How do I use uni-app's storage API (uni.setStorage, uni.getStorage)?
Uni-app provides a convenient way to store and retrieve data locally using its storage API, which includes uni.setStorage
for storing data and uni.getStorage
for retrieving it. Here's how to use these APIs:
-
Using
uni.setStorage
:- This method is used to store data in the local storage.
- The syntax is
uni.setStorage(Object object)
, where theobject
is a parameter with propertieskey
anddata
. -
Example:
uni.setStorage({ key: 'userInfo', data: { name: 'John Doe', age: 30 }, success: function () { console.log('Data stored successfully'); } });
- The
success
callback is optional and can be used to handle successful storage operations.
-
Using
uni.getStorage
:- This method is used to retrieve data from the local storage.
- The syntax is
uni.getStorage(Object object)
, where theobject
has akey
property and optionalsuccess
callback. -
Example:
uni.getStorage({ key: 'userInfo', success: function (res) { console.log('Data retrieved:', res.data); }, fail: function (res) { console.log('Failed to retrieve data:', res); } });
- The
success
andfail
callbacks are optional and can be used to handle the data retrieval result.
By following these examples, you can effectively store and retrieve data using uni-app's storage API.
What are the best practices for managing data with uni.setStorage and uni.getStorage?
When using uni.setStorage
and uni.getStorage
, adhering to best practices ensures efficient and secure data management:
-
Use Meaningful Keys:
- Choose clear and descriptive keys for your data. This makes it easier to manage and understand your data structures.
-
Avoid Storing Sensitive Data:
- Do not store sensitive information such as passwords or personal identification numbers directly in local storage. If necessary, use encryption.
-
Data Serialization:
- Ensure that the data you store is serialized properly, especially if it's a complex object. JSON.stringify() can be used to serialize data before storing.
-
Handle Asynchronous Nature:
- Both
setStorage
andgetStorage
are asynchronous. Use callbacks or promises to handle operations, ensuring your app behaves correctly while waiting for data operations to complete.
- Both
-
Error Handling:
- Implement error handling using the
fail
callbacks to gracefully manage cases where data operations fail.
- Implement error handling using the
-
Clean Up Unused Data:
- Regularly review and remove outdated or unnecessary data to keep the storage clean and efficient.
-
Size Limitations:
- Be aware of the storage limitations (typically around 5MB for most platforms) and manage your data accordingly, considering offloading to remote storage if necessary.
By following these best practices, you can effectively manage local data in your uni-app applications.
How can I troubleshoot common issues when using uni.getStorage to retrieve data?
Troubleshooting common issues when using uni.getStorage
can be straightforward if you follow these steps:
-
Check the Key:
- Ensure that the key you are using to retrieve the data matches exactly the key used to store it. Typos or incorrect keys are common issues.
-
Review Data Serialization:
- If you serialized data before storing it (e.g., with JSON.stringify()), ensure you deserialize it (e.g., with JSON.parse()) when retrieving it.
-
Verify Asynchronous Handling:
- Make sure you are correctly handling the asynchronous nature of
uni.getStorage
. Ensure callbacks or promises are used properly to handle the result.
- Make sure you are correctly handling the asynchronous nature of
-
Check for Errors:
- Use the
fail
callback to catch and log any errors that occur during the retrieval process. This can help identify issues like storage being full or corrupted data.
- Use the
-
Inspect Stored Data:
- Use platform-specific tools (e.g., browser dev tools for web, Xcode for iOS, Android Studio for Android) to manually inspect the stored data and verify its integrity.
-
Ensure Proper Permissions:
- On some platforms, you might need to request specific permissions to access local storage. Ensure these permissions are correctly set up.
-
Review Code Synchronization:
- If working in a team, ensure that all developers are using the same version of the code to avoid discrepancies in data storage and retrieval.
By carefully following these troubleshooting steps, you can effectively resolve common issues when using uni.getStorage
.
What are the limitations or considerations when using uni.setStorage for data storage?
When using uni.setStorage
for data storage, it's important to be aware of the following limitations and considerations:
-
Storage Size Limit:
- Most platforms have a storage limit of around 5MB. Exceeding this limit may result in storage failures or data loss.
-
Asynchronous Operations:
-
uni.setStorage
operations are asynchronous, which means you must handle the storage process using callbacks or promises. This can add complexity to your code.
-
-
Data Persistence:
- Data stored with
uni.setStorage
is generally persistent but can be cleared by the user or the system in certain scenarios (e.g., app data clearance, device reset).
- Data stored with
-
Security Concerns:
- Data stored in local storage is not inherently secure. It can potentially be accessed by other apps or through device rooting/jailbreaking. Sensitive data should be encrypted if stored locally.
-
Cross-Platform Compatibility:
- While
uni.setStorage
aims to provide a consistent API across platforms, slight differences in behavior might exist. It's essential to test thoroughly on all target platforms.
- While
-
Performance Considerations:
- Frequent storage operations can impact app performance. Consider batching operations or using alternative data management strategies for better performance.
-
Synchronous Alternatives:
- In some cases, you might need synchronous data storage.
uni.setStorageSync
anduni.getStorageSync
are available, but they can block the main thread and should be used cautiously.
- In some cases, you might need synchronous data storage.
-
Data Type Limitations:
- Only certain data types (typically stringifiable data) can be stored. Complex objects may need to be serialized before storage.
Understanding these limitations and considerations will help you use uni.setStorage
more effectively and make informed decisions about when to use local storage and when to seek alternative solutions.
The above is the detailed content of How do I use uni-app's storage API (uni.setStorage, uni.getStorage)?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
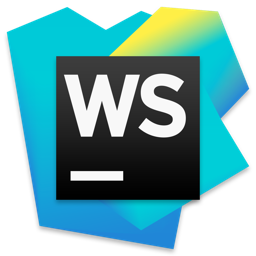
WebStorm Mac version
Useful JavaScript development tools

Notepad++7.3.1
Easy-to-use and free code editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Chinese version
Chinese version, very easy to use
