Creating and Using Stored Procedures and Functions in PL/SQL
Creating and using stored procedures and functions in PL/SQL involves several key steps. First, you need to understand the basic syntax. Stored procedures are blocks of PL/SQL code that perform a specific task, often involving multiple SQL statements. They don't return a value directly. Functions, on the other hand, are similar but always return a single value.
Creating a Stored Procedure:
CREATE OR REPLACE PROCEDURE my_procedure (param1 IN NUMBER, param2 OUT VARCHAR2) AS variable1 NUMBER := 0; BEGIN -- Your PL/SQL code here SELECT COUNT(*) INTO variable1 FROM my_table WHERE column1 = param1; param2 := 'Record count: ' || variable1; EXCEPTION WHEN OTHERS THEN param2 := 'Error occurred'; END; /
This example shows a procedure my_procedure
that takes a number as input (param1
) and returns a string message through an output parameter (param2
). The /
at the end is a crucial part of the syntax in SQL*Plus or SQL Developer to execute the command.
Creating a Function:
CREATE OR REPLACE FUNCTION my_function (param1 IN NUMBER) RETURN NUMBER AS variable1 NUMBER := 0; BEGIN SELECT SUM(column2) INTO variable1 FROM my_table WHERE column1 = param1; RETURN variable1; EXCEPTION WHEN NO_DATA_FOUND THEN RETURN 0; END; /
This function my_function
takes a number as input and returns the sum of a column from a table. Note the RETURN
statement, essential for functions. The EXCEPTION
block handles the case where no data is found.
Using Stored Procedures and Functions:
Stored procedures are called using the EXECUTE
statement or within other PL/SQL blocks:
EXECUTE my_procedure(10, :output_variable); DBMS_OUTPUT.PUT_LINE(:output_variable);
Functions can be called directly within SQL statements or PL/SQL blocks:
SELECT my_function(20) FROM dual; SELECT column1, my_function(column1) FROM my_table;
Best Practices for Optimizing PL/SQL Stored Procedures and Functions for Performance
Optimizing PL/SQL for performance involves several strategies focusing on efficient SQL and PL/SQL coding practices.
-
Minimize Context Switching: Reduce the number of times your code switches between the PL/SQL engine and the SQL engine. This is achieved by fetching data in bulk using
FORALL
statements instead of individualINSERT
orUPDATE
statements in loops. -
Use Bulk Operations: Use
FORALL
statements for bulk DML operations. This significantly reduces the overhead of repeated context switches. -
Efficient Data Retrieval: Use appropriate
WHERE
clauses to filter data efficiently. Avoid usingSELECT *
and instead specify only the necessary columns. - Index Optimization: Ensure that appropriate indexes are created on the tables your PL/SQL code accesses. Indexes dramatically speed up data retrieval.
-
Avoid Cursors When Possible: Cursors can be performance bottlenecks. If possible, use set-based operations (e.g.,
SELECT INTO
) instead of explicit cursors. If you must use cursors, consider using implicit cursors where appropriate or optimizing cursor fetching. - Proper Data Type Usage: Use appropriate data types to avoid implicit conversions, which can impact performance.
- Debugging and Profiling: Use PL/SQL Profiler or other debugging tools to identify performance bottlenecks. This allows for targeted optimization efforts.
- Code Review: Regular code reviews help identify areas for improvement and prevent the introduction of performance issues.
Handling Exceptions and Errors Within PL/SQL Stored Procedures and Functions
Error handling is crucial for robust PL/SQL code. The EXCEPTION
block allows you to gracefully handle errors without crashing the entire application.
BEGIN -- Your PL/SQL code here EXCEPTION WHEN NO_DATA_FOUND THEN -- Handle NO_DATA_FOUND exception DBMS_OUTPUT.PUT_LINE('No data found.'); WHEN OTHERS THEN -- Handle other exceptions DBMS_OUTPUT.PUT_LINE('An error occurred: ' || SQLERRM); -- Log the error for later analysis END;
This example demonstrates a basic EXCEPTION
block. The WHEN OTHERS
clause catches any unhandled exceptions. SQLERRM
provides the error message. It's essential to log errors for debugging and monitoring purposes. More specific exception handling is preferable over a general WHEN OTHERS
block to provide more informative error messages and facilitate better debugging. Consider using custom exceptions for specific application errors.
Key Differences Between PL/SQL Stored Procedures and Functions, and When to Use Each
The primary difference lies in their return values:
- Stored Procedures: Do not return values directly. They perform actions and can modify data, but any output is typically through OUT parameters or by modifying database tables.
- Functions: Always return a single value. They are often used for calculations or retrieving specific data. They can be used within SQL statements.
When to use Stored Procedures:
- Performing complex database operations involving multiple SQL statements.
- Updating or modifying data in multiple tables.
- Performing tasks that don't require a single return value.
- Creating reusable units of code for various database operations.
When to use Functions:
- Calculating a single value based on input parameters.
- Retrieving a single piece of information from the database.
- Using the result directly within SQL statements.
- Creating reusable units of code for computations or data retrieval.
In essence, use procedures for actions and functions for calculations and data retrieval. The choice depends on the specific task you need to accomplish. If you need to return a single value, a function is the better choice. If you are performing a series of actions without a single return value, a procedure is more suitable.
The above is the detailed content of How do I create and use stored procedures and functions in PL/SQL?. For more information, please follow other related articles on the PHP Chinese website!

Oracleoffersacomprehensivesuiteofproductsandservicesincludingdatabasemanagement,cloudcomputing,enterprisesoftware,andhardwaresolutions.1)OracleDatabasesupportsvariousdatamodelswithefficientmanagementfeatures.2)OracleCloudInfrastructure(OCI)providesro

The development history of Oracle software from database to cloud computing includes: 1. Originated in 1977, it initially focused on relational database management system (RDBMS), and quickly became the first choice for enterprise-level applications; 2. Expand to middleware, development tools and ERP systems to form a complete set of enterprise solutions; 3. Oracle database supports SQL, providing high performance and scalability, suitable for small to large enterprise systems; 4. The rise of cloud computing services further expands Oracle's product line to meet all aspects of enterprise IT needs.

MySQL and Oracle selection should be based on cost, performance, complexity and functional requirements: 1. MySQL is suitable for projects with limited budgets, is simple to install, and is suitable for small to medium-sized applications. 2. Oracle is suitable for large enterprises and performs excellently in handling large-scale data and high concurrent requests, but is costly and complex in configuration.

Oracle helps businesses achieve digital transformation and data management through its products and services. 1) Oracle provides a comprehensive product portfolio, including database management systems, ERP and CRM systems, helping enterprises automate and optimize business processes. 2) Oracle's ERP systems such as E-BusinessSuite and FusionApplications realize end-to-end business process automation, improve efficiency and reduce costs, but have high implementation and maintenance costs. 3) OracleDatabase provides high concurrency and high availability data processing, but has high licensing costs. 4) Performance optimization and best practices include the rational use of indexing and partitioning technology, regular database maintenance and compliance with coding specifications.
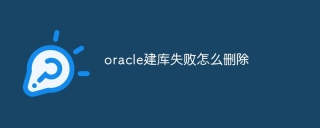
Steps to delete the failed database after Oracle failed to build a library: Use sys username to connect to the target instance. Use DROP DATABASE to delete the database. Query v$database to confirm that the database has been deleted.

In Oracle, the FOR LOOP loop can create cursors dynamically. The steps are: 1. Define the cursor type; 2. Create the loop; 3. Create the cursor dynamically; 4. Execute the cursor; 5. Close the cursor. Example: A cursor can be created cycle-by-circuit to display the names and salaries of the top 10 employees.
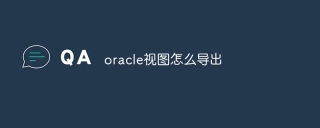
Oracle views can be exported through the EXP utility: Log in to the Oracle database. Start the EXP utility, specifying the view name and export directory. Enter export parameters, including target mode, file format, and tablespace. Start exporting. Verify the export using the impdp utility.
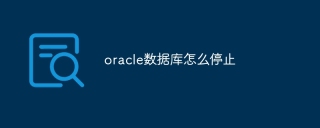
To stop an Oracle database, perform the following steps: 1. Connect to the database; 2. Shutdown immediately; 3. Shutdown abort completely.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
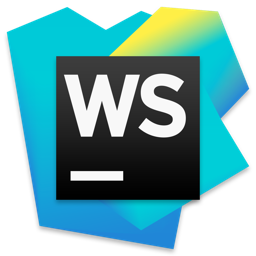
WebStorm Mac version
Useful JavaScript development tools
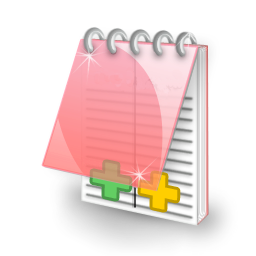
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
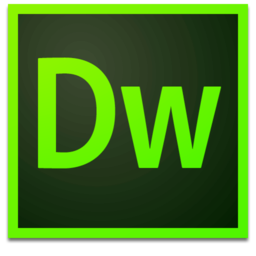
Dreamweaver Mac version
Visual web development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.