


How do I perform basic operations with Redis data structures (SET, GET, LPUSH, RPUSH, SADD, HSET)?
Redis is an open-source, in-memory data structure store that can be used as a database, cache, and message broker. It supports various data structures, and here's how to perform basic operations on them:
-
SET: The SET command is used to set the value of a key. It overwrites the old value if the key already exists.
SET key value
-
GET: The GET command is used to get the value of a key. If the key does not exist, it returns
nil
.GET key
-
LPUSH: The LPUSH command is used to insert all the specified values at the head of the list stored at the key. If the key does not exist, it will be created as an empty list before performing the push operation.
LPUSH key value1 value2 value3
-
RPUSH: The RPUSH command is similar to LPUSH but inserts values at the tail of the list.
RPUSH key value1 value2 value3
-
SADD: The SADD command is used to add one or more members to a set. If the key does not exist, a new set is created.
SADD key member1 member2 member3
-
HSET: The HSET command is used to set the value of a field in a hash stored at key. If the key does not exist, a new key holding a hash is created.
HSET key field value
These commands are fundamental operations used to interact with Redis data structures. It's important to understand the use cases for each to maximize efficiency.
What are the best practices for managing Redis data structures efficiently?
Efficient management of Redis data structures is crucial for performance optimization. Here are some best practices:
- Choose the Right Data Structure: Understand the differences between Redis data structures (e.g., strings, lists, sets, hashes) and choose the one that best fits your use case. For example, use lists for queues or stacks, sets for unique collections, and hashes for storing objects.
-
Use Expiry Times: Set expiration times for keys that are not needed indefinitely. This helps in managing memory and prevents data from becoming stale.
SETEX key seconds value
-
Batch Operations: Whenever possible, use batch operations to reduce network round trips. For example, use
MSET
for setting multiple keys orMGET
for getting multiple values.MSET key1 value1 key2 value2 MGET key1 key2
- Avoid Large Keys: Large keys can lead to performance issues. If you need to store large amounts of data, consider breaking it down into smaller keys or using Redis Cluster to distribute data across multiple nodes.
- Use Redis Persistence: Depending on your use case, choose either RDB or AOF persistence. RDB is faster but may result in data loss, while AOF offers greater data integrity but may impact performance.
-
Monitor and Optimize Memory Usage: Use Redis's built-in commands like
INFO memory
to monitor memory usage andMEMORY USAGE key
to check the memory used by specific keys. Optimize your data model accordingly.
How can I troubleshoot common issues when using Redis commands like SET and GET?
Troubleshooting Redis can involve several common issues related to commands like SET and GET. Here are some steps to diagnose and resolve them:
-
Key Not Found: If a GET command returns
nil
, it means the key does not exist. Verify the key name and check if it was set correctly.GET non-existent-key
-
Connection Issues: If you cannot connect to Redis, check the server status, port configuration, and network settings. Use the
PING
command to test the connection.PING
- Data Persistence: If data is not being persisted as expected, verify your persistence settings. Ensure that you are using RDB or AOF correctly and that the server has write permissions to the persistence files.
-
Performance Problems: If Redis is slow, use the
SLOWLOG
command to identify slow queries and theINFO
command to monitor performance metrics. Optimize your data model and consider scaling your Redis instance if necessary.SLOWLOG GET INFO
-
Memory Issues: If Redis is using too much memory, use
MEMORY USAGE
to identify large keys andINFO memory
to monitor overall memory usage. Implement eviction policies and manage key expiration times effectively.
What are some advanced techniques for optimizing Redis data structure operations?
Advanced techniques for optimizing Redis data structure operations can significantly enhance performance. Here are some strategies:
-
Pipeline Commands: Use command pipelining to send multiple commands to Redis in a single network round trip. This can dramatically reduce latency for bulk operations.
# Example in Redis CLI with pipelining enabled redis-cli --pipe < commands.txt
-
Lua Scripts: Use Redis's Lua scripting to execute complex operations in a single step. This reduces the number of round trips and allows for atomic operations.
EVAL "return redis.call('SET', KEYS[1], ARGV[1])" 1 mykey myvalue
-
Pub/Sub Pattern: Implement a pub/sub pattern to enable real-time communication between clients. This can be useful for notification systems and real-time updates.
SUBSCRIBE channel PUBLISH channel message
- Redis Cluster: Use Redis Cluster for horizontal scaling. This distributes data across multiple nodes, improving read and write performance for large datasets.
-
HyperLogLog: Use HyperLogLog for counting unique elements in large datasets with minimal memory usage. This is particularly useful for analytics and counting unique visitors to a website.
PFADD hll element1 element2 element3 PFCOUNT hll
-
Redis Streams: Use Redis Streams for reliable message queuing and event sourcing. This provides a more powerful alternative to lists for managing time-series data and events.
XADD mystream * field1 value1 field2 value2 XRANGE mystream -
By implementing these advanced techniques, you can optimize Redis operations for better performance and scalability.
The above is the detailed content of How do I perform basic operations with Redis data structures (SET, GET, LPUSH, RPUSH, SADD, HSET)?. For more information, please follow other related articles on the PHP Chinese website!
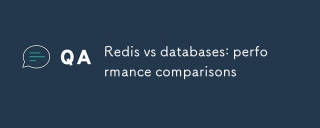
Redisoutperformstraditionaldatabasesinspeedforread/writeoperationsduetoitsin-memorynature,whiletraditionaldatabasesexcelincomplexqueriesanddataintegrity.1)Redisisidealforreal-timeanalyticsandcaching,offeringphenomenalperformance.2)Traditionaldatabase
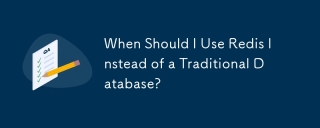
UseRedisinsteadofatraditionaldatabasewhenyourapplicationrequiresspeedandreal-timedataprocessing,suchasforcaching,sessionmanagement,orreal-timeanalytics.Redisexcelsin:1)Caching,reducingloadonprimarydatabases;2)Sessionmanagement,simplifyingdatahandling
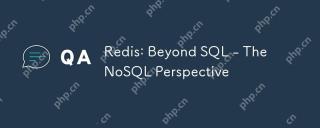
Redis goes beyond SQL databases because of its high performance and flexibility. 1) Redis achieves extremely fast read and write speed through memory storage. 2) It supports a variety of data structures, such as lists and collections, suitable for complex data processing. 3) Single-threaded model simplifies development, but high concurrency may become a bottleneck.
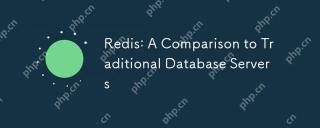
Redis is superior to traditional databases in high concurrency and low latency scenarios, but is not suitable for complex queries and transaction processing. 1.Redis uses memory storage, fast read and write speed, suitable for high concurrency and low latency requirements. 2. Traditional databases are based on disk, support complex queries and transaction processing, and have strong data consistency and persistence. 3. Redis is suitable as a supplement or substitute for traditional databases, but it needs to be selected according to specific business needs.
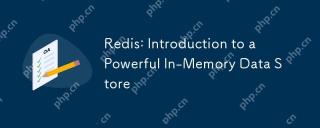
Redisisahigh-performancein-memorydatastructurestorethatexcelsinspeedandversatility.1)Itsupportsvariousdatastructureslikestrings,lists,andsets.2)Redisisanin-memorydatabasewithpersistenceoptions,ensuringfastperformanceanddatasafety.3)Itoffersatomicoper
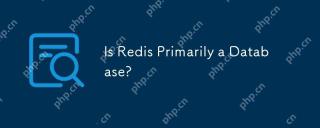
Redis is primarily a database, but it is more than just a database. 1. As a database, Redis supports persistence and is suitable for high-performance needs. 2. As a cache, Redis improves application response speed. 3. As a message broker, Redis supports publish-subscribe mode, suitable for real-time communication.
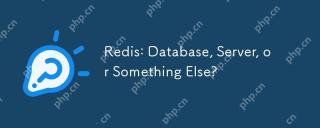
Redisisamultifacetedtoolthatservesasadatabase,server,andmore.Itfunctionsasanin-memorydatastructurestore,supportsvariousdatastructures,andcanbeusedasacache,messagebroker,sessionstorage,andfordistributedlocking.
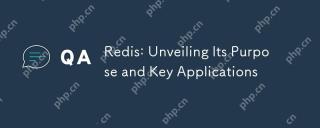
Redisisanopen-source,in-memorydatastructurestoreusedasadatabase,cache,andmessagebroker,excellinginspeedandversatility.Itiswidelyusedforcaching,real-timeanalytics,sessionmanagement,andleaderboardsduetoitssupportforvariousdatastructuresandfastdataacces


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
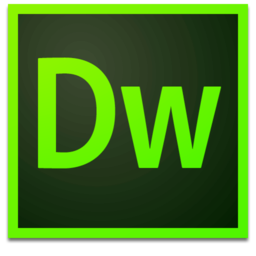
Dreamweaver Mac version
Visual web development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
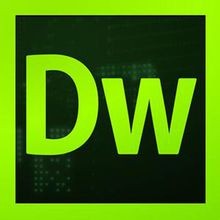
Dreamweaver CS6
Visual web development tools
