What are buffered channels in Go?
Buffered channels in Go are a type of channel that can hold a limited number of values, unlike unbuffered channels, which can only hold one value at a time. When you create a buffered channel, you specify its buffer size as part of the channel declaration. For example, ch := make(chan int, 100)
creates a channel with a buffer size of 100 integers.
The key feature of buffered channels is that a send operation on a buffered channel will not block if the channel is not full. This means that the sender can proceed with its execution without waiting for a receiver to be ready, as long as there is space in the buffer. On the other hand, if the buffer is full, the sender will block until a receiver takes a value from the channel, freeing up space.
Buffered channels are particularly useful in scenarios where you want to decouple the sender and receiver processes to some extent, allowing the sender to continue its operations without immediately waiting for the receiver.
How do buffered channels improve the performance of Go programs?
Buffered channels can improve the performance of Go programs in several ways:
- Reduced Blocking: By allowing a certain number of values to be stored in the buffer, buffered channels can reduce the amount of time that senders and receivers spend waiting for each other. This can lead to more efficient use of CPU time and improved overall throughput.
- Better Resource Utilization: With buffered channels, the sender can continue processing and sending data without being blocked as frequently. This can lead to better utilization of system resources, especially in scenarios where the sender and receiver operate at different speeds.
- Smoother Data Flow: Buffered channels can help smooth out the flow of data between goroutines. For instance, if the producer generates data bursts and the consumer processes it at a steady pace, a buffered channel can help absorb these bursts without causing the producer to wait.
- Load Balancing: In scenarios where multiple goroutines are sending data to the same channel, a buffered channel can help balance the load by allowing some goroutines to continue while others wait for space to become available.
Overall, the use of buffered channels can lead to more responsive and efficient programs, especially in scenarios involving asynchronous communication and data streaming.
What are the key differences between buffered and unbuffered channels in Go?
The key differences between buffered and unbuffered channels in Go are as follows:
-
Buffer Size:
- Buffered Channels: Have a specified buffer size, allowing them to hold multiple values.
- Unbuffered Channels: Have no buffer size, meaning they can only hold one value at a time.
-
Blocking Behavior:
- Buffered Channels: A send operation blocks only if the channel's buffer is full. A receive operation blocks if the buffer is empty.
- Unbuffered Channels: Both send and receive operations block until the other operation is ready. This means a send operation will not complete until a corresponding receive operation is ready, and vice versa.
-
Synchronization:
- Buffered Channels: Provide a level of decoupling between senders and receivers because the sender does not need to wait for an immediate receiver, as long as the buffer is not full.
- Unbuffered Channels: Enforce strict synchronization because the sender must wait for a receiver before proceeding, ensuring that the sending and receiving goroutines are synchronized at the point of communication.
-
Use Cases:
- Buffered Channels: Are suitable for scenarios where the producer and consumer operate at different speeds or where you want to handle bursts of data without blocking the sender.
- Unbuffered Channels: Are ideal for scenarios where strict synchronization between goroutines is required, such as in scenarios where one goroutine needs to wait for another to complete an action before proceeding.
What scenarios are best suited for using buffered channels in Go?
Buffered channels are best suited for the following scenarios in Go:
- Asynchronous Processing: When you want to allow the sender to continue processing without being blocked by the receiver. For example, in a data processing pipeline where the producer generates data at a faster rate than the consumer can process it, a buffered channel can store the data until the consumer is ready.
- Handling Bursts of Data: If your program needs to handle bursts of data, such as in network applications or real-time data processing, buffered channels can help smooth out the data flow. The buffer absorbs the bursts, preventing the sender from being blocked.
- Decoupling Goroutines: When you want to decouple the execution of goroutines to some extent. Buffered channels can allow the sender to continue its work without immediate concern for whether the receiver is ready, as long as the buffer is not full.
- Load Balancing: In scenarios where multiple producers are sending data to the same channel, a buffered channel can help balance the load by allowing some producers to continue while others wait for space to become available in the buffer.
- Rate Limiting: Buffered channels can be used to implement simple rate limiting mechanisms. For example, you can control the rate at which requests are sent to a server by using a buffered channel with a limited size, ensuring that only a certain number of requests are in flight at any given time.
In summary, buffered channels in Go are particularly useful in scenarios where you need to manage the flow of data between goroutines in a way that allows for some level of asynchrony and buffering, improving overall system performance and responsiveness.
The above is the detailed content of What are buffered channels in Go?. For more information, please follow other related articles on the PHP Chinese website!
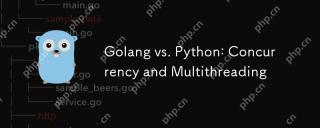
Golang is more suitable for high concurrency tasks, while Python has more advantages in flexibility. 1.Golang efficiently handles concurrency through goroutine and channel. 2. Python relies on threading and asyncio, which is affected by GIL, but provides multiple concurrency methods. The choice should be based on specific needs.
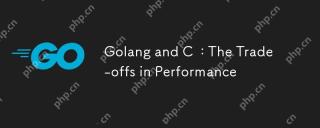
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
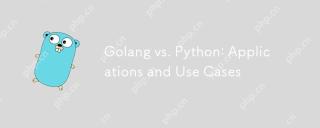
ChooseGolangforhighperformanceandconcurrency,idealforbackendservicesandnetworkprogramming;selectPythonforrapiddevelopment,datascience,andmachinelearningduetoitsversatilityandextensivelibraries.

Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
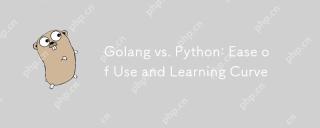
In what aspects are Golang and Python easier to use and have a smoother learning curve? Golang is more suitable for high concurrency and high performance needs, and the learning curve is relatively gentle for developers with C language background. Python is more suitable for data science and rapid prototyping, and the learning curve is very smooth for beginners.
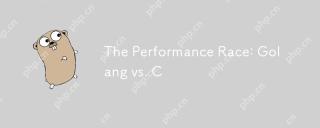
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
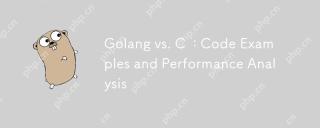
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.
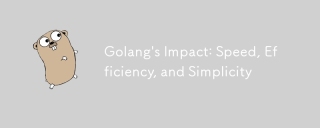
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
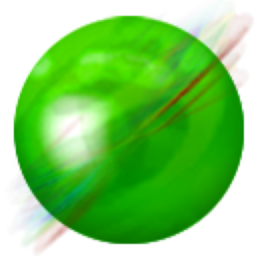
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 English version
Recommended: Win version, supports code prompts!
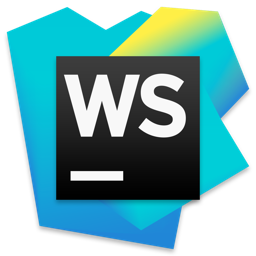
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version