How do you define and call functions in Go?
In Go, functions are defined using the func
keyword followed by the function name and a set of parentheses containing the parameter list. The body of the function is enclosed in curly braces {}
. Here is a basic example of a function definition:
func add(a int, b int) int { return a b }
In this example, add
is a function that takes two parameters of type int
and returns an int
.
To call a function in Go, you simply use the function name followed by the arguments in parentheses. For example:
result := add(3, 4) fmt.Println(result) // Output: 7
Here, add
function is called with arguments 3
and 4
, and the result is stored in the result
variable.
What are the best practices for naming functions in Go?
In Go, function naming follows certain conventions to maintain readability and consistency. Here are some best practices:
-
Use MixedCaps for Function Names: Go uses mixed caps (also known as camel case) for function names. The first letter of the function name is lowercase, and each subsequent word starts with an uppercase letter. For example,
calculateAverage
. -
Be Descriptive: Function names should clearly indicate their purpose. A function that calculates the average of a list of numbers might be named
calculateAverage
rather than something cryptic likecalcAvg
. -
Avoid Abbreviations: Unless the abbreviation is widely recognized and used within the Go community, it's better to use full words. For example,
calculate
is preferred overcalc
. -
Exported Functions: In Go, if a function name starts with an uppercase letter, it is exported and can be used by other packages. For example,
Add
is exported, whileadd
is not. - Consistency: Maintain consistency with the naming conventions used in the rest of the codebase or the standard library.
How can you pass arguments to functions in Go, and what are the differences between them?
In Go, you can pass arguments to functions using different methods, each with its own characteristics:
-
Value Parameters: The default way to pass arguments in Go is by value. When you pass an argument by value, a copy of the value is made and passed to the function. Changes to the parameter inside the function do not affect the original value outside the function.
func incrementByValue(x int) { x } a := 1 incrementByValue(a) fmt.Println(a) // Output: 1 (a remains unchanged)
-
Pointer Parameters: You can pass a pointer to a value. This allows the function to modify the original value.
func incrementByPointer(x *int) { (*x) } a := 1 incrementByPointer(&a) fmt.Println(a) // Output: 2 (a is modified)
-
Variadic Parameters: Go supports variadic functions, which can accept an indefinite number of arguments of the same type. The variadic parameter is denoted by
...
before the type.func sum(numbers ...int) int { total := 0 for _, num := range numbers { total = num } return total } fmt.Println(sum(1, 2, 3, 4)) // Output: 10
What is the significance of return values in Go functions, and how are they handled?
Return values in Go functions play a crucial role in allowing functions to communicate results back to the caller. Here are key points about return values in Go:
-
Single Return Value: A function can return a single value. The return type is specified after the parameter list.
func square(x int) int { return x * x }
-
Multiple Return Values: Go allows functions to return multiple values. This is useful for returning both a result and an error.
func divide(a, b float64) (float64, error) { if b == 0 { return 0, errors.New("division by zero") } return a / b, nil } result, err := divide(10, 2) if err != nil { fmt.Println(err) } else { fmt.Println(result) // Output: 5 }
-
Named Return Values: Go supports named return values, which can make the code more readable. Named return values are declared as part of the function signature.
func namedReturn(x int) (result int) { result = x * x return }
-
Bare Return: When using named return values, Go allows the use of a bare
return
statement, which returns the named return values.func namedReturnWithBareReturn(x int) (result int) { result = x * x return // equivalent to return result }
Return values are crucial for error handling, allowing functions to return both a result and an error status, which is a common pattern in Go programming.
The above is the detailed content of How do you define and call functions in Go?. For more information, please follow other related articles on the PHP Chinese website!
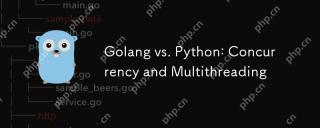
Golang is more suitable for high concurrency tasks, while Python has more advantages in flexibility. 1.Golang efficiently handles concurrency through goroutine and channel. 2. Python relies on threading and asyncio, which is affected by GIL, but provides multiple concurrency methods. The choice should be based on specific needs.
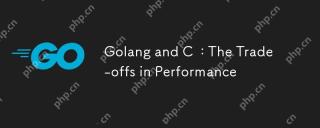
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
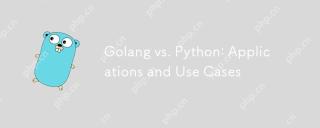
ChooseGolangforhighperformanceandconcurrency,idealforbackendservicesandnetworkprogramming;selectPythonforrapiddevelopment,datascience,andmachinelearningduetoitsversatilityandextensivelibraries.

Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
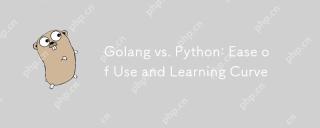
In what aspects are Golang and Python easier to use and have a smoother learning curve? Golang is more suitable for high concurrency and high performance needs, and the learning curve is relatively gentle for developers with C language background. Python is more suitable for data science and rapid prototyping, and the learning curve is very smooth for beginners.
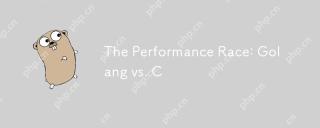
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
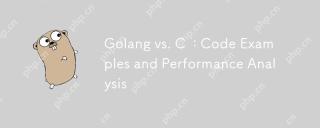
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.
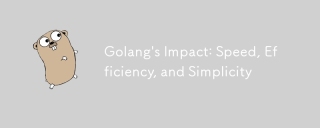
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
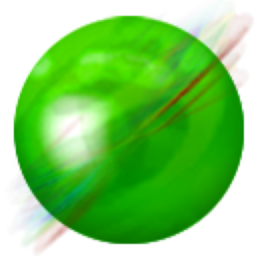
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Zend Studio 13.0.1
Powerful PHP integrated development environment
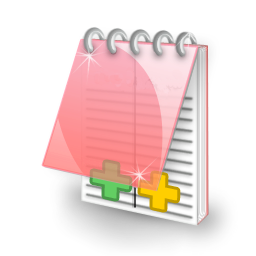
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
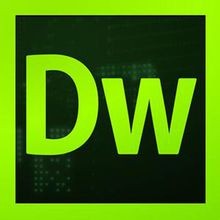
Dreamweaver CS6
Visual web development tools