What are React Hooks? Why were they introduced?
React Hooks are functions that allow developers to use state and lifecycle features in functional components. Introduced in React 16.8, hooks represent a shift towards writing more concise and reusable code in React applications. The primary reason for introducing hooks was to solve the problem of code reuse across components, particularly in the context of functional components.
Prior to hooks, developers relied heavily on class components for managing state and side effects. However, class components came with several drawbacks, such as verbose syntax, difficulty in understanding lifecycle methods, and challenges in code reuse. Functional components, on the other hand, were simpler and easier to understand, but they lacked the ability to manage state and side effects.
React Hooks were introduced to:
-
Allow State and Lifecycle in Functional Components: Hooks like
useState
anduseEffect
let functional components manage state and handle side effects, thereby removing the need for class components. - Simplify Code: Hooks simplify the component logic by breaking it down into smaller, manageable functions.
- Enable Code Reuse: Custom hooks allow developers to extract component logic into reusable functions.
- Reduce Confusion: They eliminate complex lifecycle methods, making the code easier to understand and debug.
How do React Hooks enhance functional components?
React Hooks significantly enhance functional components in several ways:
-
State Management: With the
useState
hook, functional components can now manage local state without converting to a class. This makes state management straightforward and keeps components simple and readable. -
Side Effects Handling: The
useEffect
hook allows functional components to handle side effects such as data fetching, subscriptions, or manually changing the DOM. This unifies the handling of side effects in a single place, improving readability and maintainability. -
Context Usage: The
useContext
hook simplifies accessing React context within functional components. This makes it easier to pass data through the component tree without having to pass props down manually at every level. -
Performance Optimization: Hooks like
useMemo
anduseCallback
provide performance optimizations by memoizing expensive computations or callbacks, preventing unnecessary re-renders. - Code Organization and Reusability: By using custom hooks, developers can encapsulate complex logic into reusable functions, leading to cleaner and more maintainable code.
-
Testing: Functional components using hooks are generally easier to test than class components due to their simpler nature and the absence of
this
binding issues.
What problems do React Hooks solve in state management?
React Hooks address several problems in state management:
-
Complexity of Class Components: Before hooks, state management in React required using class components, which introduced complexity due to
this
binding and lifecycle methods. Hooks allow state management in functional components, which are more intuitive and less error-prone. - Code Duplication: Managing state often required duplicating logic across multiple components. Custom hooks enable developers to reuse stateful logic without changing the component hierarchy, thus reducing code duplication.
-
Lack of Composability: With class components, composing reusable stateful logic was difficult. Hooks like
useReducer
anduseState
make it easy to compose and manage state in a more modular way. - Difficulty in Understanding State Flow: Hooks make it easier to understand how state is being used and updated within components, as the logic is more centralized and straightforward compared to the fragmented nature of lifecycle methods in class components.
-
Performance Issues: Hooks like
useMemo
anduseCallback
can help manage state more efficiently by preventing unnecessary re-renders, thus optimizing application performance.
Which React Hook is most commonly used for side effects?
The React Hook most commonly used for side effects is useEffect
. The useEffect
hook allows developers to perform side effects in function components, such as fetching data, setting up subscriptions, or manually changing the DOM.
useEffect
can be used to run code after rendering, and it can be configured to run only when certain values have changed, or just once after the initial render. This flexibility makes it a powerful tool for managing side effects in React applications.
Here is a basic example of how useEffect
is used:
import React, { useState, useEffect } from 'react'; function ExampleComponent() { const [data, setData] = useState(null); useEffect(() => { // This effect runs after every render fetchData().then(result => setData(result)); }, []); // Empty dependency array means this effect runs once on mount return ( <div> {data ? <p>Data: {data}</p> : <p>Loading...</p>} </div> ); } async function fetchData() { // Simulate an API call return new Promise(resolve => setTimeout(() => resolve('Some data'), 1000)); }
In this example, useEffect
is used to fetch data when the component mounts, demonstrating its utility in handling side effects.
The above is the detailed content of What are React Hooks? Why were they introduced?. For more information, please follow other related articles on the PHP Chinese website!

To integrate React into HTML, follow these steps: 1. Introduce React and ReactDOM in HTML files. 2. Define a React component. 3. Render the component into HTML elements using ReactDOM. Through these steps, static HTML pages can be transformed into dynamic, interactive experiences.

React’s popularity includes its performance optimization, component reuse and a rich ecosystem. 1. Performance optimization achieves efficient updates through virtual DOM and diffing mechanisms. 2. Component Reuse Reduces duplicate code by reusable components. 3. Rich ecosystem and one-way data flow enhance the development experience.

React is the tool of choice for building dynamic and interactive user interfaces. 1) Componentization and JSX make UI splitting and reusing simple. 2) State management is implemented through the useState hook to trigger UI updates. 3) The event processing mechanism responds to user interaction and improves user experience.

React is a front-end framework for building user interfaces; a back-end framework is used to build server-side applications. React provides componentized and efficient UI updates, and the backend framework provides a complete backend service solution. When choosing a technology stack, project requirements, team skills, and scalability should be considered.

The relationship between HTML and React is the core of front-end development, and they jointly build the user interface of modern web applications. 1) HTML defines the content structure and semantics, and React builds a dynamic interface through componentization. 2) React components use JSX syntax to embed HTML to achieve intelligent rendering. 3) Component life cycle manages HTML rendering and updates dynamically according to state and attributes. 4) Use components to optimize HTML structure and improve maintainability. 5) Performance optimization includes avoiding unnecessary rendering, using key attributes, and keeping the component single responsibility.

React is the preferred tool for building interactive front-end experiences. 1) React simplifies UI development through componentization and virtual DOM. 2) Components are divided into function components and class components. Function components are simpler and class components provide more life cycle methods. 3) The working principle of React relies on virtual DOM and reconciliation algorithm to improve performance. 4) State management uses useState or this.state, and life cycle methods such as componentDidMount are used for specific logic. 5) Basic usage includes creating components and managing state, and advanced usage involves custom hooks and performance optimization. 6) Common errors include improper status updates and performance issues, debugging skills include using ReactDevTools and Excellent

React is a JavaScript library for building user interfaces, with its core components and state management. 1) Simplify UI development through componentization and state management. 2) The working principle includes reconciliation and rendering, and optimization can be implemented through React.memo and useMemo. 3) The basic usage is to create and render components, and the advanced usage includes using Hooks and ContextAPI. 4) Common errors such as improper status update, you can use ReactDevTools to debug. 5) Performance optimization includes using React.memo, virtualization lists and CodeSplitting, and keeping code readable and maintainable is best practice.

React combines JSX and HTML to improve user experience. 1) JSX embeds HTML to make development more intuitive. 2) The virtual DOM mechanism optimizes performance and reduces DOM operations. 3) Component-based management UI to improve maintainability. 4) State management and event processing enhance interactivity.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
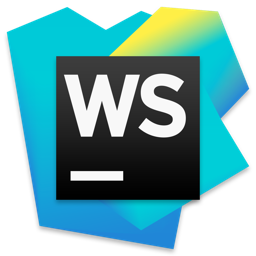
WebStorm Mac version
Useful JavaScript development tools
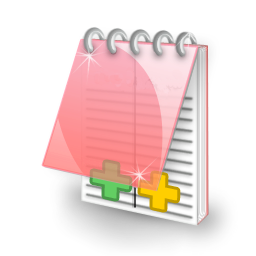
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
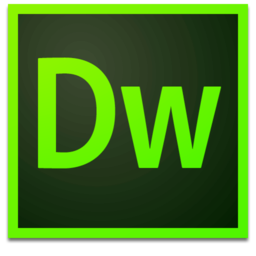
Dreamweaver Mac version
Visual web development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.