What is the empty interface (interface{}) in Go?
The empty interface in Go, denoted as interface{}
, is a special type of interface that contains no methods. Because it defines no methods, any value in Go satisfies the empty interface. This means that a variable of type interface{}
can hold values of any type, including built-in types like int
, string
, and float64
, as well as user-defined types such as structs and pointers.
Here's a simple example to illustrate:
var i interface{} i = 42 fmt.Println(i) // prints 42 i = "hello" fmt.Println(i) // prints hello
In this example, i
is declared as an interface{}
, and it can be assigned values of different types, showcasing its ability to hold any value.
What are the common use cases for the empty interface in Go programming?
The empty interface is commonly used in Go programming for several purposes:
-
General-purpose containers: When you need a slice or map to hold values of different types, the empty interface can be used. For example, in the standard library, the
fmt.Printf
function usesinterface{}
to accept values of any type for formatting.values := []interface{}{"hello", 42, true} for _, v := range values { fmt.Println(v) }
-
Function arguments and return types: When a function needs to handle arbitrary data types, the empty interface can be used as a parameter or return type. This is often seen in generic libraries or when writing callback functions.
func DoSomething(v interface{}) { fmt.Printf("Received value of type: %T\n", v) }
-
Polymorphism: Although Go does not support traditional object-oriented polymorphism, the empty interface can be used to implement something similar. Libraries like
encoding/json
use it to marshal and unmarshal data of any type.type Any interface{} var data Any json.Unmarshal([]byte(`{"name":"John", "age":30}`), &data)
How does the empty interface facilitate dynamic typing in Go?
Go is a statically typed language, but the empty interface enables a form of dynamic typing. Here’s how:
-
Type Assertion and Type Switch: With
interface{}
, you can use type assertions and type switches to determine the underlying type at runtime and extract its value. This gives you the flexibility to work with different types without knowing them at compile time.var i interface{} = "hello" // Type assertion s, ok := i.(string) if ok { fmt.Println(s) // prints hello } // Type switch switch v := i.(type) { case string: fmt.Println("It's a string:", v) case int: fmt.Println("It's an int:", v) default: fmt.Println("Unknown type") }
-
Reflection: The
reflect
package in Go can be used with the empty interface to inspect and manipulate values dynamically. This is useful for writing generic code that can operate on any data type.import "reflect" func Inspect(v interface{}) { val := reflect.ValueOf(v) fmt.Println("Type:", val.Type()) fmt.Println("Kind:", val.Kind()) fmt.Println("Value:", val.Interface()) }
By using these mechanisms, the empty interface allows Go programs to achieve dynamic typing behavior, which is otherwise not available in a statically typed language.
What are the potential drawbacks of using the empty interface in Go?
While the empty interface is powerful and flexible, it also comes with several potential drawbacks:
-
Loss of Type Safety: Using
interface{}
means you lose the type safety that Go usually enforces at compile time. Errors that might have been caught at compile time are deferred until runtime, which can lead to bugs that are harder to trace and fix. - Performance Overhead: When you use the empty interface, Go needs to perform runtime type checks and conversions, which can introduce performance overhead. This is especially true when using reflection, which can be significantly slower than native operations.
- Code Complexity: Overuse of the empty interface can lead to more complex and harder-to-understand code. Without explicit type information, it can be challenging for other developers (or even yourself after some time) to understand what types of data are expected and how they should be handled.
- Misuse for Generics: Before Go 1.18 introduced generics, the empty interface was often used as a workaround for generic programming. However, it’s not a substitute for true generics and can lead to less efficient and less maintainable code. With the advent of generics, it’s generally better to use them instead of relying on the empty interface for generic purposes.
In summary, while the empty interface is a powerful tool in Go, it should be used judiciously and with an understanding of its trade-offs.
The above is the detailed content of What is the empty interface (interface{}) in Go?. For more information, please follow other related articles on the PHP Chinese website!
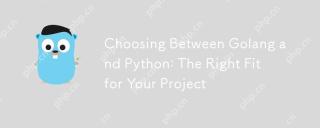
Golangisidealforperformance-criticalapplicationsandconcurrentprogramming,whilePythonexcelsindatascience,rapidprototyping,andversatility.1)Forhigh-performanceneeds,chooseGolangduetoitsefficiencyandconcurrencyfeatures.2)Fordata-drivenprojects,Pythonisp
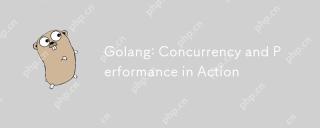
Golang achieves efficient concurrency through goroutine and channel: 1.goroutine is a lightweight thread, started with the go keyword; 2.channel is used for secure communication between goroutines to avoid race conditions; 3. The usage example shows basic and advanced usage; 4. Common errors include deadlocks and data competition, which can be detected by gorun-race; 5. Performance optimization suggests reducing the use of channel, reasonably setting the number of goroutines, and using sync.Pool to manage memory.
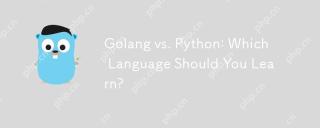
Golang is more suitable for system programming and high concurrency applications, while Python is more suitable for data science and rapid development. 1) Golang is developed by Google, statically typing, emphasizing simplicity and efficiency, and is suitable for high concurrency scenarios. 2) Python is created by Guidovan Rossum, dynamically typed, concise syntax, wide application, suitable for beginners and data processing.
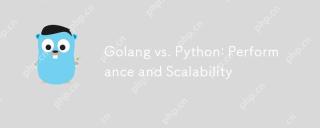
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
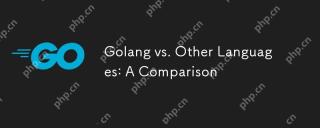
Go language has unique advantages in concurrent programming, performance, learning curve, etc.: 1. Concurrent programming is realized through goroutine and channel, which is lightweight and efficient. 2. The compilation speed is fast and the operation performance is close to that of C language. 3. The grammar is concise, the learning curve is smooth, and the ecosystem is rich.
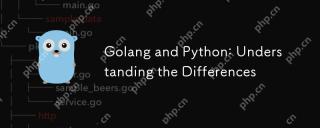
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
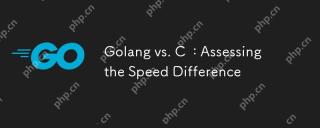
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
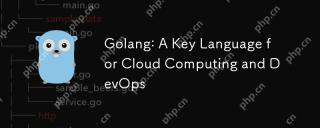
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
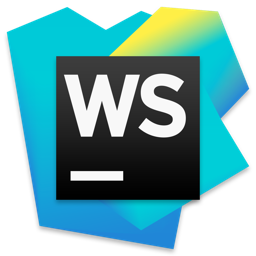
WebStorm Mac version
Useful JavaScript development tools
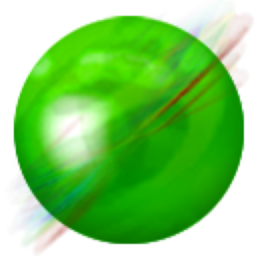
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.