How do I use uni-app's animation API?
To use uni-app's animation API, you need to follow these steps:
-
Create an Animation Instance: Begin by creating an animation instance using
uni.createAnimation(options)
. Theoptions
parameter allows you to set default properties like duration, timing function, and delay.const animation = uni.createAnimation({ duration: 1000, timingFunction: 'ease', });
-
Define Animation Actions: Use the methods provided by the animation instance to define the actions you want to perform. Common methods include
translate()
,rotate()
,scale()
, andopacity()
. These actions modify the properties of the animation instance.animation.translate(30, 30).rotate(45).scale(2, 2).step();
-
Export the Animation Data: After defining the actions, you need to export the animation data to be used in your view. You can export the animation data by calling
export()
method of the animation instance.this.animationData = animation.export();
-
Apply the Animation to a View: Finally, apply the exported animation data to the view using the
animation
property in the view's style.<view :animation="animationData">Animated View</view>
What are the key functions in uni-app's animation API?
The key functions in uni-app's animation API include:
-
createAnimation(options): This function is used to create a new animation instance. The
options
object can include properties likeduration
,timingFunction
,delay
, andtransformOrigin
. -
translate(x, y): Moves the element by the specified
x
andy
values. - rotate(deg): Rotates the element by the specified degrees.
-
scale(sx, [sy]): Scales the element. The
sx
value scales the element horizontally, and the optionalsy
value scales it vertically. Ifsy
is not provided, it defaults to thesx
value. -
opacity(value): Sets the opacity of the element, where
value
is a number between 0 and 1. -
step(options): Marks the end of one set of actions and allows you to start a new set with different properties. The
options
parameter can override the default properties of the animation. - export(): Exports the current animation state so it can be applied to a view.
Can I combine multiple animations in uni-app?
Yes, you can combine multiple animations in uni-app using the step()
method. This method allows you to segment your animation into different steps, each with its own set of properties and timing.
Here is an example of how to combine multiple animations:
const animation = uni.createAnimation(); animation.translate(30, 30).step({ duration: 300 }); animation.rotate(45).step({ duration: 300 }); animation.scale(2, 2).step({ duration: 300 }); this.animationData = animation.export();
In this example, the element will first move 30 pixels to the right and 30 pixels down over 300 milliseconds, then rotate 45 degrees over the next 300 milliseconds, and finally scale to twice its size over another 300 milliseconds.
How do I control the timing of animations in uni-app?
To control the timing of animations in uni-app, you can use the following methods and properties:
-
Duration: Set the
duration
property when creating the animation instance or within thestep()
method to control how long each segment of the animation lasts.const animation = uni.createAnimation({ duration: 1000, // Default duration for all steps }); animation.translate(30, 30).step({ duration: 500 }); // Override duration for this step
-
Timing Function: Use the
timingFunction
property to control the speed curve of the animation. Options includelinear
,ease
,ease-in
,ease-out
, andease-in-out
.const animation = uni.createAnimation({ timingFunction: 'ease-in-out', });
-
Delay: Use the
delay
property to add a delay before the animation starts.const animation = uni.createAnimation({ delay: 500, // Delay the start of the animation by 500ms });
-
Step: Use the
step()
method to segment your animation into different steps, each with its own timing properties.animation.translate(30, 30).step({ duration: 300, timingFunction: 'ease-in' }); animation.rotate(45).step({ duration: 300, timingFunction: 'ease-out' });
By carefully setting these properties, you can precisely control the timing and flow of your animations in uni-app.
The above is the detailed content of How do I use uni-app's animation API?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
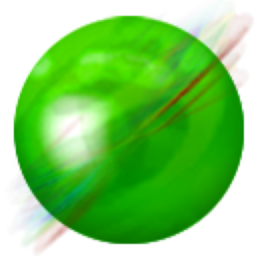
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Zend Studio 13.0.1
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor
