How to Implement Real-Time Data Synchronization with Swoole and MySQL?
Implementing real-time data synchronization with Swoole and MySQL involves leveraging Swoole's asynchronous capabilities to handle data efficiently and MySQL's transactional features to ensure data integrity. Here's a step-by-step guide to set up this system:
-
Setup Swoole Server:
Start by setting up a Swoole server that can handle WebSocket or HTTP connections. Swoole's coroutine-based programming model allows for efficient handling of multiple concurrent connections.$server = new Swoole\WebSocket\Server("0.0.0.0", 9501); $server->on('open', function($server, $request) { echo "connection open: {$request->fd}\n"; }); $server->on('message', function($server, $frame) { echo "received message: {$frame->data}\n"; $server->push($frame->fd, json_encode(["message" => $frame->data])); }); $server->on('close', function($server, $fd) { echo "connection close: {$fd}\n"; }); $server->start();
-
Connect to MySQL:
Use Swoole's coroutine MySQL client to connect to the database. This client allows for non-blocking database operations, which is crucial for maintaining performance.$db = new Swoole\Coroutine\MySQL(); $db->connect([ 'host' => '127.0.0.1', 'port' => 3306, 'user' => 'username', 'password' => 'password', 'database' => 'database' ]);
-
Implement Data Synchronization:
Use a combination of Swoole's coroutine and MySQL's replication or triggers to synchronize data in real-time. For example, you can set up a coroutine to periodically check for updates and broadcast them to connected clients.$server->on('workerStart', function($server, $workerId) use($db) { Swoole\Timer::tick(1000, function() use($db, $server) { $result = $db->query('SELECT * FROM updates WHERE processed = 0'); foreach($result as $row) { $server->push($row['clientId'], json_encode($row)); $db->query("UPDATE updates SET processed = 1 WHERE id = {$row['id']}"); } }); });
What are the best practices for optimizing Swoole and MySQL for real-time data sync?
To optimize Swoole and MySQL for real-time data synchronization, consider the following best practices:
-
Use Coroutines Efficiently:
Leverage Swoole's coroutines to handle database operations asynchronously, reducing wait times and improving overall throughput. -
Optimize Database Queries:
Ensure your MySQL queries are optimized. Use indexing, limit the amount of data retrieved, and consider using database views or stored procedures for complex operations. -
Implement Caching:
Use caching mechanisms (like Redis) to reduce the load on the database. Store frequently accessed data in memory to speed up read operations. -
Connection Pooling:
Use Swoole's connection pooling to manage database connections efficiently. This reduces the overhead of establishing new connections and helps scale the application. -
Monitor and Scale:
Regularly monitor the performance of both Swoole and MySQL. Use tools like Swoole's built-in metrics and MySQL's performance schema to identify bottlenecks and scale resources accordingly. -
Use Transactions:
When updating data, use MySQL transactions to ensure data consistency, especially when dealing with multiple operations that need to be atomic.
Which Swoole features should I prioritize for efficient MySQL data synchronization?
When focusing on efficient MySQL data synchronization with Swoole, prioritize the following features:
-
Coroutines:
Swoole's coroutines enable asynchronous, non-blocking I/O operations, which are crucial for handling multiple concurrent connections and database operations without performance degradation. -
Connection Pooling:
This feature helps manage a pool of database connections, reducing the overhead associated with creating new connections for each operation, thus improving performance and scalability. -
Timer:
The Timer API in Swoole allows for scheduling periodic tasks, which can be used to poll the database for changes or updates, ensuring timely synchronization. -
WebSocket Support:
WebSocket enables real-time, bidirectional communication between the server and clients, which is ideal for pushing updates as soon as they are available. -
Asynchronous MySQL Client:
Swoole's coroutine MySQL client enables non-blocking database queries, which are essential for maintaining high performance during synchronization tasks.
How can I ensure data consistency when using Swoole for real-time updates with MySQL?
Ensuring data consistency when using Swoole for real-time updates with MySQL involves several strategies:
-
Use Transactions:
MySQL transactions ensure that a series of database operations are completed atomically. UseSTART TRANSACTION
andCOMMIT
to wrap your update operations.$db->query('START TRANSACTION'); $db->query('UPDATE table SET column = value WHERE condition'); $db->query('COMMIT');
-
Implement Optimistic Locking:
Use versioning or timestamps in your records to prevent concurrent updates from causing conflicts. If a conflict occurs, you can retry the operation or merge changes manually. -
Use Binlog for Replication:
MySQL's binary log (binlog) can be used to replicate changes to another database, which can then be used to ensure data consistency across different systems. -
Ensure Idempotency:
Design your update operations to be idempotent, so repeating the same operation multiple times has the same effect as performing it once. This helps manage retries and ensures consistency. -
Monitor and Log:
Use logging and monitoring tools to track database operations and detect any anomalies or inconsistencies. Tools like Swoole's logging API and MySQL's error logs can help identify issues quickly.
By following these strategies and leveraging Swoole's powerful features, you can maintain high data consistency while achieving real-time synchronization with MySQL.
The above is the detailed content of How to Implement Real-Time Data Synchronization with Swoole and MySQL?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
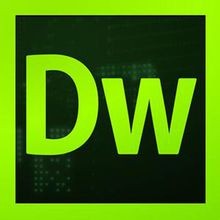
Dreamweaver CS6
Visual web development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 Chinese version
Chinese version, very easy to use