How do I use Java's try-with-resources statement for automatic resource management?
How do I use Java's try-with-resources statement for automatic resource management?
Java's try-with-resources statement is designed to simplify the management of resources, such as file streams or database connections, that need to be closed after their use. This statement was introduced in Java 7 as part of the Automatic Resource Block Management (ARM) feature.
To use the try-with-resources statement, you need to declare resource variables that implement the AutoCloseable
or its subinterface Closeable
within the try
clause. These resources will be automatically closed at the end of the statement, whether the block of code completes normally or an exception is thrown.
Here’s an example of how to use it:
try (FileInputStream fis = new FileInputStream("input.txt"); FileOutputStream fos = new FileOutputStream("output.txt")) { // Use the resources byte[] buffer = new byte[1024]; int bytesRead; while ((bytesRead = fis.read(buffer)) != -1) { fos.write(buffer, 0, bytesRead); } } // fis and fos are automatically closed here
In this example, FileInputStream
and FileOutputStream
both implement Closeable
, so they can be used within a try-with-resources block. Once the block ends, these streams are automatically closed, eliminating the need for a finally
block to manually close them.
What types of resources can be managed using Java's try-with-resources?
The try-with-resources statement can manage any resource that implements the AutoCloseable
interface. AutoCloseable
is a base interface in Java that defines a single method close()
which is called automatically when the resource is no longer needed. The Closeable
interface extends AutoCloseable
and is used specifically for resources that deal with I/O operations.
Common types of resources that can be managed include:
-
File Streams: Such as
FileInputStream
andFileOutputStream
, which are used for reading from and writing to files. -
Database Connections: Such as
Connection
,Statement
, andResultSet
objects used for database operations. -
Network Sockets: Such as
Socket
andServerSocket
used for network communication. -
Custom Resources: Any custom class that implements
AutoCloseable
and manages resources that need to be closed.
By implementing AutoCloseable
, developers can create their own classes that can be used within a try-with-resources block, ensuring proper resource cleanup.
How does the try-with-resources statement improve code readability and maintainability?
The try-with-resources statement improves code readability and maintainability in several ways:
-
Reduced Boilerplate Code: It eliminates the need for a
finally
block to manually close resources. This results in less code and fewer lines to maintain. -
Improved Exception Handling: With traditional try-catch-finally blocks, exception handling in the
finally
block can sometimes mask or interfere with exceptions thrown in thetry
block. Try-with-resources ensures that resources are closed without masking any exceptions. - Clearer Resource Scope: By declaring resources directly in the try statement, it is immediately clear what resources are being managed and where they are being used.
- Ensured Resource Closure: The automatic closing of resources prevents common bugs related to forgetting to close resources, which can lead to resource leaks.
Here's an example comparing traditional and try-with-resources approaches:
Traditional:
FileInputStream fis = null; try { fis = new FileInputStream("input.txt"); // Use fis } catch (IOException e) { // Handle exception } finally { if (fis != null) { try { fis.close(); } catch (IOException e) { // Handle exception from closing } } }
Try-with-resources:
try (FileInputStream fis = new FileInputStream("input.txt")) { // Use fis } // fis is automatically closed
The latter is much clearer and reduces the chances of leaving resources open.
What are the best practices for handling exceptions when using try-with-resources in Java?
When using try-with-resources in Java, it's essential to follow best practices for exception handling to maintain the robustness and clarity of your code:
- Catching Multiple Exceptions: You can catch different types of exceptions that might be thrown from within the try block or during resource closure:
try (FileInputStream fis = new FileInputStream("input.txt")) { // Use fis } catch (IOException e) { // Handle IOException } catch (Exception e) { // Handle other exceptions }
-
Suppressed Exceptions: When an exception is thrown in the try block and another exception occurs during the automatic closing of resources, the latter becomes a suppressed exception of the former. You can access these suppressed exceptions using the
getSuppressed()
method:
try (FileInputStream fis = new FileInputStream("input.txt")) { // Use fis } catch (IOException e) { for (Throwable se : e.getSuppressed()) { // Handle suppressed exceptions } }
- Re-throwing Exceptions: If you need to handle the primary exception but re-throw it or a custom exception, you can do so while still dealing with any suppressed exceptions:
try (FileInputStream fis = new FileInputStream("input.txt")) { // Use fis } catch (IOException e) { for (Throwable se : e.getSuppressed()) { // Handle suppressed exceptions } throw new CustomException("Failed to process file", e); }
- Logging: Always log exceptions, especially those related to resource handling, to ensure that you have a record of what went wrong:
try (FileInputStream fis = new FileInputStream("input.txt")) { // Use fis } catch (IOException e) { logger.error("An error occurred while processing the file", e); for (Throwable se : e.getSuppressed()) { logger.error("Suppressed exception occurred", se); } }
By following these practices, you ensure that your code using try-with-resources handles exceptions in a clean and effective manner, improving both its robustness and maintainability.
The above is the detailed content of How do I use Java's try-with-resources statement for automatic resource management?. For more information, please follow other related articles on the PHP Chinese website!
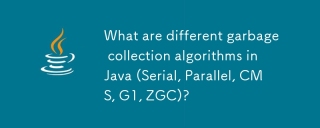
The article discusses various Java garbage collection algorithms (Serial, Parallel, CMS, G1, ZGC), their performance impacts, and suitability for applications with large heaps.

The article discusses the Java Virtual Machine (JVM), detailing its role in running Java programs across different platforms. It explains the JVM's internal processes, key components, memory management, garbage collection, and performance optimizatio
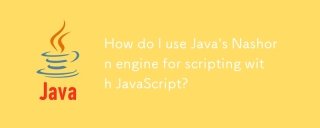
Java's Nashorn engine enables JavaScript scripting within Java apps. Key steps include setting up Nashorn, managing scripts, and optimizing performance. Main issues involve security, memory management, and future compatibility due to Nashorn's deprec
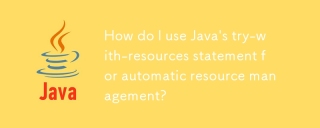
Java's try-with-resources simplifies resource management by automatically closing resources like file streams or database connections, improving code readability and maintainability.
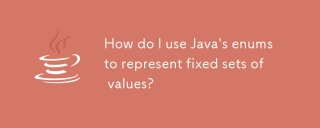
Java enums represent fixed sets of values, offering type safety, readability, and additional functionality through custom methods and constructors. They enhance code organization and can be used in switch statements for efficient value handling.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
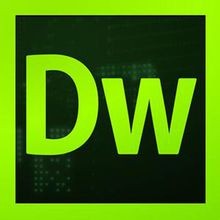
Dreamweaver CS6
Visual web development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),