


How do I use Layui's layer module to create modal windows and dialog boxes?
To use Layui's layer module to create modal windows and dialog boxes, you first need to include the Layui library in your project. You can do this by downloading Layui from its official website and including the necessary CSS and JavaScript files in your HTML.
Once Layui is set up, you can create modal windows and dialog boxes using the layer.open
method. Here's a basic example of how to create a simple modal window:
<!DOCTYPE html> <html> <head> <title>Layui Modal Example</title> <link rel="stylesheet" href="path/to/layui/css/layui.css"> </head> <body> <button onclick="openModal()">Open Modal</button> <script src="path/to/layui/layui.js"></script> <script> layui.use('layer', function(){ var layer = layui.layer; function openModal() { layer.open({ type: 1, title: 'Modal Title', content: '<div style="padding: 20px;">This is a modal window.</div>', area: ['300px', '200px'] }); } }); </script> </body> </html>
In this example, layer.open
is called with an options object that specifies the type of layer (1 for an HTML layer), the title of the modal, the content, and the dimensions of the modal window.
What are the different types of dialog boxes I can create with Layui's layer module?
Layui's layer module provides several types of dialog boxes, each serving different purposes. Here are the main types:
-
Alert Dialog (
type: 0
):
Used to show a message to the user. It has a single "OK" button.layer.alert('This is an alert message.', {icon: 0});
-
Confirm Dialog (
type: 0
):
Used to ask the user for confirmation. It has "OK" and "Cancel" buttons.layer.confirm('Are you sure?', {icon: 3, title:'Confirm'}, function(index){ //do something layer.close(index); });
-
Prompt Dialog (
type: 0
):
Used to get input from the user. It includes an input field and "OK" and "Cancel" buttons.layer.prompt({title: 'Enter your name', formType: 2}, function(text, index){ layer.close(index); layer.msg('Your name is: ' text); });
-
Tab Dialog (
type: 1
):
Used to display content with tabs. It is an HTML layer that can contain multiple tabs.layer.tab({ area: ['600px', '300px'], tab: [{ title: 'Tab 1', content: 'Content of Tab 1' }, { title: 'Tab 2', content: 'Content of Tab 2' }] });
-
Page Dialog (
type: 2
):
Used to load an external page into a dialog.layer.open({ type: 2, title: 'External Page', content: 'external-page.html', area: ['300px', '200px'] });
-
Iframe Dialog (
type: 2
):
Similar to the Page Dialog, but it loads content into an iframe.layer.open({ type: 2, title: 'Iframe Content', content: 'https://example.com', area: ['300px', '200px'] });
How can I customize the appearance and behavior of modal windows using Layui's layer module?
Layui's layer module provides numerous options for customizing the appearance and behavior of modal windows. Here are some common ways to do so:
-
Size and Position:
You can control the size and position of the modal window usingarea
andoffset
options.layer.open({ type: 1, content: 'Custom Modal Content', area: ['500px', '300px'], // Width and Height offset: ['100px', '200px'] // Top and Left offset });
-
Title and Close Button:
You can customize the title and whether to show the close button.layer.open({ type: 1, title: ['Custom Title', 'background-color:#009688; color:#fff;'], // Title with custom styles content: 'Content', closeBtn: 0 // Hide close button });
-
Animation:
You can specify different animations for opening the modal using theanim
option.layer.open({ type: 1, content: 'Content', anim: 2 // Animation type (0-6) });
-
Buttons and Callbacks:
You can add custom buttons with callbacks to handle user interactions.layer.open({ type: 1, content: 'Content', btn: ['OK', 'Cancel'], yes: function(index, layero){ // OK button clicked layer.close(index); }, btn2: function(index, layero){ // Cancel button clicked layer.close(index); } });
-
Styles:
You can apply custom styles to the modal window using CSS..layui-layer-title { background-color: #333; color: #fff; } .layui-layer-content { background-color: #f0f0f0; }
What are some common pitfalls to avoid when using Layui's layer module for modal windows and dialog boxes?
When using Layui's layer module, it's important to avoid common pitfalls that can lead to issues. Here are some key points to consider:
-
Improper Closure:
Always make sure to close the layer properly to prevent memory leaks. Uselayer.close(index)
to close a specific layer.var index = layer.open({...}); layer.close(index);
-
Multiple Layers:
Be cautious when opening multiple layers at the same time, as it can confuse users. Ensure that previous layers are closed before opening new ones. -
Accessibility:
Ensure that modal windows are accessible. Provide keyboard navigation and ensure that the content is readable for screen readers. -
Performance:
Loading heavy content into modal windows can slow down your application. Consider usingtype: 2
for external pages to reduce the load on the main page. -
Responsive Design:
Ensure that your modal windows are responsive. Use percentage values forarea
to make them adapt to different screen sizes.layer.open({ area: ['80%', '60%'] });
-
Cross-Origin Issues:
When usingtype: 2
to load external pages or iframes, be aware of cross-origin issues. Make sure the external content is from the same domain or properly configured for CORS.
By being mindful of these potential pitfalls, you can use Layui's layer module more effectively and create better user experiences.
The above is the detailed content of How do I use Layui's layer module to create modal windows and dialog boxes?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
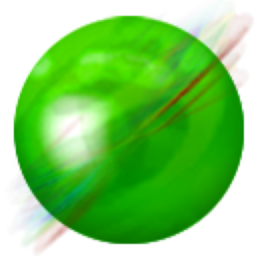
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
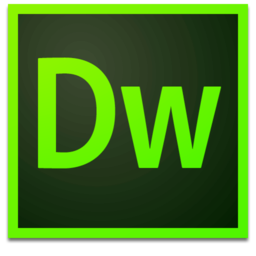
Dreamweaver Mac version
Visual web development tools
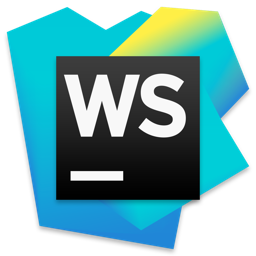
WebStorm Mac version
Useful JavaScript development tools
