


What are the different types of inheritance in C (e.g., single inheritance, multiple inheritance, virtual inheritance)?
In C , inheritance is a fundamental feature of object-oriented programming that allows a class to inherit properties and methods from another class. There are several types of inheritance, each with its own characteristics and use cases:
-
Single Inheritance:
Single inheritance occurs when a derived class inherits from a single base class. This is the simplest form of inheritance and is commonly used in C programming. It provides a straightforward way to extend the functionality of a base class. -
Multiple Inheritance:
Multiple inheritance happens when a derived class inherits from more than one base class. This type of inheritance can provide more complex and flexible class hierarchies, as it allows a class to inherit behaviors from multiple sources. -
Multilevel Inheritance:
Multilevel inheritance occurs when a derived class inherits from a base class, which itself is derived from another class. This forms a chain of inheritance, allowing for more specialized classes to be created at each level. -
Hierarchical Inheritance:
Hierarchical inheritance is when multiple derived classes inherit from a single base class. This can be useful when several classes need to share common functionality from a single base class. -
Hybrid Inheritance:
Hybrid inheritance is a combination of two or more types of inheritance mentioned above. It can include multiple, multilevel, and hierarchical inheritance in a single program. -
Virtual Inheritance:
Virtual inheritance is used to solve the "diamond problem" that can occur with multiple inheritance. When two classes inherit from a common base class and another class inherits from these two classes, the common base class would be included twice in the derived class. Virtual inheritance ensures that the common base class is included only once in the derived class.
How does multiple inheritance in C differ from single inheritance, and what are its potential benefits?
Multiple inheritance and single inheritance differ in the following ways:
-
Number of Base Classes:
In single inheritance, a derived class inherits from only one base class. In multiple inheritance, a derived class can inherit from more than one base class. -
Complexity:
Multiple inheritance can lead to more complex class hierarchies and can be more challenging to manage due to potential conflicts between base classes. -
Functionality:
Multiple inheritance allows a derived class to combine functionalities from multiple base classes, which can be particularly useful for creating more versatile and specialized classes.
The potential benefits of multiple inheritance include:
-
Combining Functionalities:
It allows a class to inherit and combine the functionalities of multiple base classes, which can be useful in creating complex, feature-rich classes. -
Code Reuse:
By inheriting from multiple classes, a derived class can reuse code from all its base classes, reducing redundancy and improving maintainability. -
Flexibility:
Multiple inheritance provides more flexibility in designing class hierarchies, allowing developers to model real-world relationships more accurately.
What is the purpose of virtual inheritance in C , and when should it be used?
The purpose of virtual inheritance in C is to prevent the duplication of base class instances in situations where multiple inheritance is used. This is particularly relevant in addressing the "diamond problem," which occurs when two classes inherit from a common base class, and another class inherits from both of these classes.
In the diamond problem, without virtual inheritance, the common base class would be included twice in the final derived class, leading to ambiguity and potential conflicts. Virtual inheritance ensures that the common base class is included only once, thus avoiding these issues.
Virtual inheritance should be used in the following scenarios:
-
Preventing Duplicate Inheritance:
When dealing with the diamond problem, virtual inheritance ensures that a shared base class is inherited only once, preventing duplication. -
Maintaining a Single Instance:
If it's necessary to ensure that a class has only one instance of a particular base class, even when inheriting from multiple classes that share that base class. -
Avoiding Ambiguity:
To resolve potential ambiguities that can arise from inheriting the same method or member variable from multiple paths in the inheritance hierarchy.
Can you explain the concept of single inheritance in C and provide a simple example of its implementation?
Single inheritance in C is the process where a derived class inherits from a single base class. This allows the derived class to inherit all the public and protected members of the base class, enabling the derived class to extend or specialize the functionality of the base class.
Here is a simple example of single inheritance in C :
#include <iostream> using namespace std; // Base class class Animal { public: void eat() { cout << "The animal is eating." << endl; } }; // Derived class class Dog : public Animal { public: void bark() { cout << "The dog is barking." << endl; } }; int main() { Dog myDog; myDog.eat(); // Inherited from Animal myDog.bark(); // Defined in Dog return 0; }
In this example:
-
Animal
is the base class with a methodeat()
. -
Dog
is the derived class that inherits fromAnimal
using thepublic
access specifier, which means it can access the public members ofAnimal
. - The
Dog
class adds its own methodbark()
. - In the
main()
function, an instance ofDog
is created, and it can call botheat()
(inherited fromAnimal
) andbark()
(defined inDog
).
This demonstrates how single inheritance allows the Dog
class to inherit and extend the functionality of the Animal
class.
The above is the detailed content of What are the different types of inheritance in C (e.g., single inheritance, multiple inheritance, virtual inheritance)?. For more information, please follow other related articles on the PHP Chinese website!
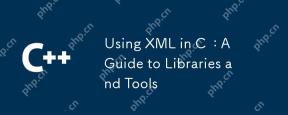
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
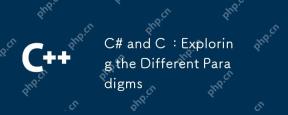
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
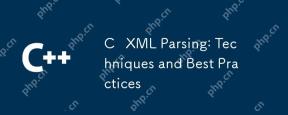
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
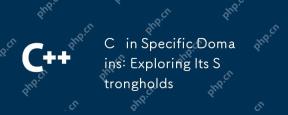
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
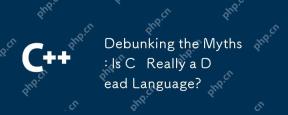
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.
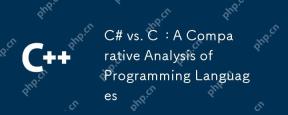
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.
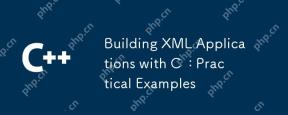
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.
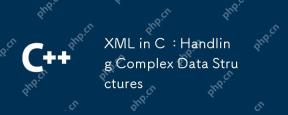
Working with XML data structures in C can use the TinyXML or pugixml library. 1) Use the pugixml library to parse and generate XML files. 2) Handle complex nested XML elements, such as book information. 3) Optimize XML processing code, and it is recommended to use efficient libraries and streaming parsing. Through these steps, XML data can be processed efficiently.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
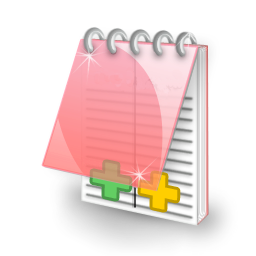
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Linux new version
SublimeText3 Linux latest version

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
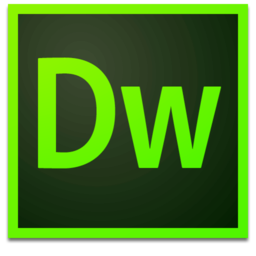
Dreamweaver Mac version
Visual web development tools
