


How do I use uni-app's API for accessing device features (camera, geolocation, etc.)?
How do I use uni-app's API for accessing device features (camera, geolocation, etc.)?
To use uni-app's API for accessing device features, you need to familiarize yourself with the specific APIs provided for different device functionalities. Here’s a brief guide on how to use some of these APIs:
-
Camera: To use the camera API, you can call
uni.chooseImage
to let users select images from the camera or photo album. For real-time camera access, you can useuni.createCameraContext
to create a camera context and manipulate the camera within your app.uni.chooseImage({ count: 1, // Number of images to choose sizeType: ['original', 'compressed'], // Original or compressed sourceType: ['camera'], // Source can be camera or album success: function (res) { const tempFilePaths = res.tempFilePaths // Handle the result } });
-
Geolocation: For geolocation, you can use
uni.getLocation
to get the current location of the device. This can be used for location-based services or mapping features.uni.getLocation({ type: 'wgs84', success: function (res) { console.log('Latitude: ' res.latitude); console.log('Longitude: ' res.longitude); } });
Each API has its own set of parameters and callback functions to handle the success and failure scenarios. You should refer to the uni-app documentation for a detailed list of all supported APIs and their parameters.
What specific permissions are required to use the camera and geolocation in uni-app?
To use the camera and geolocation features in a uni-app project, you must ensure that the necessary permissions are declared in your app's configuration file (manifest.json
). Here are the specific permissions you need to include:
-
Camera Permission: Add the following to the
manifest.json
file under thepermissions
section:"permissions": { "camera": true }
-
Geolocation Permission: Similarly, for geolocation, you need to include:
"permissions": { "location": true }
These permissions need to be requested at runtime as well, depending on the platform. For instance, on Android, you might need to call uni.authorize
to request these permissions explicitly before using the related APIs.
uni.authorize({ scope: 'scope.camera', success() { // User has authorized the camera access } });
Remember, handling permissions varies across different platforms, and you should consult the uni-app documentation for detailed platform-specific guidelines.
Can you provide a code example of how to implement geolocation tracking in a uni-app project?
Here’s a simple example of how you can implement geolocation tracking in a uni-app project. This code snippet uses uni.getLocation
to get the user's current location and updates it every few seconds:
let watchId; function startTracking() { watchId = uni.startLocationUpdate({ success: function (res) { console.log('Location update started'); }, fail: function (err) { console.error('Failed to start location update: ', err); } }); uni.onLocationChange(function (res) { console.log('Current Location: ' res.latitude ', ' res.longitude); // You can send this data to a server or update your UI }); } function stopTracking() { uni.stopLocationUpdate({ success: function (res) { console.log('Location update stopped'); uni.offLocationChange(); // Stop listening to location changes }, fail: function (err) { console.error('Failed to stop location update: ', err); } }); } // Start tracking when the app initializes startTracking(); // Stop tracking when the app closes or when needed // stopTracking();
This example sets up a continuous location update which you can then use to track the user's movements. Remember to handle the start and stop of the tracking appropriately in your app lifecycle.
How do I handle potential errors when accessing device features like the camera in uni-app?
Handling errors when accessing device features like the camera in uni-app involves understanding the structure of the API responses and implementing error handling within your callback functions. Here's how you can approach this:
-
Using Callbacks: Most uni-app APIs use callback functions to handle success and error states. You should always implement the
fail
andcomplete
callbacks alongside thesuccess
callback to handle errors effectively.uni.chooseImage({ count: 1, sizeType: ['original', 'compressed'], sourceType: ['camera'], success: function (res) { const tempFilePaths = res.tempFilePaths console.log('Image selected:', tempFilePaths); }, fail: function (err) { console.error('Failed to access camera:', err); // Handle the error, perhaps by displaying a user-friendly message uni.showToast({ title: 'Failed to access camera. Please try again.', icon: 'none' }); }, complete: function () { // This will always be called, whether the operation succeeded or failed console.log('Camera access attempt completed'); } });
-
Permission Errors: Since permissions are required for accessing features like the camera, you should also check if the necessary permissions are granted. If not, you can guide the user to grant these permissions.
uni.authorize({ scope: 'scope.camera', success() { // Permission granted, proceed with camera operations }, fail() { // Permission denied, handle it appropriately uni.showModal({ title: 'Permission Required', content: 'Please grant camera permission to proceed.', success: function (res) { if (res.confirm) { uni.openSetting(); // Open app settings to allow user to change permissions } } }); } });
By implementing these strategies, you can ensure a smoother user experience even when errors occur. Always make sure to log errors for debugging purposes and provide clear feedback to the user.
The above is the detailed content of How do I use uni-app's API for accessing device features (camera, geolocation, etc.)?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
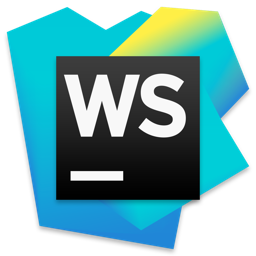
WebStorm Mac version
Useful JavaScript development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Chinese version
Chinese version, very easy to use
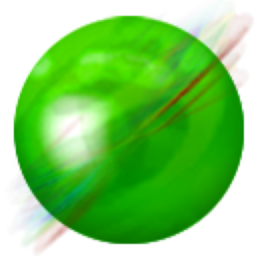
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
