What are methods in Go?
In Go, a method is a function with a special receiver argument. The receiver appears in its own argument list between the func
keyword and the method name. Methods are associated with a type and can be used to perform operations on values of that type. This concept is similar to object-oriented programming where methods are essentially functions that belong to a class or an object. However, Go does not have classes, so methods are attached directly to types.
Methods allow you to define behaviors that can be performed on instances of a type. For example, you might define a method to update a field of a struct or to perform some calculation based on the fields of the struct. This makes your code more readable and organized, as related functionality is grouped together with the data it operates on.
How do you define a method in Go?
To define a method in Go, you use the following syntax:
func (receiver ReceiverType) MethodName(parameters) returnType { // Method body }
Here's a breakdown of the components:
-
func
is the keyword to start a function or method declaration. -
(receiver ReceiverType)
specifies the receiver.ReceiverType
can be a struct or any other type, andreceiver
is the name you give to the receiver parameter within the method. -
MethodName
is the name of the method. -
parameters
are the parameters the method takes, similar to a function. -
returnType
is the type of the value returned by the method, if any.
For example, if you have a Person
struct and want to define a method to set the person's age, you could do it like this:
type Person struct { Name string Age int } func (p *Person) SetAge(newAge int) { p.Age = newAge }
In this example, SetAge
is a method on the Person
type, and it modifies the Age
field of the Person
instance.
What is the difference between a method and a function in Go?
The primary difference between a method and a function in Go is that a method has a receiver argument, while a function does not. This receiver argument allows the method to be associated with a specific type, which makes the method callable on instances of that type.
Here are the key differences:
- Receiver Argument: A method has a receiver, which binds it to a type. A function does not have this feature.
-
Usage: Methods are called on instances of the type they are associated with, using the dot notation (e.g.,
person.SetAge(30)
). Functions are called directly (e.g.,SetAge(person, 30)
). - Organization: Methods group related behavior with the data type they operate on, which can make your code more readable and maintainable. Functions are standalone and can be used in a more modular way.
-
Syntax: The syntax for calling a method (
instance.Method()
) is different from calling a function (Function(instance)
).
Can methods in Go be associated with any type?
In Go, methods can be associated with almost any type, but there are some restrictions:
-
Basic Types: You can define methods on basic types like
int
,float64
,string
, etc., but you need to define a new type based on these basic types first. For example:type MyInt int func (m MyInt) Double() int { return int(m * 2) }
-
Structs: Methods can be directly associated with structs, which is the most common use case.
type Rectangle struct { width, height float64 } func (r Rectangle) Area() float64 { return r.width * r.height }
-
Arrays and Slices: You cannot directly associate methods with arrays or slices, but you can create a type that wraps an array or slice and then associate methods with that type.
type IntSlice []int func (s IntSlice) Sum() int { sum := 0 for _, v := range s { sum = v } return sum }
-
Pointers: You can define methods on pointer types, which is often useful for modifying the state of the receiver.
type Counter int func (c *Counter) Increment() { *c }
- Interfaces: Methods cannot be directly associated with interfaces, but interfaces can be used to define a set of methods that a type must implement.
In summary, while you can associate methods with a wide range of types in Go, you typically need to define a new type if you want to use methods with basic types or arrays/slices.
The above is the detailed content of What are methods in Go?. For more information, please follow other related articles on the PHP Chinese website!
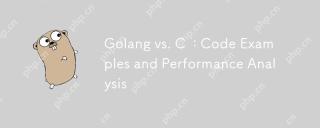
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.
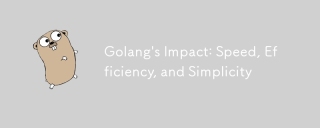
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
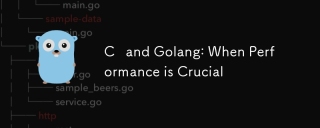
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
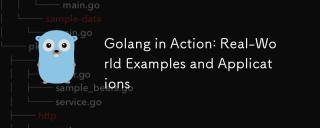
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
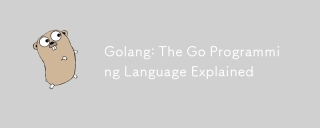
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
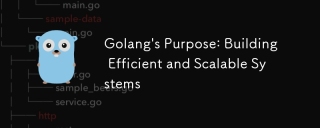
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
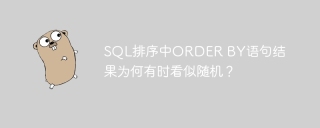
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
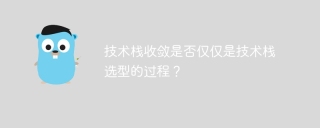
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
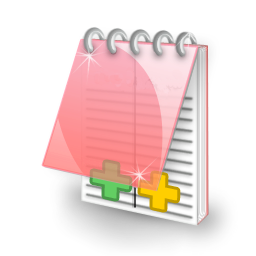
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
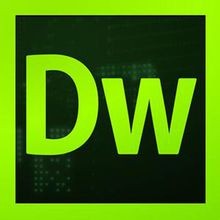
Dreamweaver CS6
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.