How do I use computed properties in uni-app?
In uni-app, computed properties are used to create properties that are derived from other data in your component. To use computed properties, you need to define them within the computed
field of your component options. Here's how you can do it:
-
Define the computed property: Inside the
computed
field, you define a function that returns the computed value. This function should not have any arguments. - Use the computed property: You can then use the computed property in your template or within other methods of your component as if it were a regular data property.
Here's a basic structure for using a computed property in a uni-app component:
export default { data() { return { // Your data properties here firstName: 'John', lastName: 'Doe' }; }, computed: { fullName() { return this.firstName ' ' this.lastName; } } }
In this example, fullName
is a computed property that depends on firstName
and lastName
. Whenever firstName
or lastName
changes, fullName
will automatically update.
What are the benefits of using computed properties in uni-app development?
Using computed properties in uni-app development offers several benefits:
- Reactivity: Computed properties are reactive, meaning they automatically update when their dependent values change. This eliminates the need for manual updates, reducing the risk of errors and making your code more maintainable.
- Readability: By encapsulating complex logic within computed properties, your templates and methods remain clean and easier to read. This separation of concerns makes your code more organized.
- Efficiency: Computed properties are cached based on their reactive dependencies. If the dependencies have not changed, the cached result is returned, saving computational resources. This can improve performance, especially with complex calculations.
- Reusability: Computed properties can be reused throughout your component, reducing code duplication. You can use them in templates, methods, or even within other computed properties.
- Declarative Data Flow: Computed properties support a declarative approach to data flow, making it easier to understand the state of your application and how it changes over time.
Can you provide an example of how to implement a computed property in a uni-app project?
Let's say you're working on a uni-app project where you need to display the total price of items in a shopping cart. Here's an example of how to implement a computed property to calculate the total price:
export default { data() { return { cartItems: [ { name: 'Item 1', price: 10, quantity: 2 }, { name: 'Item 2', price: 15, quantity: 1 } ] }; }, computed: { totalPrice() { return this.cartItems.reduce((total, item) => { return total (item.price * item.quantity); }, 0); } }, template: ` <view> <text>Total Price: {{ totalPrice }}</text> </view> ` }
In this example, totalPrice
is a computed property that calculates the total price of items in the cart by iterating through the cartItems
array. Whenever cartItems
changes, totalPrice
will automatically update, and the new total will be reflected in the template.
How do computed properties in uni-app differ from regular data properties?
Computed properties and regular data properties in uni-app serve different purposes and have several key differences:
- Derivation vs. Storage: Computed properties are derived from other data in your component, whereas regular data properties are used to store raw data directly. Computed properties do not hold their own state; they depend on other data properties.
- Reactivity: Both computed properties and data properties are reactive, meaning they can trigger updates in the UI when they change. However, computed properties update automatically when their dependencies change, while data properties must be manually updated.
- Caching: Computed properties are cached based on their reactive dependencies. If the dependencies haven't changed since the last computation, the cached result is returned. Data properties do not have this caching mechanism.
- Usage: Computed properties are typically used for complex calculations or transformations of data, making it easier to manage the state of your application. Data properties are used for storing the initial state or user input that doesn't require complex logic.
-
Declaration: Computed properties are declared in the
computed
field of your component options, while data properties are declared in thedata
field.
In summary, computed properties are powerful tools for managing derived data in uni-app, enhancing reactivity, readability, and efficiency, while data properties are used for storing raw data.
The above is the detailed content of How do I use computed properties in uni-app?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor
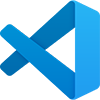
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Zend Studio 13.0.1
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
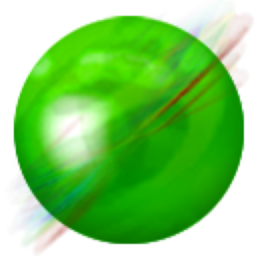
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
