


What are the key benefits of using the Singleton pattern in PHP applications?
The Singleton pattern in PHP is a creational design pattern that restricts the instantiation of a class to a single instance. This pattern is useful in scenarios where exactly one object is needed to coordinate actions across the system. Here are some key benefits of using the Singleton pattern in PHP applications:
- Controlled Access to a Single Instance: The Singleton pattern ensures that only one instance of the class is created, which can be useful for managing system-wide resources such as database connections or loggers. This can prevent the overhead of creating multiple instances of resource-intensive objects.
- Global Point of Access: The Singleton pattern provides a global point of access to that instance, which simplifies the code by eliminating the need to pass the object reference around. It’s particularly useful for utility classes where it’s desirable to have a single point of access to the utility methods.
- Memory Efficiency: By limiting the number of instances, the Singleton pattern can lead to more efficient memory usage. This is particularly important in environments with limited resources, where creating multiple instances could be wasteful.
- Easier Configuration Management: Since there's only one instance, configuration management becomes easier. For example, setting up a logging system where a single logger instance manages all logs across an application can be achieved more easily with a Singleton.
- Lazy Initialization: The Singleton pattern often implements lazy initialization, meaning the instance is created only when it’s needed, which can contribute to performance improvements.
However, it's important to use the Singleton pattern judiciously, as overuse can lead to issues such as tight coupling and difficulties in unit testing.
How can the Factory pattern improve code maintainability in PHP projects?
The Factory pattern is another creational design pattern that provides an interface for creating objects in a superclass but allows subclasses to alter the type of objects that will be created. Here’s how it can improve code maintainability in PHP projects:
- Decoupling Object Creation from Usage: The Factory pattern separates the process of creating an object from the code that uses the object. This separation reduces dependencies and makes the code more modular and easier to maintain.
- Flexibility and Extensibility: By using a factory, you can easily introduce new types of objects without changing the existing code that uses the factory. This makes it easier to extend the system without affecting existing functionality.
- Centralized Object Creation Logic: The Factory pattern centralizes the logic for creating objects, which makes it easier to manage and modify the creation process. If the creation logic needs to change, you only need to modify the factory class.
- Improved Testability: With the Factory pattern, you can easily mock or stub the factory in unit tests, which makes testing more straightforward and less dependent on the actual implementation of the objects being created.
- Consistency in Object Creation: The Factory pattern ensures that objects are created in a consistent manner, which can help prevent errors that might occur if objects were created directly in multiple places throughout the codebase.
By implementing the Factory pattern, developers can create more maintainable and scalable PHP applications.
What are some practical scenarios where the Observer pattern is particularly useful in PHP?
The Observer pattern is a behavioral design pattern that defines a one-to-many dependency between objects so that when one object changes state, all its dependents are notified and updated automatically. Here are some practical scenarios where the Observer pattern is particularly useful in PHP:
- Event-Driven Systems: In applications where events trigger actions, such as user interface components reacting to user inputs, the Observer pattern can be used to notify components of changes. For example, in a web application, when a user submits a form, various parts of the application might need to be updated or notified.
- Real-Time Data Updates: In scenarios where data needs to be updated in real-time, such as stock market applications or live sports scores, the Observer pattern can be used to push updates to all interested parties as soon as the data changes.
- Logging and Monitoring: The Observer pattern can be used to implement logging and monitoring systems where multiple loggers or monitors need to be notified of system events. For example, when an error occurs, different loggers might need to record the error in different ways.
- Model-View-Controller (MVC) Frameworks: In MVC frameworks, the Observer pattern is often used to keep the view in sync with the model. When the model changes, the view is notified and updated accordingly, ensuring that the user interface reflects the current state of the data.
- Content Management Systems (CMS): In CMS applications, the Observer pattern can be used to notify different parts of the system when content is updated. For example, when a new article is published, various modules might need to be notified to update caches, generate sitemaps, or trigger notifications.
By using the Observer pattern, developers can create more flexible and responsive PHP applications that can handle complex interactions and dependencies between objects.
How can the Strategy pattern enhance the flexibility of PHP applications?
The Strategy pattern is a behavioral design pattern that defines a family of algorithms, encapsulates each one, and makes them interchangeable. This pattern lets the algorithm vary independently from clients that use it. Here’s how the Strategy pattern can enhance the flexibility of PHP applications:
- Interchangeable Algorithms: The Strategy pattern allows different algorithms to be selected at runtime, which makes it easy to switch between different strategies without changing the client code. For example, in a payment processing system, you might have different payment strategies (e.g., credit card, PayPal, bank transfer) that can be swapped out as needed.
- Open/Closed Principle: The Strategy pattern adheres to the Open/Closed Principle, which states that software entities should be open for extension but closed for modification. By using the Strategy pattern, you can add new strategies without modifying existing code, which enhances the flexibility and maintainability of the application.
- Decoupling: The Strategy pattern decouples the client code from the specific algorithm implementation, which reduces dependencies and makes the code more modular. This decoupling allows for easier testing and maintenance.
- Improved Code Reusability: By encapsulating algorithms in separate strategy classes, you can reuse these strategies across different parts of the application or even in different applications, which promotes code reuse and reduces duplication.
- Easier Configuration and Customization: The Strategy pattern makes it easier to configure and customize the behavior of an application. For example, in a sorting application, you might have different sorting strategies (e.g., quicksort, mergesort, insertion sort) that can be configured based on the specific needs of the application.
By implementing the Strategy pattern, developers can create more flexible and adaptable PHP applications that can easily accommodate changes and new requirements.
The above is the detailed content of PHP Design Patterns Examples: Singleton, Factory, Observer, Strategy.. For more information, please follow other related articles on the PHP Chinese website!
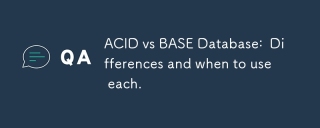
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
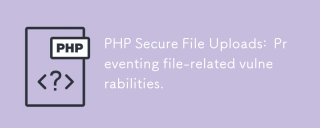
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
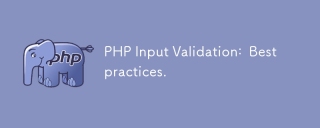
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
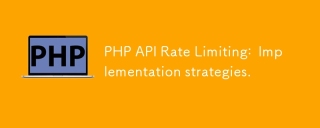
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
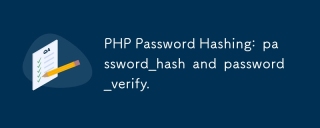
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
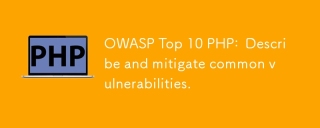
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
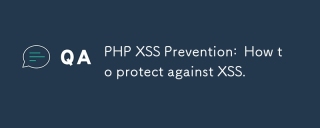
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

Atom editor mac version download
The most popular open source editor
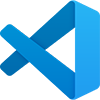
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Zend Studio 13.0.1
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software