


What Are the Best Ways to Optimize Workerman for Low-Latency Applications?
To optimize Workerman for low-latency applications, several key strategies can be implemented:
- Use Asynchronous Programming: Workerman is built on an event-driven model, which is ideal for handling many concurrent connections with low latency. Ensure that your code uses non-blocking I/O operations to keep the event loop running efficiently.
- Optimize Network Configuration: Adjusting the network settings can significantly impact latency. For instance, enable TCP_NODELAY to disable Nagle's algorithm, which can introduce delays in sending small packets.
- Efficient Resource Management: Ensure that Workerman is configured to use the optimal number of worker processes. Too many workers can lead to increased overhead, while too few may not fully utilize system resources. Monitor CPU and memory usage to find the right balance.
- Minimize Data Transfer: Reduce the amount of data sent over the network by compressing data where possible and only sending necessary information. Use efficient serialization formats like Protocol Buffers or MessagePack.
- Use Load Balancing: Implement load balancing to distribute incoming connections across multiple Workerman instances. This helps in maintaining low latency by preventing any single instance from becoming a bottleneck.
- Regular Updates and Monitoring: Keep Workerman updated to the latest version to benefit from performance improvements and bug fixes. Use monitoring tools to track latency and adjust configurations as needed.
How can you configure Workerman settings to minimize latency in real-time applications?
To configure Workerman settings for minimizing latency in real-time applications, consider the following adjustments:
-
Increase Worker Count: Set the worker count appropriately based on your system's capabilities. For example, if you have a quad-core CPU, you might set the worker count to 4 or 8 to leverage hyper-threading. This can be done in the configuration file:
'count' => 4,
-
Adjust Connection and Timeout Settings: Tweak settings related to connection management and timeouts to optimize for real-time applications. For instance, set a shorter connection timeout to quickly release idle connections:
'max_package_size' => 1024000, // 1MB 'heartbeat_time' => 30, // Heartbeat interval in seconds 'heartbeat_expire_time' => 90, // Connection considered dead after no heartbeat in seconds
-
Enable TCP_NODELAY: As mentioned earlier, enabling TCP_NODELAY can reduce latency by avoiding Nagle's algorithm. This can be set in the Workerman configuration:
'tcp_nodelay' => true,
-
Configure Buffering: Adjust buffer sizes and settings to optimize data transfer. For example, you might set a smaller send buffer to reduce latency:
'send_buffer_size' => 65535, // Smaller send buffer
-
SSL/TLS Settings: If your application uses SSL/TLS, optimize the settings for faster handshakes and less overhead. Consider using session caching and tuning the cipher suite:
'ssl' => [ 'verify_peer' => false, 'verify_peer_name' => false, 'allow_self_signed' => true, 'ciphers' => 'ECDHE-ECDSA-AES128-GCM-SHA256:ECDHE-RSA-AES128-GCM-SHA256', ],
What are the most effective coding practices to enhance Workerman's performance for low-latency needs?
To enhance Workerman's performance for low-latency needs, adhere to the following coding practices:
- Use Asynchronous I/O: Leverage Workerman's event-driven architecture by using asynchronous I/O operations. Avoid blocking calls by using non-blocking functions for database queries, file operations, and network communications.
- Minimize CPU Usage: Optimize your code to reduce CPU-intensive operations. Use caching mechanisms to store frequently accessed data and avoid redundant calculations.
- Efficient Data Structures: Choose appropriate data structures that allow for fast access and manipulation. For instance, use hash tables for quick lookups and avoid unnecessary iterations over large datasets.
- Connection Pooling: Implement connection pooling for databases and other external services to reduce the overhead of creating new connections for each request.
- Code Optimization: Profile your code to identify bottlenecks and optimize those sections. Use efficient algorithms and keep the code modular to facilitate easier updates and optimizations.
- Error Handling: Implement efficient error handling to avoid unnecessary logging or excessive stack traces that can slow down the application. Use centralized error handling where feasible.
- Code Review and Testing: Regularly review and test your code to ensure it remains performant. Use automated testing tools to quickly identify and fix performance issues.
Are there specific tools or plugins that can help monitor and improve Workerman's latency?
Yes, several tools and plugins can help monitor and improve Workerman's latency:
-
Workerman's Built-in Monitoring: Workerman comes with built-in monitoring tools that can help track performance metrics, including latency. You can enable the statistics server to gather real-time data:
use Workerman\Worker; use Workerman\WebServer; // Statistics server $statistic_server = new Worker('Text://0.0.0.0:55656'); $statistic_server->count = 1; $statistic_server->name = 'StatisticServer'; // Web server for statistics $web = new WebServer('http://0.0.0.0:55858'); $web->count = 1; $web->addRoot('www.Statistics.com', __DIR__ . '/Web'); Worker::runAll();
- Prometheus and Grafana: Use Prometheus to collect metrics and Grafana to visualize them. You can expose Workerman metrics to Prometheus and set up dashboards in Grafana to monitor latency and other performance indicators.
- New Relic: This APM tool provides detailed performance monitoring and can help identify latency issues in Workerman applications. It offers real-time insights and can track application performance over time.
- Datadog: Datadog provides comprehensive monitoring and analytics for your Workerman applications. It can help track latency, throughput, and other metrics, and offers alerting features to notify you of performance issues.
-
Workerman Plugins: There are several plugins available for Workerman that can help with performance optimization:
- Workerman/Monitor: Provides monitoring capabilities for Workerman applications.
- Workerman/Autoloader: Helps in optimizing the autoloading of classes, which can indirectly improve performance.
By utilizing these tools and plugins, you can effectively monitor and improve Workerman's latency, ensuring your applications run smoothly and efficiently.
The above is the detailed content of What Are the Best Ways to Optimize Workerman for Low-Latency Applications?. For more information, please follow other related articles on the PHP Chinese website!

Workerman's WebSocket client enhances real-time communication with features like asynchronous communication, high performance, scalability, and security, easily integrating with existing systems.

The article discusses using Workerman, a high-performance PHP server, to build real-time collaboration tools. It covers installation, server setup, real-time feature implementation, and integration with existing systems, emphasizing Workerman's key f
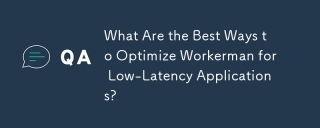
The article discusses optimizing Workerman for low-latency applications, focusing on asynchronous programming, network configuration, resource management, data transfer minimization, load balancing, and regular updates.
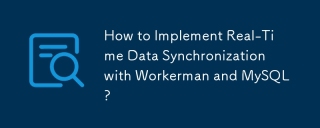
The article discusses implementing real-time data synchronization using Workerman and MySQL, focusing on setup, best practices, ensuring data consistency, and addressing common challenges.
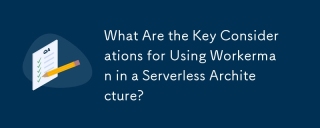
The article discusses integrating Workerman into serverless architectures, focusing on scalability, statelessness, cold starts, resource management, and integration complexity. Workerman enhances performance through high concurrency, reduced cold sta
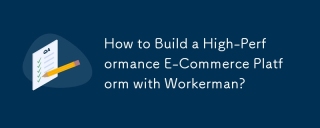
The article discusses building a high-performance e-commerce platform using Workerman, focusing on its features like WebSocket support and scalability to enhance real-time interactions and efficiency.
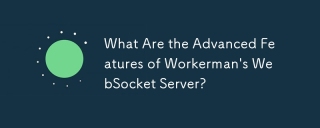
Workerman's WebSocket server enhances real-time communication with features like scalability, low latency, and security measures against common threats.
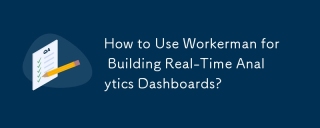
The article discusses using Workerman, a high-performance PHP server, to build real-time analytics dashboards. It covers installation, server setup, data processing, and frontend integration with frameworks like React, Vue.js, and Angular. Key featur


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
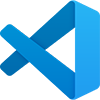
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
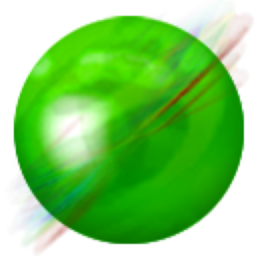
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
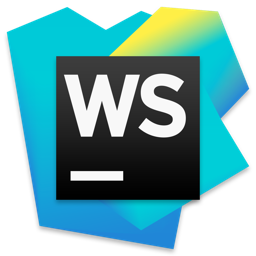
WebStorm Mac version
Useful JavaScript development tools