How do you use panic and recover in Go?
In Go, panic
and recover
are used to handle runtime errors and exceptional conditions. Here’s a detailed explanation of how to use them:
-
Panic:
- The
panic
function is used to stop the normal execution of a function. When apanic
is triggered, the current function stops executing and returns control to its caller. If the caller doesn't handle the panic, the process continues up the call stack until either the panic is recovered or the program terminates. -
To invoke
panic
, you simply call thepanic
function with a value that provides information about the error. This value can be of any type, though it’s common to use a string or an error.func examplePanic() { panic("This is a panic!") }
- The
-
Recover:
- The
recover
function is used to regain control of a panicking goroutine. It is only effective during the execution of a deferred function. -
To use
recover
, you must first defer a function that callsrecover
. If a panic occurs within the scope of the function where the deferred function was declared, the deferred function will execute, andrecover
will return the value passed topanic
.func exampleRecover() { defer func() { if r := recover(); r != nil { fmt.Println("Recovered from panic:", r) } }() panic("This is a panic!") }
- The
What are the common use cases for panic and recover in Go?
The panic
and recover
mechanism in Go is typically used in the following scenarios:
-
Handling Unrecoverable Errors:
- When a function encounters an unrecoverable error that prevents it from continuing execution safely,
panic
can be used. For example, if a required configuration file is missing or corrupted, apanic
might be appropriate.
- When a function encounters an unrecoverable error that prevents it from continuing execution safely,
-
Error Propagation in Complex Functions:
- In deeply nested function calls,
panic
can be a straightforward way to propagate errors up the call stack, especially if conventional error handling becomes cumbersome.
- In deeply nested function calls,
-
Library or Framework Design:
- Some libraries and frameworks use
panic
andrecover
to handle unexpected conditions internally, ensuring that they can recover gracefully and potentially report the error to the user.
- Some libraries and frameworks use
-
Testing and Debugging:
- During development and testing,
panic
can be used to quickly identify and debug issues by stopping execution at the point of failure.
- During development and testing,
-
Ensuring Critical Operations:
- In scenarios where certain operations must succeed (e.g., closing a file),
panic
can be used within a deferred function to ensure these operations are completed even if the main execution path encounters an error.
- In scenarios where certain operations must succeed (e.g., closing a file),
How does the panic and recover mechanism affect Go program performance?
The use of panic
and recover
can have several performance implications on a Go program:
-
Stack Unwinding:
- When a
panic
is triggered, the Go runtime unwinds the stack until it finds a function with a deferred function that can recover from the panic. This process can be costly in terms of CPU cycles and memory usage, particularly if the call stack is deep.
- When a
-
Program Termination:
- If a
panic
is not recovered, it will cause the program to terminate. This can lead to resource leaks and other undesirable behaviors, affecting the overall performance and reliability of the program.
- If a
-
Deferred Function Execution:
- Deferred functions are executed even in the presence of a
panic
, which can introduce additional overhead. However, this is also a mechanism that allowsrecover
to work effectively.
- Deferred functions are executed even in the presence of a
-
Debugging and Logging:
-
panic
andrecover
can be used for logging and debugging purposes. While this aids in development and maintenance, excessive use can slow down execution due to the additional processing required for logging.
-
-
Resource Management:
- Proper use of
panic
andrecover
can ensure that resources are managed correctly (e.g., closing files or connections), which can prevent performance degradation due to resource leaks.
- Proper use of
What are the best practices for using panic and recover in Go?
Here are some best practices for using panic
and recover
in Go:
-
Use Panic Sparingly:
- Reserve
panic
for truly exceptional circumstances where the program cannot recover and continue safely. Regular error handling should be used for expected errors.
- Reserve
-
Always Recover:
- If you use
panic
, ensure there is a recovery mechanism in place to handle it. This prevents unnecessary program termination and allows for graceful handling of the error.
- If you use
-
Defer Functions for Recovery:
- Always use deferred functions to set up recovery points. This ensures that
recover
is called in the correct context and can catch any panics within the function.
- Always use deferred functions to set up recovery points. This ensures that
-
Provide Meaningful Error Information:
- When calling
panic
, pass a meaningful error message or value that helps in debugging and understanding the cause of the panic.
- When calling
-
Avoid Panic in Libraries:
- Libraries should generally avoid using
panic
directly, as the decision to handle panics should be left to the application using the library. Instead, libraries should return errors that the calling code can handle appropriately.
- Libraries should generally avoid using
-
Test Panic and Recovery:
- Ensure that your testing covers scenarios where
panic
is triggered andrecover
is used. This helps in verifying that your error handling mechanisms work as expected.
- Ensure that your testing covers scenarios where
-
Document Panic Usage:
- Clearly document where and why
panic
is used within your code. This helps other developers understand the intent and expected behavior of the code.
- Clearly document where and why
By following these best practices, you can effectively leverage panic
and recover
in Go to handle exceptional cases while maintaining the robustness and reliability of your programs.
The above is the detailed content of How do you use panic and recover in Go?. For more information, please follow other related articles on the PHP Chinese website!
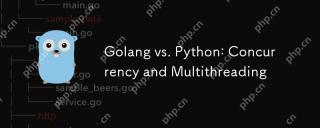
Golang is more suitable for high concurrency tasks, while Python has more advantages in flexibility. 1.Golang efficiently handles concurrency through goroutine and channel. 2. Python relies on threading and asyncio, which is affected by GIL, but provides multiple concurrency methods. The choice should be based on specific needs.
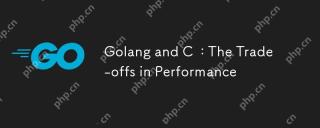
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
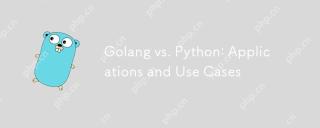
ChooseGolangforhighperformanceandconcurrency,idealforbackendservicesandnetworkprogramming;selectPythonforrapiddevelopment,datascience,andmachinelearningduetoitsversatilityandextensivelibraries.

Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
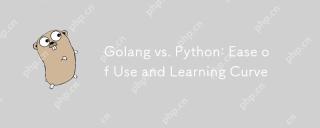
In what aspects are Golang and Python easier to use and have a smoother learning curve? Golang is more suitable for high concurrency and high performance needs, and the learning curve is relatively gentle for developers with C language background. Python is more suitable for data science and rapid prototyping, and the learning curve is very smooth for beginners.
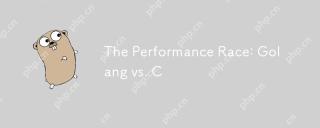
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
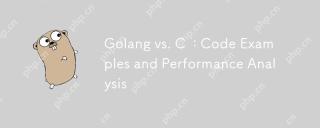
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.
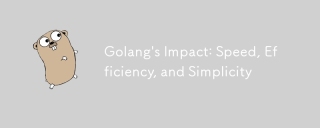
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
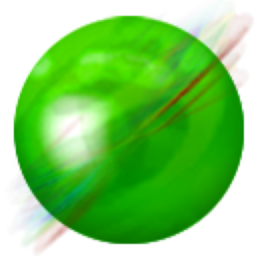
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Zend Studio 13.0.1
Powerful PHP integrated development environment
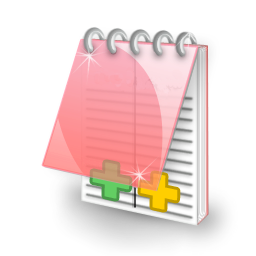
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
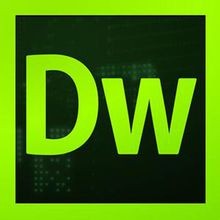
Dreamweaver CS6
Visual web development tools