Enhance user experience on information-rich pages with a simple, in-page search function. Forget database queries or JSON parsing – this method directly searches the rendered text content of a webpage. While browser built-in search exists, this approach offers a refined filtering experience, highlighting relevant results for easier navigation.
Here's a live demo showcasing the functionality: [link to demo]
I utilize this technique in a real-world project: https://www.php.cn/link/2a60eed05079970d61abad679da7ae8f.
Leveraging JavaScript
This tutorial employs JavaScript to manage all interactive elements. Specifically, it will:
- Identify searchable content.
- Monitor user input in the search field.
- Filter the
innerText
of searchable elements. - Check if text includes the search term (
.includes()
is key here). - Toggle element visibility based on search term matches.
Basic HTML Structure
Let's assume an FAQ page where each question is presented as a "card" with a title and content:
<h1 id="FAQ-Section">FAQ Section</h1> <div> <h3 id="Who-are-we">Who are we</h3> <p>It has survived not only five centuries, but also the leap into electronic typesetting, remaining essentially unchanged. It was popularized </p> </div> <div> <h3 id="What-we-do">What we do</h3> <p>It has survived not only five centuries, but also the leap into electronic typesetting, remaining essentially unchanged. It was popularized </p> </div> <div> <h3 id="Why-work-here">Why work here</h3> <p>It has survived not only five centuries, but also the leap into electronic typesetting, remaining essentially unchanged. It was popularized</p> </div> <div> <h3 id="Learn-more">Learn more</h3> <p>Want to learn more about us?</p> </div>
For a page with numerous questions, this structure scales effectively.
To enable interactivity, we'll use a single CSS rule:
.is-hidden { display: none; }
This class will be dynamically added or removed to control element visibility. We'll also add a search input:
<label for="searchbox">Search:</label> <input type="text" id="searchbox">
Core JavaScript Functionality
The following JavaScript code manages the search functionality:
function liveSearch() { let cards = document.querySelectorAll('.cards'); // Select card elements let searchQuery = document.getElementById("searchbox").value; // Get search term cards.forEach(card => { let textContent = card.innerText.toLowerCase(); //Normalize to lowercase let searchTermLower = searchQuery.toLowerCase(); //Normalize to lowercase if (textContent.includes(searchTermLower)) { card.classList.remove('is-hidden'); // Show matching cards } else { card.classList.add('is-hidden'); // Hide non-matching cards } }); } // Add a small delay to prevent excessive function calls let typingTimer; let typeInterval = 500; // Half a second let searchInput = document.getElementById('searchbox'); searchInput.addEventListener('keyup', () => { clearTimeout(typingTimer); typingTimer = setTimeout(liveSearch, typeInterval); });
The code iterates through cards, checks for search term inclusion (case-insensitive), and updates visibility accordingly. A delay prevents performance issues from rapid keystrokes.
Expanding Search Capabilities: Fuzzy Matching
To incorporate fuzzy matching (searching for related keywords, even if not explicitly displayed), consider using hidden elements or attributes:
Method 1: Hidden Elements
Add hidden elements containing keywords:
<div> <h3 id="Who-are-we">Who are we</h3> <p>...</p> <span class="hidden-keywords">secret, company, history</span> </div>
Modify liveSearch
to include textContent
instead of innerText
to access hidden keywords.
Method 2: Attributes
Use attributes like alt
for images:
<img src="/static/imghwm/default1.png" data-src="https://img.php.cn/upload/article/000/000/000/174227042512545.jpg?x-oss-process=image/resize,p_40" class="lazy" alt="In-Page Filtered Search With Vanilla JavaScript ">
Adjust liveSearch
to use getAttribute('alt')
to search attribute values.
Important Note
This search method is limited to content already rendered in the DOM. It's not suitable for searching external databases or large datasets.
Conclusion
This simple, yet effective, in-page search solution, implemented with vanilla JavaScript, can significantly improve the usability of web pages with extensive content. Adapt and expand upon this technique to suit various applications, from FAQ pages to image galleries.
The above is the detailed content of In-Page Filtered Search With Vanilla JavaScript. For more information, please follow other related articles on the PHP Chinese website!
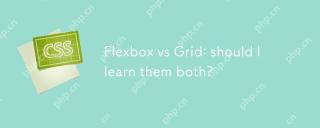
Yes,youshouldlearnbothFlexboxandGrid.1)Flexboxisidealforone-dimensional,flexiblelayoutslikenavigationmenus.2)Gridexcelsintwo-dimensional,complexdesignssuchasmagazinelayouts.3)Combiningbothenhanceslayoutflexibilityandresponsiveness,allowingforstructur
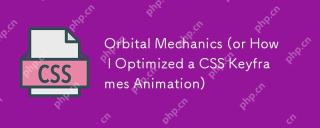
What does it look like to refactor your own code? John Rhea picks apart an old CSS animation he wrote and walks through the thought process of optimizing it.
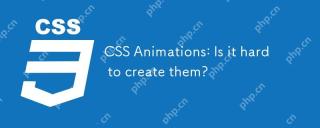
CSSanimationsarenotinherentlyhardbutrequirepracticeandunderstandingofCSSpropertiesandtimingfunctions.1)Startwithsimpleanimationslikescalingabuttononhoverusingkeyframes.2)Useeasingfunctionslikecubic-bezierfornaturaleffects,suchasabounceanimation.3)For
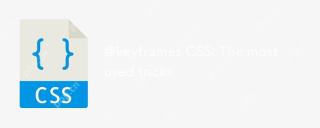
@keyframesispopularduetoitsversatilityandpowerincreatingsmoothCSSanimations.Keytricksinclude:1)Definingsmoothtransitionsbetweenstates,2)Animatingmultiplepropertiessimultaneously,3)Usingvendorprefixesforbrowsercompatibility,4)CombiningwithJavaScriptfo
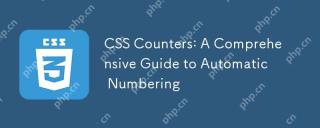
CSSCountersareusedtomanageautomaticnumberinginwebdesigns.1)Theycanbeusedfortablesofcontents,listitems,andcustomnumbering.2)Advancedusesincludenestednumberingsystems.3)Challengesincludebrowsercompatibilityandperformanceissues.4)Creativeusesinvolvecust
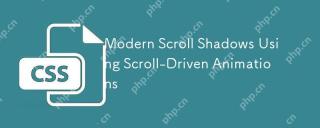
Using scroll shadows, especially for mobile devices, is a subtle bit of UX that Chris has covered before. Geoff covered a newer approach that uses the animation-timeline property. Here’s yet another way.
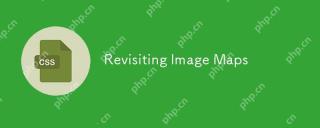
Let’s run through a quick refresher. Image maps date all the way back to HTML 3.2, where, first, server-side maps and then client-side maps defined clickable regions over an image using map and area elements.
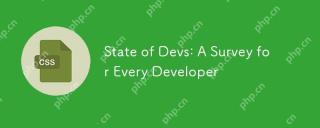
The State of Devs survey is now open to participation, and unlike previous surveys it covers everything except code: career, workplace, but also health, hobbies, and more.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
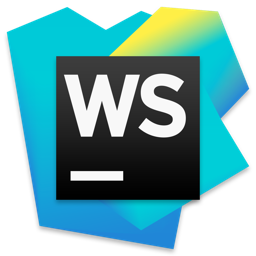
WebStorm Mac version
Useful JavaScript development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Linux new version
SublimeText3 Linux latest version
