How do I use uni-app with third-party mapping libraries?
To use uni-app with third-party mapping libraries, you'll need to follow these steps:
- Choose a Mapping Library: Popular third-party mapping libraries that work with uni-app include AMap (Gaode Map), Baidu Map, and Google Maps. Depending on your target region, select the most suitable library.
-
Install the SDK: Each mapping library has its own SDK. For instance:
- For AMap, you can use
uni-app
's plugin system to install theamap-wx
SDK by adding it to yourmanifest.json
file. - For Baidu Map, you might need to manually include the SDK in your project or use a similar plugin.
- For Google Maps, you'll typically include the JavaScript API directly in your
index.html
file.
- For AMap, you can use
- Configure the SDK: Each mapping library requires some form of configuration. This usually involves setting an API key or client ID in your project's configuration files or directly in your code.
-
Integrate the Map into Your App: You'll typically create a component or page in your
uni-app
that initializes and displays the map. Here's an example of how you might use AMap:<template> <view> <map id="map" style="width: 100%; height: 300px;"></map> </view> </template> <script> export default { data() { return { mapContext: null, }; }, onReady() { this.initMap(); }, methods: { initMap() { this.mapContext = uni.createMapContext('map', this); this.mapContext.initAMap({ key: 'your_amap_key', success: function(res) { console.log('AMap initialized successfully'); } }); } } } </script>
- Use Mapping Functions: After initializing the map, you can use the library's API to perform functions like adding markers, drawing routes, or geocoding.
What are the best practices for integrating third-party mapping libraries into uni-app?
Integrating third-party mapping libraries into uni-app requires careful consideration to ensure a smooth and efficient user experience. Here are some best practices:
- Use Asynchronous Loading: Load the mapping libraries asynchronously to prevent blocking the main thread. This improves the perceived performance of your app.
- Optimize for Mobile Devices: Since uni-app is primarily used for developing mobile apps, ensure that your map integration is optimized for touch interactions and limited screen real estate.
- Manage API Keys Securely: Store API keys and sensitive information in environment variables or configuration files that are not part of your version control system to prevent accidental exposure.
- Leverage Caching: Use caching mechanisms to store map data locally where possible to reduce network requests and improve load times.
- Handle Errors Gracefully: Implement error handling to manage situations where the map fails to load or where there are API issues. This ensures that your app remains functional even when the mapping service is unavailable.
- Responsive Design: Ensure that your map component is responsive and adapts well to different screen sizes and orientations.
- Performance Testing: Regularly test your app's performance, especially the map component, to identify and address any bottlenecks or lag.
Can I use multiple third-party mapping libraries simultaneously in uni-app, and how?
Yes, you can use multiple third-party mapping libraries simultaneously in uni-app, but it requires careful management to avoid conflicts and performance issues. Here’s how you can do it:
- Separate Components: Create separate components for each mapping library. This keeps the logic for each map isolated and manageable.
- Conditional Rendering: Use conditional rendering in your templates to show only the relevant map based on user selection or geolocation.
- API Key Management: Ensure that you manage API keys for each service separately and securely.
- Event Handling: Handle events and interactions for each map independently to avoid conflicts.
Here is an example of how you might implement this:
<template> <view> <button @click="showAMap">Show AMap</button> <button @click="showBMap">Show Baidu Map</button> <map v-if="showAMapFlag" id="amap" style="width: 100%; height: 300px;"></map> <map v-if="showBMapFlag" id="bmap" style="width: 100%; height: 300px;"></map> </view> </template> <script> export default { data() { return { showAMapFlag: false, showBMapFlag: false, }; }, methods: { showAMap() { this.showAMapFlag = true; this.showBMapFlag = false; // Initialize AMap here }, showBMap() { this.showAMapFlag = false; this.showBMapFlag = true; // Initialize Baidu Map here } } } </script>
Are there any specific performance considerations when using third-party mapping libraries in uni-app?
Yes, there are specific performance considerations when using third-party mapping libraries in uni-app:
- Initialization Overhead: The initial loading and setup of a mapping library can be resource-intensive. Consider lazy-loading the map component to mitigate this.
- Network Requests: Mapping libraries often make numerous network requests to fetch tiles and data. Optimize these by enabling caching and reducing the frequency of requests where possible.
- Memory Usage: Maps, particularly those with high detail levels, can consume significant amounts of memory. Monitor your app’s memory usage and consider reducing the map’s resolution or area if necessary.
- Rendering Performance: High-quality maps can strain the device's graphics capabilities. Use lower detail levels or fewer layers if performance issues arise.
- API Rate Limits: Be aware of and manage API rate limits to avoid disruptions in service. This might involve implementing rate limiting in your app to spread out requests.
- Battery Consumption: On mobile devices, maps can lead to increased battery consumption due to continuous updates and data fetching. Implement strategies to limit this, such as reducing the refresh rate of the map when the app is in the background.
- Cross-Platform Compatibility: Ensure that the mapping library performs well across all platforms supported by uni-app. This might involve additional optimizations for specific operating systems or devices.
By carefully managing these aspects, you can ensure that your uni-app remains performant and user-friendly even with integrated third-party mapping libraries.
The above is the detailed content of How do I use uni-app with third-party mapping libraries?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
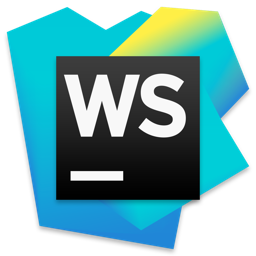
WebStorm Mac version
Useful JavaScript development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Chinese version
Chinese version, very easy to use
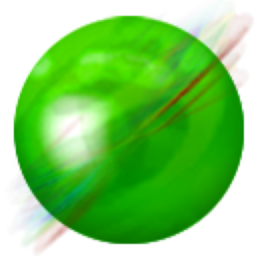
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
