How do I handle navigation between pages in uni-app?
In uni-app, handling navigation between pages can be accomplished using several built-in APIs that simplify the process. The core of page navigation in uni-app revolves around a few key methods:
-
uni.navigateTo(Object): This method is used to retain the current page in the stack and open a new page. When the new page is closed, you return to the original page. Here's an example of how to use it:
uni.navigateTo({ url: 'path/to/page' });
-
uni.redirectTo(Object): This method is used to close the current page and open a new one. Unlike
navigateTo
, this method does not keep the current page in the stack.uni.redirectTo({ url: 'path/to/new-page' });
-
uni.reLaunch(Object): This method is used to close all opened pages and open a new page. This is useful when you want to clear the navigation stack.
uni.reLaunch({ url: 'path/to/relaunch-page' });
-
uni.switchTab(Object): This method is used to navigate to a tabbar page, which is defined in your pages.json file.
uni.switchTab({ url: '/pages/index/index' });
-
uni.navigateBack(Object): This method is used to return to the previous page. You can specify the number of pages to go back by setting the
delta
parameter.uni.navigateBack({ delta: 1 });
By utilizing these methods, you can effectively manage navigation between pages in your uni-app project.
What are the best practices for managing page navigation in uni-app?
Managing page navigation efficiently in uni-app involves adhering to best practices that enhance user experience and app performance. Here are some best practices to consider:
- Consistent Navigation Pattern: Ensure that your navigation pattern is consistent throughout the app. This helps users understand how to move through different sections of your app.
- Use of Tabbar: If your app has several main sections, consider using a tabbar. It's easier for users to switch between different sections and can improve navigation efficiency.
- Minimize Navigation Stack Depth: Try to keep the navigation stack as shallow as possible. Deep navigation stacks can be confusing and make it harder for users to navigate back to the home screen.
- Clear and Descriptive URLs: Use clear and descriptive URLs for each page. This not only helps in development and debugging but also makes it easier to maintain and update the navigation structure.
-
Proper Use of Navigation Methods: Choose the appropriate navigation method for your use case. For example, use
navigateTo
for opening details pages,redirectTo
for replacing the current page, andreLaunch
for resetting the navigation stack. - Testing Navigation Flows: Regularly test the navigation flows in your app to ensure they are logical and efficient. This helps in identifying and fixing any navigation issues that could confuse users.
- Accessibility: Ensure that your navigation is accessible, including the use of appropriate labels and visual indicators for navigation elements.
By following these best practices, you can create a navigation system in uni-app that is user-friendly and efficient.
Can I use custom animations for page transitions in uni-app?
Yes, you can use custom animations for page transitions in uni-app. While uni-app provides default animations for page transitions, you can customize these animations by modifying the pageTransition
property in the app.vue
file or using CSS transitions and animations directly on the page elements.
To set custom page transition animations in the app.vue
file, you can use the following example:
export default { onLaunch: function() { console.log('App Launch') }, onShow: function() { console.log('App Show') }, onHide: function() { console.log('App Hide') }, globalData: { userInfo: null }, pageTransition: { enterTransition: 'fade-in', leaveTransition: 'fade-out' } }
You can define the fade-in
and fade-out
transitions in your CSS file:
@keyframes fade-in { from { opacity: 0; } to { opacity: 1; } } @keyframes fade-out { from { opacity: 1; } to { opacity: 0; } } .fade-in { animation: fade-in 0.3s ease-in-out; } .fade-out { animation: fade-out 0.3s ease-in-out; }
In addition to using app.vue
for global transitions, you can apply custom animations directly to elements using CSS or JavaScript, allowing for more granular control over the page transition effects.
How do I pass data between pages during navigation in uni-app?
Passing data between pages during navigation in uni-app can be achieved through several methods. Here are the most common approaches:
-
Using URL Parameters: You can pass data as query parameters in the URL when navigating between pages. This method is suitable for small amounts of data.
uni.navigateTo({ url: 'path/to/page?name=John&age=30' });
To access the data in the target page, use the
onLoad
method:export default { onLoad: function(options) { let name = options.name; let age = options.age; console.log(name, age); } }
-
Using uni.setStorageSync and uni.getStorageSync: For larger amounts of data or data that needs to be persisted across sessions, you can use the storage API to pass data between pages.
// In the source page let userData = {name: 'John', age: 30}; uni.setStorageSync('userData', userData); // Navigate to the new page uni.navigateTo({ url: 'path/to/page' }); // In the target page export default { onLoad: function() { let userData = uni.getStorageSync('userData'); console.log(userData.name, userData.age); } }
-
Using Global Variables: You can also pass data using global variables defined in the
app.vue
file. This method is useful for sharing data that needs to be accessible across multiple pages.// In app.vue export default { globalData: { userData: null } } // In the source page let app = getApp(); app.globalData.userData = {name: 'John', age: 30}; // In the target page export default { onLoad: function() { let app = getApp(); let userData = app.globalData.userData; console.log(userData.name, userData.age); } }
By utilizing these methods, you can effectively pass data between pages in your uni-app project, ensuring seamless communication and data transfer between different parts of your application.
The above is the detailed content of How do I handle navigation between pages in uni-app?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
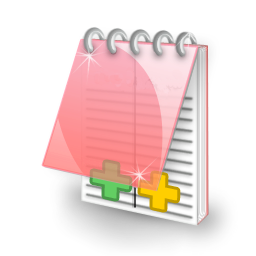
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 English version
Recommended: Win version, supports code prompts!

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
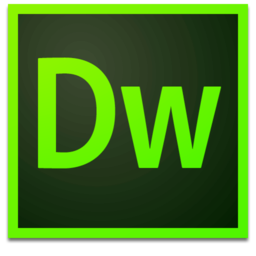
Dreamweaver Mac version
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
