How do you group data using the GROUP BY clause?
The GROUP BY clause in SQL is used to group rows that have the same values in specified columns into summary rows. It is commonly used with aggregate functions (such as COUNT, MAX, MIN, SUM, AVG) to perform calculations on each group of data. Here's how you can use the GROUP BY clause:
-
Basic Syntax: The basic syntax of a GROUP BY query is:
SELECT column1, aggregate_function(column2) FROM table_name GROUP BY column1;
In this example,
column1
is used to group the data, andaggregate_function(column2)
is applied to each group. -
Example: Suppose you have a table called
sales
with columnsregion
andamount
. You want to find the total sales amount for each region. The query would be:SELECT region, SUM(amount) as total_sales FROM sales GROUP BY region;
This query groups the data by the
region
column and calculates the sum ofamount
for each group. -
Multiple Columns: You can group by multiple columns by listing them in the GROUP BY clause, separated by commas. For example:
SELECT region, product, SUM(amount) as total_sales FROM sales GROUP BY region, product;
This query will group the data by both
region
andproduct
, and calculate the sum ofamount
for each unique combination ofregion
andproduct
.
What are the common functions used with GROUP BY to aggregate data?
When using the GROUP BY clause, several common aggregate functions are used to perform calculations on the grouped data. These functions include:
-
COUNT(): Counts the number of rows in each group. For example:
SELECT region, COUNT(*) as number_of_sales FROM sales GROUP BY region;
-
SUM(): Calculates the total of a numeric column in each group. For example:
SELECT region, SUM(amount) as total_sales FROM sales GROUP BY region;
-
AVG(): Calculates the average of a numeric column in each group. For example:
SELECT region, AVG(amount) as average_sale FROM sales GROUP BY region;
-
MAX(): Finds the maximum value of a column in each group. For example:
SELECT region, MAX(amount) as max_sale FROM sales GROUP BY region;
-
MIN(): Finds the minimum value of a column in each group. For example:
SELECT region, MIN(amount) as min_sale FROM sales GROUP BY region;
These functions allow you to summarize data in various ways, providing insights into the grouped data.
How can GROUP BY be combined with HAVING to filter grouped results?
The HAVING clause is used in conjunction with the GROUP BY clause to filter grouped results based on a condition. Unlike the WHERE clause, which filters rows before grouping, the HAVING clause filters groups after they have been created. Here's how you can use them together:
-
Basic Syntax: The syntax of a query combining GROUP BY and HAVING is:
SELECT column1, aggregate_function(column2) FROM table_name GROUP BY column1 HAVING condition;
-
Example: Suppose you want to find regions with total sales greater than $10,000 from the
sales
table. The query would be:SELECT region, SUM(amount) as total_sales FROM sales GROUP BY region HAVING SUM(amount) > 10000;
This query first groups the data by
region
, calculates the total sales for each region, and then filters the results to include only those regions where the total sales exceed $10,000. -
Combining with WHERE: You can also use WHERE to filter rows before grouping and HAVING to filter groups. For example:
SELECT region, SUM(amount) as total_sales FROM sales WHERE amount > 0 GROUP BY region HAVING SUM(amount) > 10000;
In this query, the WHERE clause first filters out any rows with a negative or zero amount, the data is then grouped by
region
, and the HAVING clause filters the groups based on the total sales.
What are the potential pitfalls to avoid when using GROUP BY in SQL queries?
When using the GROUP BY clause in SQL queries, there are several potential pitfalls to avoid:
-
Incorrect Column References: In a query with GROUP BY, any column in the SELECT list that is not an aggregate function must be included in the GROUP BY clause. Forgetting to include a non-aggregated column in the GROUP BY clause can result in an error or unexpected results. For example:
SELECT region, product, SUM(amount) -- This will cause an error if 'product' is not included in GROUP BY FROM sales GROUP BY region;
The correct version would be:
SELECT region, product, SUM(amount) FROM sales GROUP BY region, product;
-
Mixing Aggregate and Non-Aggregate Columns: Mixing aggregate and non-aggregate columns in the SELECT list without proper grouping can lead to unexpected results. For instance:
SELECT region, SUM(amount), amount -- This will cause an error because 'amount' is not aggregated FROM sales GROUP BY region;
To fix this, you need to either group by
amount
or use an aggregate function on it. -
Using HAVING Without GROUP BY: The HAVING clause is meant to be used with GROUP BY. Using HAVING without GROUP BY will result in an error in many SQL databases. For example:
SELECT region, SUM(amount) FROM sales HAVING SUM(amount) > 10000; -- This will cause an error because GROUP BY is missing
The correct version would be:
SELECT region, SUM(amount) FROM sales GROUP BY region HAVING SUM(amount) > 10000;
- Performance Issues with Large Datasets: GROUP BY operations can be computationally expensive, especially with large datasets. Poorly optimized queries can lead to performance issues. To mitigate this, consider using appropriate indexing and avoiding unnecessary columns in the GROUP BY clause.
- Order of Operations: Remember that the order of operations in SQL is WHERE, GROUP BY, HAVING, and then SELECT. Misunderstanding this order can lead to incorrect results. For example, the WHERE clause filters rows before grouping, while HAVING filters groups after grouping.
By being aware of these pitfalls, you can write more effective and efficient GROUP BY queries.
The above is the detailed content of How do you group data using the GROUP BY clause?. For more information, please follow other related articles on the PHP Chinese website!
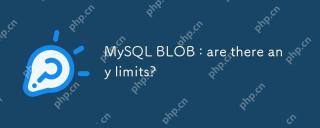
MySQLBLOBshavelimits:TINYBLOB(255bytes),BLOB(65,535bytes),MEDIUMBLOB(16,777,215bytes),andLONGBLOB(4,294,967,295bytes).TouseBLOBseffectively:1)ConsiderperformanceimpactsandstorelargeBLOBsexternally;2)Managebackupsandreplicationcarefully;3)Usepathsinst
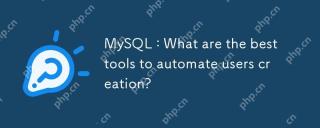
The best tools and technologies for automating the creation of users in MySQL include: 1. MySQLWorkbench, suitable for small to medium-sized environments, easy to use but high resource consumption; 2. Ansible, suitable for multi-server environments, simple but steep learning curve; 3. Custom Python scripts, flexible but need to ensure script security; 4. Puppet and Chef, suitable for large-scale environments, complex but scalable. Scale, learning curve and integration needs should be considered when choosing.
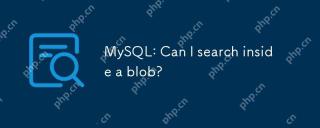
Yes,youcansearchinsideaBLOBinMySQLusingspecifictechniques.1)ConverttheBLOBtoaUTF-8stringwithCONVERTfunctionandsearchusingLIKE.2)ForcompressedBLOBs,useUNCOMPRESSbeforeconversion.3)Considerperformanceimpactsanddataencoding.4)Forcomplexdata,externalproc
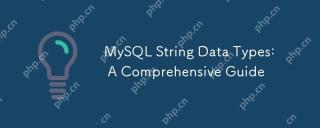
MySQLoffersvariousstringdatatypes:1)CHARforfixed-lengthstrings,idealforconsistentlengthdatalikecountrycodes;2)VARCHARforvariable-lengthstrings,suitableforfieldslikenames;3)TEXTtypesforlargertext,goodforblogpostsbutcanimpactperformance;4)BINARYandVARB
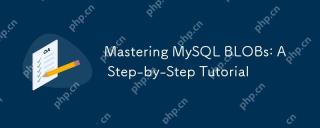
TomasterMySQLBLOBs,followthesesteps:1)ChoosetheappropriateBLOBtype(TINYBLOB,BLOB,MEDIUMBLOB,LONGBLOB)basedondatasize.2)InsertdatausingLOAD_FILEforefficiency.3)Storefilereferencesinsteadoffilestoimproveperformance.4)UseDUMPFILEtoretrieveandsaveBLOBsco
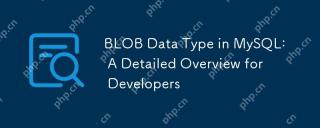
BlobdatatypesinmysqlareusedforvoringLargebinarydatalikeImagesoraudio.1) Useblobtypes (tinyblobtolongblob) Basedondatasizeneeds. 2) Storeblobsin Perplate Petooptimize Performance.3) ConsidersxterNal Storage Forel Blob Romana DatabasesizerIndimprovebackupupe
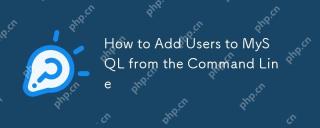
ToadduserstoMySQLfromthecommandline,loginasroot,thenuseCREATEUSER'username'@'host'IDENTIFIEDBY'password';tocreateanewuser.GrantpermissionswithGRANTALLPRIVILEGESONdatabase.*TO'username'@'host';anduseFLUSHPRIVILEGES;toapplychanges.Alwaysusestrongpasswo
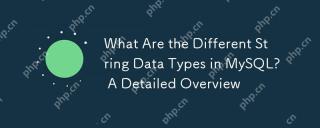
MySQLofferseightstringdatatypes:CHAR,VARCHAR,BINARY,VARBINARY,BLOB,TEXT,ENUM,andSET.1)CHARisfixed-length,idealforconsistentdatalikecountrycodes.2)VARCHARisvariable-length,efficientforvaryingdatalikenames.3)BINARYandVARBINARYstorebinarydata,similartoC


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
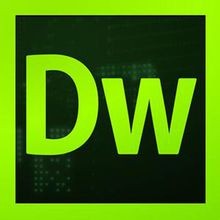
Dreamweaver CS6
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
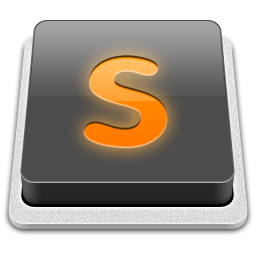
SublimeText3 Mac version
God-level code editing software (SublimeText3)
