Creating a tool to generate impressive front-end effects is a fantastic way to learn, hone your skills, and potentially gain recognition, regardless of your front-end experience level. Many popular online generators exist, such as Sarah Drasner's Hero Generator and CSS Grid Generator, Themesburg's Glassmorphism CSS Generator, and Components AI's extensive collection, including their Text Shadows generator.
This article demonstrates building an Animated Background Gradient Generator.
Project Setup with Next.js
This project utilizes Next.js for its ease of use. Begin by creating a new Next.js app:
npx create-next-app animated-gradient-background-generator
Modify pages/index.js
to serve as the application's shell:
import Head from "next/head"; import { SettingsProvider } from "../context/SettingsContext"; import Controls from "../components/Controls"; import Output from "../components/Output"; export default function Home() { return ( <title>Animated CSS Gradient Background Generator</title> <meta content="A tool for creating animated background gradients in pure CSS." name="description"> <link href="/favicon.ico" rel="icon"> <settingsprovider> <main style="{{" padding: textalign:> <h1 id="Animated-CSS-Gradient-Background-Generator">Animated CSS Gradient Background Generator</h1> <controls></controls> <output></output> </main> </settingsprovider> > ); }
Animated Gradients in CSS
The foundation for the generator is based on an example by Manuel Pinto, featuring animated CSS gradient backgrounds. The core CSS is:
body { background: linear-gradient(-45deg, #ee7752, #e73c7e, #23a6d5, #23d5ab); background-size: 400% 400%; animation: gradient 15s ease infinite; } @keyframes gradient { 0% { background-position: 0% 50%; } 50% { background-position: 100% 50%; } 100% { background-position: 0% 50%; } }
React Components for Gradient Generation
The generator will allow users to configure:
- An array of gradient colors
- Gradient angle
- Animation speed
The context/SettingsContext.js
file manages these settings using a higher-order component and default values:
import React, { useState, createContext } from "react"; const SettingsContext = createContext({ colorSelection: [] }); const SettingsProvider = ({ children }) => { const [colorSelection, setColorSelection] = useState([ "deepskyblue", "darkviolet", "blue", ]); const [angle, setAngle] = useState(300); const [speed, setSpeed] = useState(5); return ( <settingscontext.provider value="{{" colorselection setcolorselection angle setangle speed setspeed> {children} </settingscontext.provider> ); }; export { SettingsContext, SettingsProvider };
The application will consist of:
- Controls: Adjusts settings.
- AnimatedBackground: Displays the generated gradient.
- Output: Shows the generated CSS code.
The components/Controls.js
file (partially shown, full implementation requires Colors.js
and AddColor.js
components detailed below):
import React from "react"; import Colors from "./Colors"; import AddColor from "./AddColor"; import AngleRange from "./AngleRange"; import SpeedRange from "./SpeedRange"; import Random from "./Random"; const Controls = (props) => ( <div> <colors></colors> <addcolor></addcolor> <anglerange></anglerange> <speedrange></speedrange> <random></random> </div> ); export default Controls;
The components/Colors.js
component (handling color selection and deletion):
import React, { useContext } from "react"; import { SettingsContext } from "../context/SettingsContext"; const Colors = () => { const { colorSelection, setColorSelection } = useContext(SettingsContext); const onDelete = (deleteColor) => { setColorSelection(colorSelection.filter((color) => color !== deleteColor)); }; return ( <div> {colorSelection.map((color) => ( <div key="{color}" style="{{" display: width: height: marginright: marginbottom: background: color borderradius: position:> <button onclick="{()"> onDelete(color)} style={{ background: "crimson", color: "white", borderRadius: "50%", position: "absolute", top: "-8px", right: "-8px", border: "none", fontSize: "18px", lineHeight: 1, width: "24px", height: "24px", cursor: "pointer", }} > × </button> </div> ))} </div> ); }; export default Colors;
The components/AddColor.js
component (using react-color
for color picking):
// ... (import statements) const AddColor = () => { const [displayPicker, setDisplayPicker] = useState(false); const { colorSelection, setColorSelection } = useContext(SettingsContext); const handleColorChange = (color) => { setColorSelection([...colorSelection, color.hex]); setDisplayPicker(false); }; return ( <div> <button onclick="{()"> setDisplayPicker(!displayPicker)}> Add Color </button> {displayPicker && ( <chromepicker color="#fff" onchangecomplete="{handleColorChange}"></chromepicker> )} </div> ); }; export default AddColor;
The components/AngleRange.js
and components/SpeedRange.js
components (handling angle and speed adjustments):
// AngleRange.js import React, { useContext } from "react"; import { SettingsContext } from "../context/SettingsContext"; const AngleRange = () => { const { angle, setAngle } = useContext(SettingsContext); return ( <div style="{{" marginbottom:> <label htmlfor="angle">Angle:</label> <input type="range" id="angle" min="0" max="360" value="{angle}" onchange="{(e)"> setAngle(parseInt(e.target.value, 10))} /> <span>{angle} degrees</span> </div> ); }; export default AngleRange; // SpeedRange.js (similar structure, using setSpeed instead of setAngle)
The components/Random.js
component (for generating random settings):
import React, { useContext } from "react"; import { SettingsContext } from "../context/SettingsContext"; const Random = () => { const { setColorSelection, setAngle, setSpeed } = useContext(SettingsContext); const generateRandomColor = () => { return "#" Math.floor(Math.random() * 16777215).toString(16); }; const generateRandomSettings = () => { const numColors = 3 Math.floor(Math.random() * 3); const colors = Array.from({ length: numColors }, generateRandomColor); setColorSelection(colors); setAngle(Math.floor(Math.random() * 360)); setSpeed(Math.floor(Math.random() * 10) 1); }; return ( <button onclick="{generateRandomSettings}">Generate Random Settings</button> ); }; export default Random;
The components/AnimatedBackground.js
component (applies the generated styles):
import React, { useContext } from "react"; import { SettingsContext } from "../context/SettingsContext"; const AnimatedBackground = ({ children }) => { const { colorSelection, speed, angle } = useContext(SettingsContext); const background = `linear-gradient(${angle}deg, ${colorSelection.join( ", " )})`; const backgroundSize = `${colorSelection.length * 60}% ${ colorSelection.length * 60 }%`; const animation = `gradient-animation ${ colorSelection.length * Math.abs(speed - 11) }s ease infinite`; return ( <div style="{{" background backgroundsize animation minheight:> {children} </div> ); }; export default AnimatedBackground;
The components/Output.js
component (displays and allows copying the generated CSS):
import React, { useContext, useState } from "react"; import { SettingsContext } from "../context/SettingsContext"; const Output = () => { const [copied, setCopied] = useState(false); const { colorSelection, speed, angle } = useContext(SettingsContext); const generateCSS = () => { const background = `linear-gradient(${angle}deg, ${colorSelection.join( ", " )})`; const backgroundSize = `${colorSelection.length * 60}% ${ colorSelection.length * 60 }%`; const animation = `gradient-animation ${ colorSelection.length * Math.abs(speed - 11) }s ease infinite`; return ` .gradient-background { background: ${background}; background-size: ${backgroundSize}; animation: ${animation}; } @keyframes gradient-animation { 0% { background-position: 0% 50%; } 50% { background-position: 100% 50%; } 100% { background-position: 0% 50%; } } `; }; const handleCopy = () => { navigator.clipboard.writeText(generateCSS()); setCopied(true); setTimeout(() => setCopied(false), 2000); // Reset after 2 seconds }; return ( <div style="{{" margintop:> <pre class="brush:php;toolbar:false">{generateCSS()}
Remember to add the gradient-animation
keyframes to your global CSS (e.g., styles/globals.css
). Finally, wrap your main content within the AnimatedBackground
component in pages/index.js
. Don't forget to install react-color
: npm install react-color
. This detailed breakdown provides a more robust and user-friendly generator. Remember to adjust styling as needed for optimal presentation.
The above is the detailed content of Building a Cool Front End Thing Generator. For more information, please follow other related articles on the PHP Chinese website!
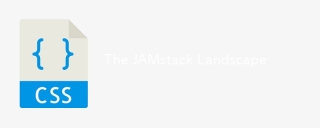
It's no big secret that Netlify invented the term JAMstack. While it's possible to embrace the JAMstack without using Netlify, it's notable that Netlify is at

I recently did an AMA over on DEV. Just taking the opportunity to port over some answers here like a good indiewebber.

Gatsby and WordPress is an interesting combo to watch. On one hand, it makes perfect sense. Gatsby can suck up data from anywhere, and with WordPress having a

This seemingly simple task had me scratching my head for a few hours while I was working on my website. As it turns out, getting the current page URL in
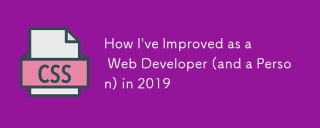
We’re sliding into the roaring twenties of the twenty-first century (cue Jazz music ?). It’s important that you and I, as responsible people, follow


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
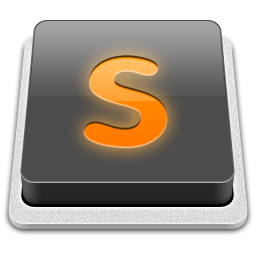
SublimeText3 Mac version
God-level code editing software (SublimeText3)
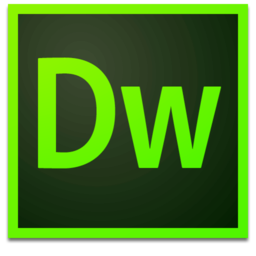
Dreamweaver Mac version
Visual web development tools