PHP Domain-Driven Design (DDD): Basic concepts and application
Domain-Driven Design (DDD) is a software development approach that focuses on understanding the business domain and modeling it effectively in code. In PHP, DDD can be applied to create more robust and maintainable applications by aligning the software closely with the business logic and processes. Here are the key aspects and their applications in PHP:
What are the key principles of Domain-Driven Design in PHP?
The key principles of Domain-Driven Design in PHP include:
- Ubiquitous Language: This principle emphasizes the importance of using a common language across all team members, including developers, domain experts, and stakeholders. In PHP, this means defining and using domain-specific terminology in your code, comments, and documentation to ensure consistency and clarity.
- Bounded Contexts: Bounded contexts help define boundaries within which a particular model is valid. In PHP, this can be implemented by organizing your code into different modules or namespaces that correspond to different bounded contexts, ensuring that each part of the application has a clear and focused purpose.
-
Entities and Value Objects: Entities are objects defined by their identity, while value objects are defined by their attributes. In PHP, you can implement these using classes. For example, a
User
class could be an entity identified by a uniqueid
, whereas aMoney
class could be a value object defined byamount
andcurrency
. - Aggregates: Aggregates are clusters of associated objects that are treated as a single unit. In PHP, this could be implemented using a root entity that manages the lifecycle of other entities and value objects within the aggregate.
- Domain Events: Domain events represent important occurrences within the domain. In PHP, you can implement event-driven architecture using events and listeners, allowing parts of your application to react to changes in the domain state.
- Repositories: Repositories abstract the storage and retrieval of aggregates. In PHP, you can create repository classes that handle database operations, ensuring that the domain layer remains independent of the data access layer.
- Services: Services encapsulate business logic that does not naturally fit within an entity or value object. In PHP, you can implement services as classes that operate on entities, value objects, and repositories.
How can DDD improve the structure and maintainability of PHP applications?
DDD can significantly improve the structure and maintainability of PHP applications in several ways:
- Improved Alignment with Business Needs: By focusing on the domain, DDD ensures that the software aligns closely with the business processes and requirements. This alignment makes the application more intuitive and easier to maintain, as changes in the business can be more easily reflected in the code.
- Modularity and Separation of Concerns: DDD promotes a modular architecture through the use of bounded contexts and aggregates. In PHP, this can lead to cleaner, more organized codebases that are easier to navigate and maintain. Each module or component is responsible for a specific part of the domain, reducing the complexity of the overall system.
- Enhanced Code Reusability: With clear definitions of entities, value objects, and services, DDD encourages the creation of reusable components. In PHP, this means that common business logic can be encapsulated in classes that can be used across different parts of the application, reducing duplication and improving maintainability.
- Better Collaboration: The use of ubiquitous language fosters better communication among team members. In PHP development, this can lead to more effective collaboration between developers, domain experts, and stakeholders, resulting in a more robust and maintainable application.
- Easier Testing: DDD's focus on the domain allows for more straightforward unit testing and integration testing. In PHP, this means you can write tests that closely align with the business rules, ensuring that the application behaves as expected and making it easier to identify and fix issues.
What are some practical steps to implement DDD in a PHP project?
Implementing Domain-Driven Design in a PHP project involves several practical steps:
-
Identify and Define Bounded Contexts: Start by mapping out the different areas of the business domain and their boundaries. In PHP, you can organize these contexts into separate namespaces or modules. For example, you might have
OrderManagement
,UserManagement
, andPaymentProcessing
as separate contexts. - Establish Ubiquitous Language: Work with domain experts to define and document the terminology used within the domain. Use this language consistently in your PHP code, comments, and documentation to ensure that everyone is on the same page.
-
Model Entities, Value Objects, and Aggregates: Identify the key entities, value objects, and aggregates within each bounded context. Implement these as classes in PHP. For example, in the
OrderManagement
context, you might have anOrder
entity (aggregate root),OrderItem
entities, and aMoney
value object. -
Implement Repositories: Create repository classes to handle the storage and retrieval of aggregates. In PHP, these repositories should encapsulate the database operations, allowing the domain layer to remain independent of the data access layer. For instance, you could have an
OrderRepository
that managesOrder
aggregates. -
Develop Domain Services: Identify any business logic that does not fit naturally within an entity or value object and implement it as domain services. In PHP, these services can be classes that operate on entities, value objects, and repositories. For example, you might have an
OrderService
that handles the business logic related to order processing. -
Implement Domain Events: Use events to notify different parts of the application about significant domain occurrences. In PHP, you can implement an event-driven architecture using events and listeners. For example, you might raise an
OrderPlaced
event when a new order is created, allowing other parts of the application to react accordingly. - Iterate and Refine: As you develop and refine your application, continue to iterate on your domain model. Work closely with domain experts to ensure that the model accurately reflects the business domain and adjust your PHP code accordingly.
By following these steps, you can effectively implement Domain-Driven Design in your PHP projects, leading to more maintainable and business-aligned software.
The above is the detailed content of PHP Domain-Driven Design (DDD): Basic concepts and application.. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 English version
Recommended: Win version, supports code prompts!

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
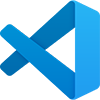
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
