How to Use Workerman for Building Real-Time Collaboration Tools?
Workerman is an open-source, high-performance PHP application server that is particularly suited for building real-time collaboration tools. To use Workerman for such applications, follow these steps:
-
Installation: First, you need to install Workerman. You can do this via Composer by running
composer require workerman/workerman
or by downloading the source code directly from the official GitHub repository. -
Setting Up a Basic Server: Create a PHP file, for example,
start.php
, and use the following code to set up a basic server:<?php use Workerman\Worker; $worker = new Worker('websocket://0.0.0.0:2346'); $worker->onMessage = function($connection, $data){ $connection->send('Hello ' . $data); }; Worker::runAll();
This sets up a WebSocket server that listens on port 2346 and responds to incoming messages.
-
Implementing Real-Time Features: For real-time collaboration tools, you would need to handle multiple user connections and manage their states. You can implement this by managing a list of connections and broadcasting messages to them:
<?php use Workerman\Worker; $worker = new Worker('websocket://0.0.0.0:2346'); $worker->connections = []; $worker->onConnect = function($connection) use ($worker) { $worker->connections[spl_object_hash($connection)] = $connection; }; $worker->onMessage = function($connection, $data) use ($worker) { foreach($worker->connections as $con) { $con->send($data); } }; $worker->onClose = function($connection) use ($worker) { unset($worker->connections[spl_object_hash($connection)]); }; Worker::runAll();
This code manages connections, broadcasts messages, and cleans up connections when they are closed.
- Testing and Deployment: Test your application using a WebSocket client like a browser's developer tools or a dedicated WebSocket client application. Once tested, you can deploy Workerman on a production server, ensuring you have the necessary configurations for scalability and security.
What are the key features of Workerman that enhance real-time collaboration?
Workerman offers several key features that enhance real-time collaboration:
- High Concurrency: Workerman is designed to handle a large number of concurrent connections efficiently, making it suitable for real-time applications with many users.
- Low Latency: It uses an event-driven, non-blocking I/O model, which minimizes latency and enhances the responsiveness of real-time collaboration tools.
- WebSocket Support: Workerman natively supports WebSockets, a key protocol for real-time communication, allowing for efficient, full-duplex communication between clients and servers.
- Scalability: With its support for clustering, Workerman can scale horizontally to manage increased traffic and user load, ensuring that the collaboration tool remains performant.
- Extensibility: Workerman supports various protocols and can be extended with custom protocols and functionalities, making it flexible for different types of real-time collaboration tools.
- Cross-Platform: It can run on different operating systems, which enhances its suitability for diverse deployment environments.
How can Workerman be integrated with existing systems for seamless real-time communication?
Integrating Workerman with existing systems for real-time communication can be achieved through the following steps:
- API Integration: Use Workerman's API to handle real-time communication while integrating it with your existing system's APIs. For instance, if you have a REST API, you can modify it to communicate with Workerman for real-time functionalities.
- Database Synchronization: Ensure that Workerman is connected to your existing database. Use triggers or scheduled jobs to synchronize data between the database and Workerman's real-time operations.
- Middleware: Implement middleware solutions to act as a bridge between Workerman and your existing system. Middleware can handle protocol translation, data transformation, and routing between Workerman and other components.
- Event-Driven Architecture: Design an event-driven architecture where Workerman listens to events from your existing system and responds accordingly. This can be done using message queues like RabbitMQ or Apache Kafka.
- Authentication and Authorization: Ensure that Workerman can use the same authentication and authorization mechanisms as your existing system, providing a seamless experience for users.
What are the best practices for optimizing Workerman's performance in large-scale collaborative environments?
To optimize Workerman's performance in large-scale collaborative environments, consider the following best practices:
- Load Balancing: Use load balancers to distribute traffic evenly across multiple Workerman instances. This helps in handling high concurrency and ensures no single server becomes a bottleneck.
- Horizontal Scaling: Scale horizontally by adding more Workerman instances. Ensure these instances can communicate with each other to maintain a coherent state across the system.
- Connection Pooling: Implement connection pooling to manage database or external service connections efficiently. This reduces the overhead of creating new connections for each request.
- Data Caching: Use caching mechanisms like Redis to store frequently accessed data. This reduces database load and improves response times for real-time operations.
- Optimized WebSocket Handling: Implement efficient WebSocket handling strategies, such as using binary data when possible and optimizing the sending of messages to reduce overhead.
- Monitoring and Logging: Implement comprehensive monitoring and logging to identify performance bottlenecks and optimize accordingly. Use tools like Prometheus and Grafana for real-time monitoring.
- Efficient Resource Management: Manage resources efficiently by setting appropriate worker numbers and thread counts based on your server's capacity and expected load.
By following these best practices, you can ensure that Workerman operates efficiently in large-scale collaborative environments, providing a smooth real-time experience for users.
The above is the detailed content of How to Use Workerman for Building Real-Time Collaboration Tools?. For more information, please follow other related articles on the PHP Chinese website!

Workerman's WebSocket client enhances real-time communication with features like asynchronous communication, high performance, scalability, and security, easily integrating with existing systems.

The article discusses using Workerman, a high-performance PHP server, to build real-time collaboration tools. It covers installation, server setup, real-time feature implementation, and integration with existing systems, emphasizing Workerman's key f
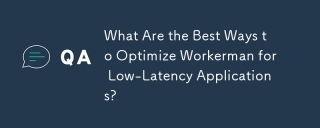
The article discusses optimizing Workerman for low-latency applications, focusing on asynchronous programming, network configuration, resource management, data transfer minimization, load balancing, and regular updates.
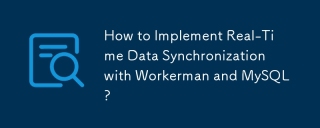
The article discusses implementing real-time data synchronization using Workerman and MySQL, focusing on setup, best practices, ensuring data consistency, and addressing common challenges.
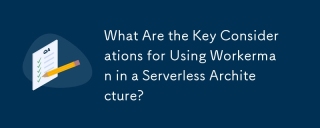
The article discusses integrating Workerman into serverless architectures, focusing on scalability, statelessness, cold starts, resource management, and integration complexity. Workerman enhances performance through high concurrency, reduced cold sta
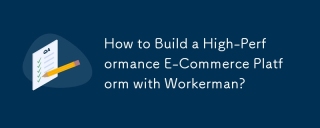
The article discusses building a high-performance e-commerce platform using Workerman, focusing on its features like WebSocket support and scalability to enhance real-time interactions and efficiency.
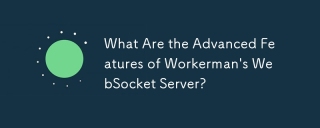
Workerman's WebSocket server enhances real-time communication with features like scalability, low latency, and security measures against common threats.
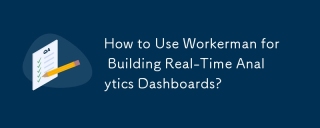
The article discusses using Workerman, a high-performance PHP server, to build real-time analytics dashboards. It covers installation, server setup, data processing, and frontend integration with frameworks like React, Vue.js, and Angular. Key featur


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
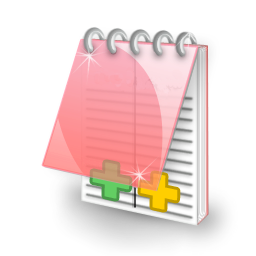
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Linux new version
SublimeText3 Linux latest version

SublimeText3 Chinese version
Chinese version, very easy to use