How do you share data between different components in a UniApp project?
In a UniApp project, sharing data between different components can be achieved through several methods, each suited to different scenarios. Here are the primary ways to handle data sharing:
-
Props and Events:
-
Props: You can pass data down from a parent component to a child component using props. This is suitable for a hierarchical data flow where the parent controls the data and passes it down to children as needed.
<template> <child-component :message="parentMessage"></child-component> </template> <script> export default { data() { return { parentMessage: 'Hello from parent!' } } } </script>
-
Events: To send data from a child to a parent, you can emit events from the child and handle them in the parent. This enables communication upwards in the component tree.
<template> <child-component @child-event="handleChildEvent"></child-component> </template> <script> export default { methods: { handleChildEvent(data) { console.log('Received data from child:', data) } } } </script>
-
-
Vuex or Pinia:
-
Using a state management library like Vuex or Pinia allows you to share data across the entire application, regardless of the component hierarchy. This is particularly useful for global states and more complex applications.
// In the Vuex store const store = new Vuex.Store({ state: { count: 0 }, mutations: { increment (state) { state.count } } }) // In a component <template> <button @click="increment">Increment</button> </template> <script> import { mapMutations } from 'vuex' export default { methods: { ...mapMutations(['increment']) } } </script>
-
-
Provide/Inject:
-
This is another method for sharing data across components that are not directly connected in the component tree. It's particularly useful for injecting dependencies into deeply nested components.
// Parent component export default { provide() { return { theme: this.theme } }, data() { return { theme: 'dark' } } } // Child component export default { inject: ['theme'] }
-
Choosing the right method depends on the specific needs of your application and the complexity of the data flow required.
What are the best practices for managing state in a UniApp application?
Effective state management is crucial for building scalable and maintainable UniApp applications. Here are some best practices:
-
Centralize Global State:
- Use a state management library like Vuex or Pinia for managing global state. This helps in keeping the state centralized and makes it easier to manage and debug complex state changes.
-
Isolate Component State:
- Keep component-specific data local to the component whenever possible. This approach follows the principle of separation of concerns and makes components more reusable and easier to test.
-
Use Computed Properties and Watchers:
- Utilize computed properties for derived state and watchers for reacting to state changes. This can help in maintaining a cleaner and more reactive UI.
-
Avoid Mutating State Directly:
- Always mutate state through defined mutations or actions in your state management system. This practice makes state changes predictable and traceable.
-
Modularize the State:
- Break down the state into modules in Vuex or use different stores in Pinia. This helps in organizing the state and actions related to different parts of the application.
-
Testing and Debugging:
- Implement thorough testing, including unit tests for state mutations and actions, as well as integration tests for how components interact with the state. Utilize Vue DevTools for debugging Vuex stores.
-
Asynchronous Operations:
- Handle asynchronous operations through actions in Vuex or using composables in Pinia. Ensure that these operations are managed consistently across the application.
-
State Persistence:
- Consider using state persistence libraries like
vuex-persistedstate
to maintain state between sessions, especially for user preferences and other non-volatile data.
- Consider using state persistence libraries like
Implementing these practices will lead to a well-structured and maintainable state management system in your UniApp project.
Can you use Vuex with UniApp to facilitate data sharing across components?
Yes, you can use Vuex with UniApp to facilitate data sharing across components. UniApp is built on top of Vue.js, and Vuex is the official state management library for Vue.js, making it fully compatible with UniApp projects.
Here’s how to integrate Vuex into a UniApp project:
-
Install Vuex:
-
First, you need to install Vuex if it's not already in your project. You can do this by running:
npm install vuex --save
-
-
Create a Vuex Store:
-
Create a new file, typically named
store.js
, to define your Vuex store. Here’s a basic example of a Vuex store:import Vue from 'vue' import Vuex from 'vuex' Vue.use(Vuex) const store = new Vuex.Store({ state: { count: 0 }, mutations: { increment(state) { state.count } }, actions: { incrementAsync({ commit }) { setTimeout(() => { commit('increment') }, 1000) } } }) export default store
-
-
Integrate the Store with UniApp:
-
In your main application file (e.g.,
main.js
), import and use the store:import Vue from 'vue' import App from './App' import store from './store' Vue.prototype.$store = store const app = new Vue({ store, ...App }) app.$mount()
-
-
Accessing the Store in Components:
-
Once the store is set up, you can access it in any component using
this.$store
. Here’s an example of how to use it in a component:<template> <view> <text>{{ $store.state.count }}</text> <button @click="increment">Increment</button> <button @click="incrementAsync">Increment Async</button> </view> </template> <script> export default { methods: { increment() { this.$store.commit('increment') }, incrementAsync() { this.$store.dispatch('incrementAsync') } } } </script>
-
By integrating Vuex, you can manage the application’s state in a centralized and predictable way, making it easier to share data across components in a UniApp project.
How do you handle data synchronization issues when sharing data between components in a UniApp project?
Handling data synchronization issues when sharing data between components in a UniApp project is crucial for maintaining a consistent and responsive application. Here are some strategies and best practices to manage data synchronization effectively:
-
Use a Single Source of Truth:
- Implement a centralized state management system like Vuex or Pinia. This ensures that all components reference the same data source, thereby reducing the risk of data inconsistencies.
-
Reactive Data Handling:
- Utilize Vue’s reactivity system to automatically update components when the state changes. This means when the state in Vuex changes, all components that depend on that state will automatically re-render with the new data.
-
Optimistic UI Updates:
- For operations that involve server-side interactions, such as updating or deleting data, you can implement optimistic UI updates. This involves updating the UI immediately based on the user’s action and then synchronizing the changes with the server in the background. If the server rejects the change, you can revert the UI state.
-
Debounce and Throttle:
- When dealing with frequent updates, such as user inputs or scrolling, use debounce and throttle techniques to limit the rate at which updates are sent to the server. This can prevent overwhelming the server and reduce the chances of data synchronization issues.
-
Error Handling and Retrying:
- Implement robust error handling and retry mechanisms for failed data synchronization attempts. This could involve catching network errors, logging them, and providing options for users to retry the operation.
-
Version Control and Conflict Resolution:
- For collaborative applications where multiple users might be editing the same data, implement a versioning system to track changes. Use conflict resolution strategies like ‘last write wins’ or more sophisticated merge algorithms based on the application’s needs.
-
Real-time Synchronization:
- If real-time data updates are crucial, consider using technologies like WebSockets or server-sent events to keep the client synchronized with the server. Libraries like Socket.IO can be integrated with UniApp for real-time communication.
-
Offline Support and Syncing:
- For applications that need to function offline, store data locally using technologies like IndexedDB or localStorage. Implement a synchronization mechanism that queues offline changes and syncs them when the device reconnects.
Here’s an example of how you might use Vuex to manage data synchronization in a UniApp project:
// store.js import Vue from 'vue' import Vuex from 'vuex' Vue.use(Vuex) const store = new Vuex.Store({ state: { todos: [] }, mutations: { addTodo(state, todo) { state.todos.push(todo) }, removeTodo(state, id) { state.todos = state.todos.filter(todo => todo.id !== id) } }, actions: { async addTodo({ commit }, todo) { // Optimistic UI update commit('addTodo', todo) try { // Sync with server await api.addTodo(todo) } catch (error) { // Revert UI state if server rejects the change commit('removeTodo', todo.id) console.error('Failed to add todo:', error) } } } }) export default store
By following these strategies, you can effectively manage data synchronization issues and ensure that your UniApp project remains consistent and reliable across different components and user interactions.
The above is the detailed content of How do you share data between different components in a UniApp project?. For more information, please follow other related articles on the PHP Chinese website!
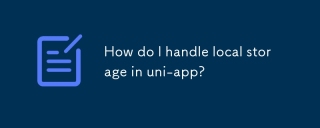
This article details uni-app's local storage APIs (uni.setStorageSync(), uni.getStorageSync(), and their async counterparts), emphasizing best practices like using descriptive keys, limiting data size, and handling JSON parsing. It stresses that lo
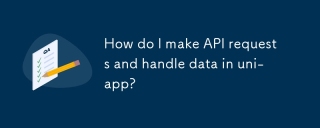
This article details making and securing API requests within uni-app using uni.request or Axios. It covers handling JSON responses, best security practices (HTTPS, authentication, input validation), troubleshooting failures (network issues, CORS, s
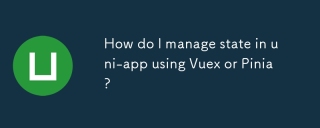
This article compares Vuex and Pinia for state management in uni-app. It details their features, implementation, and best practices, highlighting Pinia's simplicity versus Vuex's structure. The choice depends on project complexity, with Pinia suita

This article details uni-app's geolocation APIs, focusing on uni.getLocation(). It addresses common pitfalls like incorrect coordinate systems (gcj02 vs. wgs84) and permission issues. Improving location accuracy via averaging readings and handling
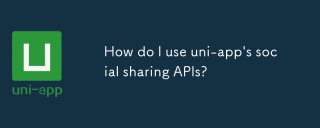
The article details how to integrate social sharing into uni-app projects using uni.share API, covering setup, configuration, and testing across platforms like WeChat and Weibo.
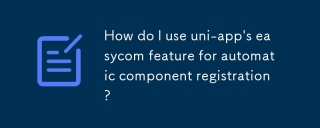
This article explains uni-app's easycom feature, automating component registration. It details configuration, including autoscan and custom component mapping, highlighting benefits like reduced boilerplate, improved speed, and enhanced readability.
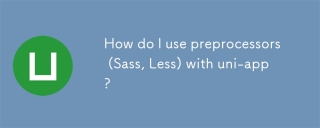
Article discusses using Sass and Less preprocessors in uni-app, detailing setup, benefits, and dual usage. Main focus is on configuration and advantages.[159 characters]
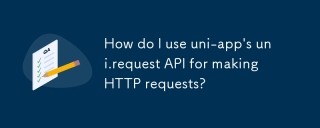
This article details uni.request API in uni-app for making HTTP requests. It covers basic usage, advanced options (methods, headers, data types), robust error handling techniques (fail callbacks, status code checks), and integration with authenticat


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
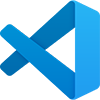
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.