes are most effectively used in scenarios where exclusive access to a shared resource is required. Common use cases include:
- Data Structures and Containers: When multiple threads need to modify a shared data structure, such as a linked list or a map, mutexes can ensure that these modifications happen atomically and safely.
- File Access: When multiple threads or processes need to read from or write to a file, a mutex can prevent simultaneous access, which could lead to data corruption or inconsistent reads.
- Database Transactions: In multi-threaded applications that interact with databases, mutexes can protect critical sections of code that perform database operations, ensuring the integrity of transactions.
- Resource Allocation: Mutexes can be used to manage the allocation of limited resources, ensuring that only one thread can allocate a resource at a time, preventing over-allocation or conflicts.
- Singleton Pattern: In multi-threaded environments, mutexes can be used to ensure that the initialization of a singleton object is thread-safe, preventing multiple instances from being created.
How do mutexes differ from semaphores in managing thread synchronization?
Mutexes and semaphores are both used for thread synchronization, but they serve different purposes and have distinct characteristics:
-
Purpose:
- Mutexes are designed to provide mutual exclusion, allowing only one thread to access a critical section at a time.
- Semaphores are more general and can be used to control access to a resource by multiple threads, based on a count. They can be used to implement both mutual exclusion and producer-consumer patterns.
-
Count:
- Mutexes have a binary state: locked or unlocked. They are typically used to protect a single resource.
- Semaphores have a count that can be greater than one, allowing a specified number of threads to access a resource simultaneously.
-
Ownership:
- Mutexes have ownership, meaning the thread that locks a mutex must be the one to unlock it. This prevents deadlocks caused by one thread locking and another trying to unlock.
- Semaphores do not have ownership; any thread can perform a wait or signal operation on a semaphore.
-
Usage:
- Mutexes are simpler and more straightforward for scenarios requiring exclusive access.
- Semaphores are more flexible and can be used in more complex scenarios, such as managing a pool of resources or implementing producer-consumer patterns.
What are the potential pitfalls of using mutexes and how can they be mitigated?
Using mutexes can introduce several potential pitfalls, but these can be mitigated with careful design and implementation:
-
Deadlocks:
- Pitfall: Deadlocks occur when two or more threads are blocked indefinitely, each waiting for the other to release a resource.
- Mitigation: Use techniques like lock ordering, timeouts, and deadlock detection algorithms. Avoid holding multiple locks simultaneously unless absolutely necessary.
-
Performance Overhead:
- Pitfall: Mutexes can introduce performance overhead due to context switching and blocking.
- Mitigation: Minimize the time spent in critical sections. Use fine-grained locking to reduce contention. Consider using lock-free algorithms or reader-writer locks where appropriate.
-
Priority Inversion:
- Pitfall: A lower-priority thread holding a mutex can delay a higher-priority thread, leading to priority inversion.
- Mitigation: Implement priority inheritance or priority ceiling protocols to ensure that a thread holding a mutex temporarily inherits the priority of the highest-priority thread waiting for it.
-
Starvation:
- Pitfall: A thread may be unable to acquire a mutex due to constant contention from other threads.
- Mitigation: Use fairness policies in mutex implementations, such as first-come, first-served (FCFS) scheduling. Consider using condition variables to allow threads to wait and be signaled when resources become available.
-
Livelock:
- Pitfall: Similar to deadlock, but threads are actively trying to resolve the situation, leading to a cycle of constant activity without progress.
- Mitigation: Implement backoff strategies or random delays to break the cycle of contention. Ensure that the retry mechanism does not lead to continuous retries without progress.
By understanding these pitfalls and applying appropriate mitigation strategies, developers can effectively use mutexes to manage concurrent access and ensure the reliability and performance of multi-threaded applications.
The above is the detailed content of What are mutexes (mutual exclusion locks)? How do they prevent race conditions?. For more information, please follow other related articles on the PHP Chinese website!
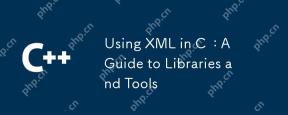
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
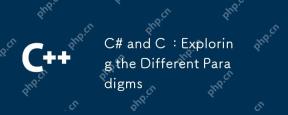
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
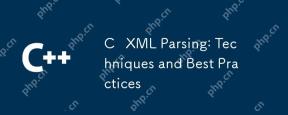
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
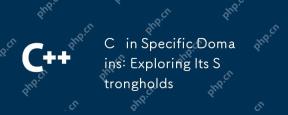
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
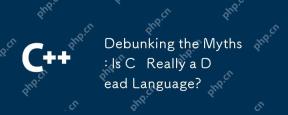
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.
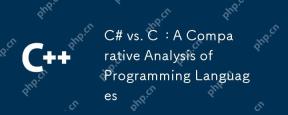
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.
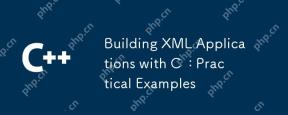
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.
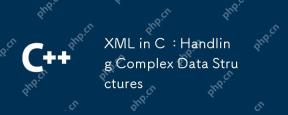
Working with XML data structures in C can use the TinyXML or pugixml library. 1) Use the pugixml library to parse and generate XML files. 2) Handle complex nested XML elements, such as book information. 3) Optimize XML processing code, and it is recommended to use efficient libraries and streaming parsing. Through these steps, XML data can be processed efficiently.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
