What is the difference between stack and heap memory allocation?
Stack and heap are two different memory areas used by programs for storing variables and objects. Here are the key differences between them:
-
Structure:
- Stack: The stack follows a Last-In-First-Out (LIFO) structure. It’s a region of memory that stores temporary variables created by each function (including the main function), with the variables being pushed onto the stack when a function is called and popped off when the function returns.
- Heap: The heap is a region of memory used for dynamic memory allocation, where blocks of memory are requested by the program as needed and can be allocated and deallocated in any order.
-
Size:
- Stack: The stack has a limited size, which is determined by the operating system when the program starts. Exceeding this limit can cause a stack overflow.
- Heap: The heap is typically larger than the stack and can grow dynamically as long as there’s free memory in the system.
-
Speed:
- Stack: Accessing stack memory is fast because of its LIFO structure and because the memory is managed by the CPU directly.
- Heap: Accessing heap memory is slower because the program must ask the operating system to allocate memory, which involves more overhead.
-
Allocation and Deallocation:
- Stack: Memory allocation and deallocation on the stack are handled automatically by the compiler, which makes it very efficient.
- Heap: Memory allocation and deallocation on the heap are managed by the programmer, which can lead to fragmentation and memory leaks if not handled correctly.
How does the allocation and deallocation of memory differ between stack and heap?
Allocation and deallocation of memory between stack and heap differ significantly:
-
Allocation:
- Stack: When a function is called, a block of memory is allocated on the stack for local variables and function parameters. This allocation happens automatically as part of the function call.
-
Heap: Memory allocation on the heap is done explicitly by the programmer using functions such as
malloc
in C or thenew
operator in C . The program requests a specific amount of memory from the system, which is then allocated from the available heap memory.
-
Deallocation:
- Stack: When a function returns, the memory allocated for its local variables is automatically deallocated by popping it off the stack. This process is managed by the system and requires no action from the programmer.
-
Heap: Deallocation of heap memory must be handled explicitly by the programmer using functions such as
free
in C or thedelete
operator in C . If the programmer fails to deallocate the memory, it can lead to memory leaks.
-
Management:
- Stack: The stack is managed directly by the CPU through the stack pointer, which is updated automatically during function calls and returns.
- Heap: The heap is managed by the operating system or a runtime environment (in languages with garbage collection), and the programmer must keep track of memory allocation and deallocation.
What are the performance implications of using stack versus heap memory?
The performance implications of using stack versus heap memory are as follows:
-
Speed:
- Stack: Operations on the stack are faster because the memory is managed directly by the CPU. Allocation and deallocation are quick, as they simply involve adjusting the stack pointer.
- Heap: Operations on the heap are slower because they require additional overhead. The system must find a suitable block of memory, which may involve searching the heap, and allocation and deallocation involve more complex operations.
-
Memory Fragmentation:
- Stack: The stack doesn’t suffer from fragmentation because memory is allocated and deallocated in a strict LIFO order.
- Heap: The heap can become fragmented over time, which can reduce performance. Fragmentation occurs when blocks of memory are allocated and deallocated in a non-sequential manner, leaving gaps in the memory that are too small to be reused effectively.
-
Size Limitations:
- Stack: The stack has a fixed size, and if this size is exceeded, it can lead to a stack overflow, causing the program to crash.
- Heap: The heap can theoretically grow as large as the available memory in the system, but it is still limited by the total system memory and virtual memory settings.
-
Memory Leaks:
- Stack: Memory leaks are virtually impossible with stack memory because the system manages the deallocation automatically.
- Heap: If memory on the heap is not deallocated properly, it can lead to memory leaks, which can significantly impact the performance and stability of a program over time.
In what scenarios would you choose to use stack memory over heap memory, and vice versa?
The choice between using stack memory and heap memory depends on the specific requirements and constraints of your program. Here are some scenarios to guide your decision:
Using Stack Memory:
- Small, Temporary Variables: If you need to store variables that are small in size and only need to be used within the scope of a single function, the stack is ideal. Examples include local variables and function parameters.
- High-Performance Needs: If your application requires high performance and fast memory access, the stack's faster allocation and deallocation times make it a better choice.
- Automatic Memory Management: If you want to avoid the complexities of manual memory management, the stack's automatic allocation and deallocation can simplify your code and reduce the risk of memory leaks.
Using Heap Memory:
- Large Data Structures: When dealing with large data structures that need to persist beyond the scope of a single function, the heap is necessary. Examples include large arrays, linked lists, or objects that need to be accessed globally.
- Dynamic Memory Allocation: If your program needs to allocate memory dynamically at runtime based on user input or other variable conditions, the heap provides the flexibility to do so.
- Long-Lived Objects: If you need to create objects that will be used for a long time or throughout the lifetime of the program, the heap is more appropriate. This is common in object-oriented programming where objects are instantiated and used across multiple functions.
- Shared Resources: If you need to share data between different parts of your program or between threads, the heap can provide a common space to store such data.
In summary, the stack is best suited for small, temporary data and high-performance needs with automatic memory management, while the heap is ideal for large, dynamically allocated data that needs to persist or be shared across your program.
The above is the detailed content of What is the difference between stack and heap memory allocation?. For more information, please follow other related articles on the PHP Chinese website!
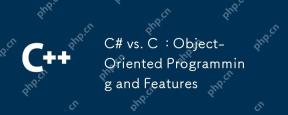
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
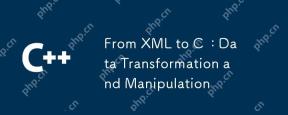
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
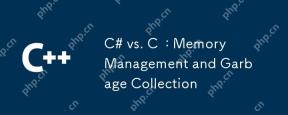
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
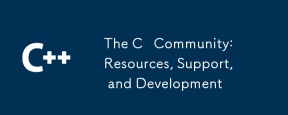
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
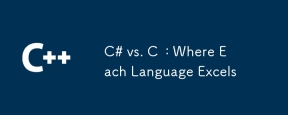
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.
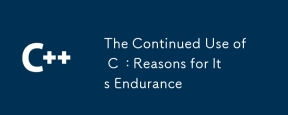
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
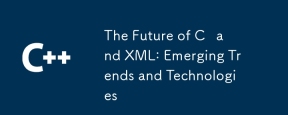
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
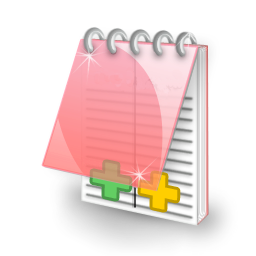
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
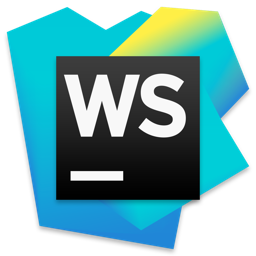
WebStorm Mac version
Useful JavaScript development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!

Zend Studio 13.0.1
Powerful PHP integrated development environment