


How do you create a stored procedure in MySQL using the CREATE PROCEDURE statement?
How do you create a stored procedure in MySQL using the CREATE PROCEDURE statement?
To create a stored procedure in MySQL, you use the CREATE PROCEDURE
statement. Here's a step-by-step guide on how to do this:
-
Start the CREATE PROCEDURE statement: Begin with
CREATE PROCEDURE
followed by the name of your procedure. -
Define parameters (if any): If your procedure requires input parameters, define them within parentheses immediately after the procedure name. Parameters can have modes like
IN
,OUT
, orINOUT
, and each parameter needs a data type. -
Define the procedure body: The procedure body is enclosed in a
BEGIN
andEND
block. Within this block, you write the SQL statements that the procedure will execute. -
Terminate statements: By default, MySQL uses the semicolon (
;
) as a statement terminator. However, when creating stored procedures, you need to temporarily change this delimiter to avoid conflicts with the semicolons used in the procedure body. Typically,//
or$$
are used as alternative delimiters.
Here's an example of how to create a simple stored procedure:
DELIMITER // CREATE PROCEDURE GetEmployeeData( IN employeeID INT ) BEGIN SELECT * FROM employees WHERE id = employeeID; END // DELIMITER ;
In this example, GetEmployeeData
is a procedure that takes an employeeID
as an input parameter and returns all the data for that employee from the employees
table.
What parameters can be included in a MySQL stored procedure?
MySQL stored procedures can include parameters of various types and modes. The parameters can be categorized into three modes:
-
IN: This is the default mode. An
IN
parameter allows you to pass a value into the procedure. The procedure can read this value but cannot modify it. -
OUT: An
OUT
parameter allows the procedure to return a value to the calling environment. The procedure can modify the value of anOUT
parameter, and the modified value is accessible after the procedure execution. -
INOUT: An
INOUT
parameter combines the properties ofIN
andOUT
. It allows you to pass a value into the procedure and also allows the procedure to modify the value and return it to the calling environment.
Parameters can also have data types such as INT
, VARCHAR
, DATE
, etc., which should be specified when declaring them. Here's an example of a stored procedure with all three types of parameters:
DELIMITER // CREATE PROCEDURE ProcessEmployeeData( IN empID INT, OUT empName VARCHAR(100), INOUT empSalary DECIMAL(10, 2) ) BEGIN SELECT first_name, last_name INTO empName FROM employees WHERE id = empID; UPDATE employees SET salary = empSalary 1000 WHERE id = empID; SELECT salary INTO empSalary FROM employees WHERE id = empID; END // DELIMITER ;
How can you modify an existing stored procedure in MySQL?
To modify an existing stored procedure in MySQL, you use the ALTER PROCEDURE
statement. However, ALTER PROCEDURE
is limited to changing certain attributes of the procedure, such as its characteristics (like security context or SQL security). To modify the actual code or logic within the procedure, you need to use the DROP PROCEDURE
and CREATE PROCEDURE
statements.
Here’s how to do it:
- Drop the existing procedure:
DROP PROCEDURE IF EXISTS ProcedureName;
- Recreate the procedure with the new definition:
DELIMITER // CREATE PROCEDURE ProcedureName( -- Parameters ) BEGIN -- New procedure body END // DELIMITER ;
For example, if you want to update the GetEmployeeData
procedure to return only the first and last names of an employee, you would do the following:
DROP PROCEDURE IF EXISTS GetEmployeeData; DELIMITER // CREATE PROCEDURE GetEmployeeData( IN employeeID INT ) BEGIN SELECT first_name, last_name FROM employees WHERE id = employeeID; END // DELIMITER ;
What are the benefits of using stored procedures in MySQL for database management?
Stored procedures offer several benefits for database management in MySQL:
- Improved Performance: Stored procedures are compiled and stored in the database, which can lead to faster execution compared to executing individual SQL statements. They can also reduce network traffic by performing multiple operations within a single call.
- Code Reusability: Stored procedures can be reused across multiple applications and scripts, reducing code duplication and making maintenance easier.
- Enhanced Security: By encapsulating logic within a stored procedure, you can control access to the underlying tables and data. Users can be granted execute permissions on the procedure without having direct access to the tables it operates on.
- Simplified Maintenance: Changes to business logic can be made in one place (the stored procedure) rather than in multiple application codebases. This centralization simplifies maintenance and ensures consistency.
- Transaction Control: Stored procedures can manage transactions more effectively, ensuring data integrity and consistency across multiple operations.
- Reduced Development Time: With stored procedures, developers can focus on higher-level application logic rather than writing complex SQL queries repeatedly.
- Portability: Stored procedures can be easily moved from one MySQL server to another, which is beneficial for environments where databases are frequently migrated or replicated.
By leveraging these benefits, stored procedures can significantly enhance the efficiency and security of database management in MySQL.
The above is the detailed content of How do you create a stored procedure in MySQL using the CREATE PROCEDURE statement?. For more information, please follow other related articles on the PHP Chinese website!
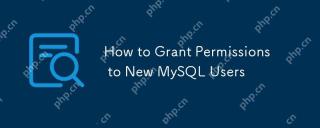
TograntpermissionstonewMySQLusers,followthesesteps:1)AccessMySQLasauserwithsufficientprivileges,2)CreateanewuserwiththeCREATEUSERcommand,3)UsetheGRANTcommandtospecifypermissionslikeSELECT,INSERT,UPDATE,orALLPRIVILEGESonspecificdatabasesortables,and4)
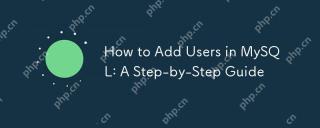
ToaddusersinMySQLeffectivelyandsecurely,followthesesteps:1)UsetheCREATEUSERstatementtoaddanewuser,specifyingthehostandastrongpassword.2)GrantnecessaryprivilegesusingtheGRANTstatement,adheringtotheprincipleofleastprivilege.3)Implementsecuritymeasuresl
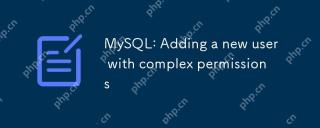
ToaddanewuserwithcomplexpermissionsinMySQL,followthesesteps:1)CreatetheuserwithCREATEUSER'newuser'@'localhost'IDENTIFIEDBY'password';.2)Grantreadaccesstoalltablesin'mydatabase'withGRANTSELECTONmydatabase.TO'newuser'@'localhost';.3)Grantwriteaccessto'
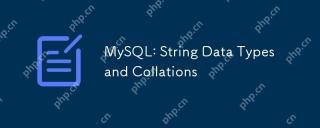
The string data types in MySQL include CHAR, VARCHAR, BINARY, VARBINARY, BLOB, and TEXT. The collations determine the comparison and sorting of strings. 1.CHAR is suitable for fixed-length strings, VARCHAR is suitable for variable-length strings. 2.BINARY and VARBINARY are used for binary data, and BLOB and TEXT are used for large object data. 3. Sorting rules such as utf8mb4_unicode_ci ignores upper and lower case and is suitable for user names; utf8mb4_bin is case sensitive and is suitable for fields that require precise comparison.
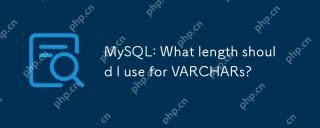
The best MySQLVARCHAR column length selection should be based on data analysis, consider future growth, evaluate performance impacts, and character set requirements. 1) Analyze the data to determine typical lengths; 2) Reserve future expansion space; 3) Pay attention to the impact of large lengths on performance; 4) Consider the impact of character sets on storage. Through these steps, the efficiency and scalability of the database can be optimized.
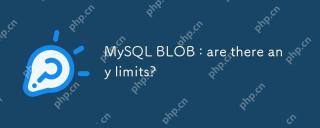
MySQLBLOBshavelimits:TINYBLOB(255bytes),BLOB(65,535bytes),MEDIUMBLOB(16,777,215bytes),andLONGBLOB(4,294,967,295bytes).TouseBLOBseffectively:1)ConsiderperformanceimpactsandstorelargeBLOBsexternally;2)Managebackupsandreplicationcarefully;3)Usepathsinst
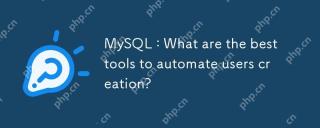
The best tools and technologies for automating the creation of users in MySQL include: 1. MySQLWorkbench, suitable for small to medium-sized environments, easy to use but high resource consumption; 2. Ansible, suitable for multi-server environments, simple but steep learning curve; 3. Custom Python scripts, flexible but need to ensure script security; 4. Puppet and Chef, suitable for large-scale environments, complex but scalable. Scale, learning curve and integration needs should be considered when choosing.
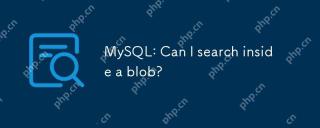
Yes,youcansearchinsideaBLOBinMySQLusingspecifictechniques.1)ConverttheBLOBtoaUTF-8stringwithCONVERTfunctionandsearchusingLIKE.2)ForcompressedBLOBs,useUNCOMPRESSbeforeconversion.3)Considerperformanceimpactsanddataencoding.4)Forcomplexdata,externalproc


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
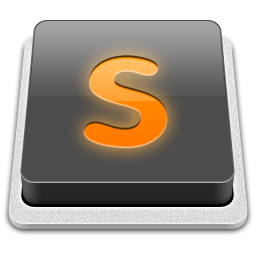
SublimeText3 Mac version
God-level code editing software (SublimeText3)

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor
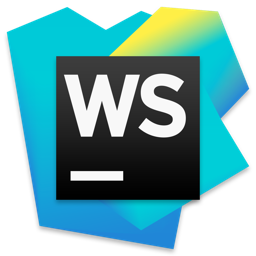
WebStorm Mac version
Useful JavaScript development tools
