


How does Vue 3's reactivity system differ from Vue 2's reactivity system?
Vue 3's reactivity system introduces significant changes and improvements over Vue 2's system. The primary difference lies in the underlying mechanism used to track and react to changes in the application state.
In Vue 2, the reactivity system was based on Object.defineProperty, which allowed Vue to intercept property access and modifications. However, this approach had limitations, such as the inability to detect property additions or deletions dynamically. Vue 2's system also required the use of Vue.set and Vue.delete to handle these operations.
Vue 3, on the other hand, adopts a new reactivity system based on ES6 Proxies. This change allows for more comprehensive reactivity tracking, including the ability to detect property additions and deletions without the need for additional methods like Vue.set and Vue.delete. The Proxy-based system in Vue 3 can observe changes to nested objects and arrays more efficiently, providing a more robust and flexible reactivity model.
Additionally, Vue 3 introduces the concept of "reactive" and "ref" for managing state. The reactive
function is used to create reactive objects, while ref
is used for creating reactive primitive values. These new APIs provide a more explicit and fine-grained control over reactivity, which was not as straightforward in Vue 2.
What performance improvements does Vue 3's reactivity system offer over Vue 2?
Vue 3's reactivity system brings several performance improvements over Vue 2, primarily due to the use of ES6 Proxies and a more efficient dependency tracking mechanism.
- Finer Granularity: Vue 3's system tracks dependencies at a more granular level, meaning that only the components that actually use a piece of reactive data will be re-rendered when that data changes. This reduces unnecessary re-renders, leading to better performance, especially in large applications.
- Tree-Shaking: Vue 3 is designed with tree-shaking in mind, allowing unused features to be eliminated from the final bundle. This results in smaller bundle sizes and faster load times.
- Improved Compilation: The new compiler in Vue 3 can generate more optimized render functions, which contribute to faster rendering and better performance.
- Reduced Overhead: The use of Proxies in Vue 3 reduces the overhead of setting up reactivity compared to the Object.defineProperty approach in Vue 2. This leads to faster initial rendering and better performance during runtime.
How does the new reactivity system in Vue 3 affect the way developers write code compared to Vue 2?
The new reactivity system in Vue 3 influences the way developers write code in several ways, primarily by introducing new APIs and changing the approach to managing state and reactivity.
-
Use of
reactive
andref
: Developers now usereactive
to create reactive objects andref
to create reactive primitive values. This is a shift from Vue 2, where reactivity was primarily managed through thedata
option in components.// Vue 3 import { reactive, ref } from 'vue'; const state = reactive({ count: 0 }); const count = ref(0);
- Explicit Reactivity: Vue 3 encourages a more explicit approach to reactivity. Developers need to be more aware of when and how they are making data reactive, which can lead to more predictable and manageable code.
-
Composition API: The new reactivity system is closely tied to the Composition API, which allows developers to organize their code into reusable functions. This is a significant shift from Vue 2's Options API, where code was organized by options like
data
,methods
, andcomputed
.// Vue 3 with Composition API import { ref, onMounted } from 'vue'; export default { setup() { const count = ref(0); onMounted(() => { console.log('Component mounted'); }); return { count }; } };
-
Handling Nested Objects: With Proxies, developers no longer need to use methods like
Vue.set
to make nested objects reactive. This simplifies the code and reduces the chance of missing reactivity.
Can you explain the concept of reactivity in Vue 3 and how it's implemented differently from Vue 2?
Reactivity in Vue 3 refers to the ability of the framework to automatically detect changes to the application state and update the DOM accordingly. This is a core feature that allows Vue applications to be highly interactive and responsive.
In Vue 3, reactivity is implemented using ES6 Proxies, which provide a more powerful and flexible way to track changes compared to Vue 2's Object.defineProperty approach. Here's how it works differently:
-
Proxy-Based Reactivity: Vue 3 uses Proxies to wrap reactive objects. When a property of a reactive object is accessed or modified, the Proxy intercepts these operations and triggers the necessary updates. This allows Vue 3 to detect changes to nested objects and arrays without additional setup.
// Vue 3 const obj = reactive({ a: 1, b: { c: 2 } }); obj.b.c = 3; // This change is automatically detected and tracked
-
Reactive and Ref APIs: Vue 3 introduces
reactive
andref
APIs to create reactive objects and primitive values, respectively. These APIs provide a more explicit way to manage reactivity, allowing developers to control which parts of their state are reactive.// Vue 3 const count = ref(0); const state = reactive({ count: 0 });
- Dependency Tracking: Vue 3's reactivity system tracks dependencies more efficiently. When a piece of reactive data is used in a component, Vue 3 creates a dependency link between the data and the component. When the data changes, only the components that depend on it are updated, reducing unnecessary re-renders.
In contrast, Vue 2's reactivity system relied on Object.defineProperty, which had limitations in detecting changes to nested objects and arrays. Vue 2 required additional methods like Vue.set
and Vue.delete
to handle these cases, making the code more verbose and error-prone.
Overall, Vue 3's reactivity system offers a more robust, efficient, and developer-friendly approach to managing state and reactivity in Vue applications.
The above is the detailed content of How does Vue 3's reactivity system differ from Vue 2's reactivity system?. For more information, please follow other related articles on the PHP Chinese website!

Classselectorsarereusableformultipleelements,whileIDselectorsareuniqueandusedonceperpage.1)Classes,denotedbyaperiod(.),areidealforstylingmultipleelementslikebuttons.2)IDs,denotedbyahash(#),areperfectforuniqueelementslikeanavigationmenu.3)IDshavehighe

In CSS style, the class selector or ID selector should be selected according to the project requirements: 1) The class selector is suitable for reuse and is suitable for the same style of multiple elements; 2) The ID selector is suitable for unique elements and has higher priority, but should be used with caution to avoid maintenance difficulties.

HTML5hasseverallimitationsincludinglackofsupportforadvancedgraphics,basicformvalidation,cross-browsercompatibilityissues,performanceimpacts,andsecurityconcerns.1)Forcomplexgraphics,HTML5'scanvasisinsufficient,requiringlibrarieslikeWebGLorThree.js.2)I

Yes,onestylecanhavemoreprioritythananotherinCSSduetospecificityandthecascade.1)Specificityactsasascoringsystemwheremorespecificselectorshavehigherpriority.2)Thecascadedeterminesstyleapplicationorder,withlaterrulesoverridingearlieronesofequalspecifici

ThesignificantgoalsofHTML5aretoenhancemultimediasupport,ensurehumanreadability,maintainconsistencyacrossdevices,andensurebackwardcompatibility.1)HTML5improvesmultimediawithnativeelementslikeand.2)ItusessemanticelementsforbetterreadabilityandSEO.3)Its

React'slimitationsinclude:1)asteeplearningcurveduetoitsvastecosystem,2)SEOchallengeswithclient-siderendering,3)potentialperformanceissuesinlargeapplications,4)complexstatemanagementasappsgrow,and5)theneedtokeepupwithitsrapidevolution.Thesefactorsshou

Reactischallengingforbeginnersduetoitssteeplearningcurveandparadigmshifttocomponent-basedarchitecture.1)Startwithofficialdocumentationforasolidfoundation.2)UnderstandJSXandhowtoembedJavaScriptwithinit.3)Learntousefunctionalcomponentswithhooksforstate

ThecorechallengeingeneratingstableanduniquekeysfordynamiclistsinReactisensuringconsistentidentifiersacrossre-rendersforefficientDOMupdates.1)Usenaturalkeyswhenpossible,astheyarereliableifuniqueandstable.2)Generatesynthetickeysbasedonmultipleattribute


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
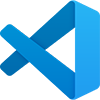
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Zend Studio 13.0.1
Powerful PHP integrated development environment
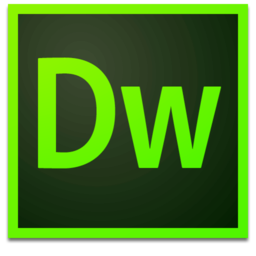
Dreamweaver Mac version
Visual web development tools
