How do you mock dependencies in your Go tests?
In Go, mocking dependencies for tests is typically achieved by using interfaces and a mocking library or by manually creating mock objects. Here’s a step-by-step process on how to do it:
-
Define an Interface: First, define an interface for the dependency you want to mock. For example, if you want to mock an HTTP client, you could define an interface like this:
type HTTPClient interface { Do(req *http.Request) (*http.Response, error) }
-
Use the Interface in Your Code: Modify your production code to use this interface rather than the concrete type. For instance, instead of using
http.Client
directly, you might use a struct field of typeHTTPClient
. -
Create a Mock: Use a mocking library like
go-mock
ortestify/mock
to generate or manually create a mock implementation of the interface. Here’s an example usingtestify/mock
:type MockHTTPClient struct { mock.Mock } func (m *MockHTTPClient) Do(req *http.Request) (*http.Response, error) { args := m.Called(req) return args.Get(0).(*http.Response), args.Error(1) }
-
Configure the Mock in Your Test: In your test, create an instance of the mock and configure it to behave in the way you want. Here’s how you might do this:
func TestMyFunction(t *testing.T) { mockClient := new(MockHTTPClient) expectedResponse := &http.Response{StatusCode: 200} mockClient.On("Do", mock.Anything).Return(expectedResponse, nil) // Use the mock client in your test myFunction(mockClient) // Assert that the mock was called as expected mockClient.AssertExpectations(t) }
By following these steps, you can effectively mock dependencies in your Go tests, allowing you to isolate the code you are testing from its dependencies.
What are the best practices for using mock objects in Go unit testing?
Using mock objects effectively in Go unit testing requires adherence to several best practices to ensure that your tests are reliable, maintainable, and truly unit tests. Here are some key best practices:
- Use Interfaces: Always mock against interfaces rather than concrete types. This allows for better decoupling and easier mocking.
- Keep Mocks Simple: Your mocks should be as simple as possible. Complex mocks can lead to complex tests, which can be hard to maintain and understand.
- Focus on Behavior: Mocks should be used to test the behavior of your code, not to replicate every single aspect of a dependency. Define clear expectations on what the mock should do.
- Minimize Mocking: Only mock what is necessary. Over-mocking can lead to tests that are brittle and slow to run. Consider using real objects when possible, especially for simple dependencies.
-
Use Mocking Libraries: Utilize libraries like
go-mock
,testify/mock
, orgomock
to reduce the boilerplate and increase the readability of your tests. - Validate Interactions: Ensure that your mocks are called as expected. This helps in verifying the flow of your code under test.
- Isolation: Ensure that your tests are truly unit tests by isolating them from each other and from external dependencies. Mocks are great for this.
- Clear Naming: Use clear and descriptive names for your mock objects and their methods. This helps in understanding the purpose of the mock at a glance.
- Error Handling: Mock error cases as well as success cases to ensure your code handles errors appropriately.
- Test Documentation: Document your tests well, especially when using mocks. Explain why you are using a mock and what behavior you are trying to test.
By following these practices, you can ensure that your use of mocks in Go unit testing is effective and contributes positively to your testing strategy.
How can you ensure that your mocks in Go tests accurately simulate real dependencies?
Ensuring that mocks in Go tests accurately simulate real dependencies is crucial for maintaining the reliability and effectiveness of your tests. Here are several strategies to help achieve this:
- Understand the Real Dependency: Before mocking, thoroughly understand the behavior of the real dependency. This includes understanding its inputs, outputs, and any side effects.
- Define Clear Expectations: Clearly define what you expect the mock to do. Use specific expectations for mock behavior, including what methods are called, with what arguments, and what they should return.
- Test Against Real Data: When possible, use real data in your tests. This helps ensure that the mock behaves similarly to how the real dependency would with real data.
-
Verify Mock Behavior: Use assertions in your tests to verify that the mock behaves as expected. For example, with
testify/mock
, you can useAssertExpectations
to check if the mock was called as intended. - Mock Error Cases: Don’t just mock happy paths. Also mock error conditions to ensure your code handles errors correctly. This helps simulate the full spectrum of real-world scenarios.
- Use Real Dependencies in Integration Tests: While unit tests might use mocks, integration tests should use real dependencies where possible. This helps validate that mocks are indeed accurate reflections of real dependencies.
- Regularly Review and Update Mocks: As the real dependency evolves, so should your mocks. Regularly review and update mocks to reflect any changes in the real dependency's behavior.
- Compare Mock and Real Outputs: In some cases, you might want to run your tests with both mocks and real dependencies to compare outputs. This can help identify discrepancies.
- Mock at the Right Level: Mock at the appropriate level of abstraction. If you’re mocking a complex system, consider mocking at a higher level rather than individual methods to better simulate real behavior.
- Document Assumptions: Clearly document any assumptions made about the behavior of the dependency. This documentation can help others understand the context and limitations of the mock.
By implementing these strategies, you can enhance the accuracy of your mocks and, in turn, the reliability of your Go tests.
What tools or libraries are recommended for mocking in Go testing?
There are several tools and libraries available for mocking in Go testing, each with its own set of features and use cases. Here are some of the most recommended ones:
-
GoMock:
- Description: GoMock is a popular mocking framework that generates mocks from interfaces using a code generator. It’s part of the official Go tools.
- Pros: Easy to use, integrates well with the Go ecosystem, and generates type-safe mocks.
- Cons: Requires a separate step to generate mocks, which can be a bit cumbersome.
-
Example Use: You can use
mockgen
to generate mock implementations from interfaces.
-
Testify/Mock:
-
Description: Part of the
testify
suite,testify/mock
is a mocking framework that allows you to define mocks directly in your test code. - Pros: No need for a separate generation step, easy to set up and use directly in tests.
- Cons: Can be less type-safe than generated mocks, as you need to handle type assertions manually.
- Example Use: Define a mock struct that implements your interface and use it directly in your tests.
-
Description: Part of the
-
Gomock:
- Description: Gomock is another mocking library that provides an easy-to-use API for creating mocks directly in your test code.
- Pros: Lightweight, easy to integrate into your test suite, and no need for code generation.
-
Cons: Similar to
testify/mock
, it may require more manual type handling. - Example Use: Create a mock implementation in your test code and configure it to respond to method calls.
-
Mockery:
- Description: Mockery is a tool that generates mocks for interfaces in Go, similar to GoMock but with a different approach and configuration.
- Pros: Generates mocks quickly, customizable, and supports multiple output formats.
- Cons: Requires a separate step to generate mocks, similar to GoMock.
-
Example Use: Use the
mockery
command to generate mocks based on your interfaces.
-
Gock:
- Description: Gock is specifically designed for mocking HTTP requests, making it useful for testing code that makes network calls.
- Pros: Focused on HTTP mocking, easy to set up and use for network-related tests.
- Cons: Limited to HTTP mocking, not suitable for general mocking needs.
- Example Use: Intercept and mock HTTP requests in your tests.
Each of these tools has its strengths and is suitable for different testing needs. The choice depends on your specific requirements, whether you prefer generated mocks or direct mocking in your tests, and the nature of the dependencies you are testing.
The above is the detailed content of How do you mock dependencies in your Go tests?. For more information, please follow other related articles on the PHP Chinese website!
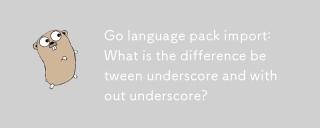
This article explains Go's package import mechanisms: named imports (e.g., import "fmt") and blank imports (e.g., import _ "fmt"). Named imports make package contents accessible, while blank imports only execute t

This article explains Beego's NewFlash() function for inter-page data transfer in web applications. It focuses on using NewFlash() to display temporary messages (success, error, warning) between controllers, leveraging the session mechanism. Limita
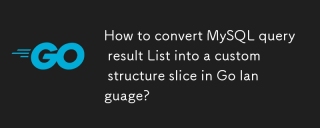
This article details efficient conversion of MySQL query results into Go struct slices. It emphasizes using database/sql's Scan method for optimal performance, avoiding manual parsing. Best practices for struct field mapping using db tags and robus
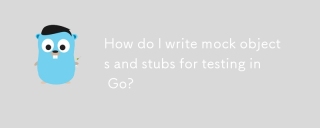
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
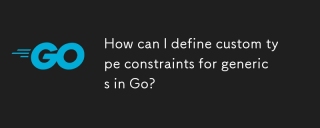
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices

This article details efficient file writing in Go, comparing os.WriteFile (suitable for small files) with os.OpenFile and buffered writes (optimal for large files). It emphasizes robust error handling, using defer, and checking for specific errors.
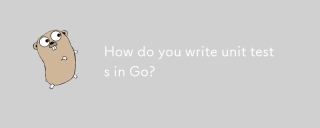
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
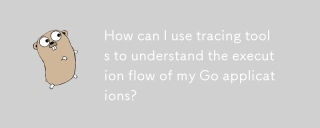
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
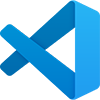
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
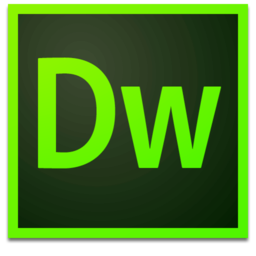
Dreamweaver Mac version
Visual web development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

Notepad++7.3.1
Easy-to-use and free code editor
