


What are generators and iterators in Python? How do they differ, and when would you use each?
What are generators and iterators in Python? How do they differ, and when would you use each?
In Python, both generators and iterators are used for implementing iterables, but they serve slightly different purposes and have different implementations.
Iterators: An iterator is an object that implements the iterator protocol, which consists of the methods __iter__()
and __next__()
. The __iter__()
method returns the iterator object itself, and __next__()
method returns the next item in the sequence. When there are no more items to return, it raises the StopIteration
exception.
Iterators are useful when you need to iterate over a sequence of data but don't need to keep the entire sequence in memory. They are commonly used with data structures like lists, tuples, and dictionaries. For example, you can create a custom iterator to iterate over a specific pattern in a sequence.
Generators: Generators are a special type of iterator that are easier to create. They use the yield
statement to return data one at a time, and they maintain their state between calls. You can create a generator function using the def
keyword with yield
inside it, or you can use generator expressions, which are similar to list comprehensions but use parentheses instead of square brackets.
Generators are particularly useful when you want to generate a large sequence of data but want to compute it lazily, i.e., only when the data is needed. This is beneficial for handling large datasets or infinite sequences.
Key Differences:
-
Implementation: Iterators are implemented with methods, whereas generators are implemented with functions that use
yield
. - State Management: Generators automatically handle state, whereas you must manually manage state in iterators.
- Creation: Generators can be created using simpler syntax compared to iterators.
When to Use Each:
- Use iterators when you need more control over the iteration process or when you need to create custom iteration behavior.
- Use generators when you want to create an iterable sequence that can be iterated over lazily, without having to store the entire sequence in memory.
How can using generators in Python improve memory efficiency in your programs?
Generators can significantly improve memory efficiency in Python programs by allowing for lazy evaluation. Here’s how:
- Lazy Evaluation: Generators produce values one at a time, only when they are requested, instead of computing and storing all values upfront. This means that you only need to keep a single value in memory at any given time, rather than the entire sequence.
- Handling Large Datasets: If you need to process a large dataset, using a generator can prevent your program from running out of memory. For example, if you are reading a large file line by line, a generator can yield each line as it is read, without needing to load the entire file into memory.
- Infinite Sequences: Generators are ideal for creating infinite sequences, such as an infinite series of numbers. You can iterate over these sequences without running into memory issues because only the requested values are generated.
- Pipelining Operations: Generators can be used to create pipelines of operations where each step in the pipeline can be processed independently and sequentially, reducing the overall memory footprint.
For instance, if you have a function that generates prime numbers:
def primes(): number = 2 while True: if all(number % prime != 0 for prime in primes_helper()): yield number number = 1 def primes_helper(): number = 2 while True: yield number number = 1 # Usage prime_gen = primes() for _ in range(10): print(next(prime_gen))
In this example, the primes()
function will generate prime numbers one at a time, allowing you to process as many as needed without storing all of them in memory.
What are some practical examples where iterators would be more suitable than generators in Python?
While generators are great for lazy evaluation, there are scenarios where using iterators might be more suitable:
-
Custom Iteration Logic: If you need more complex iteration logic that goes beyond simple yield statements, iterators can provide the necessary flexibility. For instance, if you need to iterate over a tree structure and you need to manage the state manually:
class TreeNode: def __init__(self, value, children=None): self.value = value self.children = children if children is not None else [] class TreeIterator: def __init__(self, root): self.stack = [root] def __iter__(self): return self def __next__(self): if not self.stack: raise StopIteration node = self.stack.pop() self.stack.extend(reversed(node.children)) return node.value # Usage root = TreeNode(1, [TreeNode(2), TreeNode(3, [TreeNode(4), TreeNode(5)])]) for value in TreeIterator(root): print(value)
This example demonstrates a custom iterator for traversing a tree structure, which might be more complex to implement using a generator due to the need to manage the stack manually.
- Integration with Existing Classes: When you have existing classes that need to be made iterable, you might prefer using iterators to modify their behavior without altering the class structure too much.
- Performance-Critical Code: In some cases, iterators might perform better than generators, especially when dealing with small sequences where the overhead of generator functions might be significant.
In what scenarios would you choose to implement a generator over an iterator in Python?
Choosing a generator over an iterator is appropriate in the following scenarios:
-
Large or Infinite Data Sequences: When dealing with large datasets or infinite sequences, generators are the natural choice because they allow for lazy evaluation. For example, if you are generating a Fibonacci sequence:
def fibonacci(): a, b = 0, 1 while True: yield a a, b = b, a b # Usage fib_gen = fibonacci() for _ in range(10): print(next(fib_gen))
-
Simplified Code: Generators often lead to more concise and readable code. The
yield
statement simplifies the process of generating values one at a time, which can be more intuitive than manually managing state in iterators. -
Memory Efficiency: If memory usage is a concern, generators are preferred because they do not require storing the entire dataset in memory. This is particularly useful when working with large files or streams of data:
def read_large_file(file_path): with open(file_path, 'r') as file: for line in file: yield line.strip() # Usage for line in read_large_file('large_file.txt'): process(line)
-
Chaining Operations: Generators can be easily chained together to create pipelines of operations, which can be useful for processing data in stages without needing to store intermediate results:
def process_data(data_gen): for item in data_gen: processed_item = transform(item) yield processed_item def transform(item): # Some transformation logic return item.upper() # Usage data_gen = (line for line in open('data.txt')) processed_gen = process_data(data_gen) for item in processed_gen: print(item)
In summary, generators are preferred in scenarios where lazy evaluation, memory efficiency, and code simplicity are important, whereas iterators are better suited for cases where custom iteration logic or integration with existing classes is required.
The above is the detailed content of What are generators and iterators in Python? How do they differ, and when would you use each?. For more information, please follow other related articles on the PHP Chinese website!
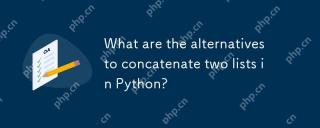
There are many methods to connect two lists in Python: 1. Use operators, which are simple but inefficient in large lists; 2. Use extend method, which is efficient but will modify the original list; 3. Use the = operator, which is both efficient and readable; 4. Use itertools.chain function, which is memory efficient but requires additional import; 5. Use list parsing, which is elegant but may be too complex. The selection method should be based on the code context and requirements.
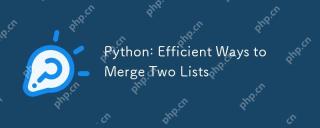
There are many ways to merge Python lists: 1. Use operators, which are simple but not memory efficient for large lists; 2. Use extend method, which is efficient but will modify the original list; 3. Use itertools.chain, which is suitable for large data sets; 4. Use * operator, merge small to medium-sized lists in one line of code; 5. Use numpy.concatenate, which is suitable for large data sets and scenarios with high performance requirements; 6. Use append method, which is suitable for small lists but is inefficient. When selecting a method, you need to consider the list size and application scenarios.
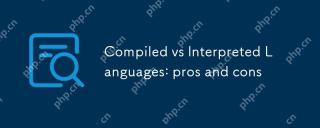
Compiledlanguagesofferspeedandsecurity,whileinterpretedlanguagesprovideeaseofuseandportability.1)CompiledlanguageslikeC arefasterandsecurebuthavelongerdevelopmentcyclesandplatformdependency.2)InterpretedlanguageslikePythonareeasiertouseandmoreportab
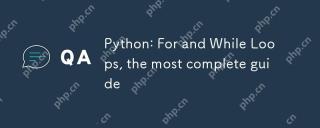
In Python, a for loop is used to traverse iterable objects, and a while loop is used to perform operations repeatedly when the condition is satisfied. 1) For loop example: traverse the list and print the elements. 2) While loop example: guess the number game until you guess it right. Mastering cycle principles and optimization techniques can improve code efficiency and reliability.
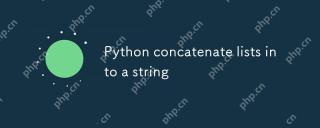
To concatenate a list into a string, using the join() method in Python is the best choice. 1) Use the join() method to concatenate the list elements into a string, such as ''.join(my_list). 2) For a list containing numbers, convert map(str, numbers) into a string before concatenating. 3) You can use generator expressions for complex formatting, such as ','.join(f'({fruit})'forfruitinfruits). 4) When processing mixed data types, use map(str, mixed_list) to ensure that all elements can be converted into strings. 5) For large lists, use ''.join(large_li
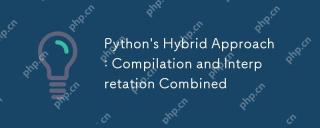
Pythonusesahybridapproach,combiningcompilationtobytecodeandinterpretation.1)Codeiscompiledtoplatform-independentbytecode.2)BytecodeisinterpretedbythePythonVirtualMachine,enhancingefficiencyandportability.
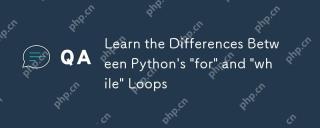
ThekeydifferencesbetweenPython's"for"and"while"loopsare:1)"For"loopsareidealforiteratingoversequencesorknowniterations,while2)"while"loopsarebetterforcontinuinguntilaconditionismetwithoutpredefinediterations.Un
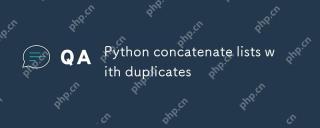
In Python, you can connect lists and manage duplicate elements through a variety of methods: 1) Use operators or extend() to retain all duplicate elements; 2) Convert to sets and then return to lists to remove all duplicate elements, but the original order will be lost; 3) Use loops or list comprehensions to combine sets to remove duplicate elements and maintain the original order.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Chinese version
Chinese version, very easy to use

SublimeText3 Linux new version
SublimeText3 Linux latest version
