How to Use Java's Cryptographic APIs for Encryption and Decryption?
Java provides a robust set of cryptographic APIs within the java.security
package and its subpackages. These APIs allow developers to perform various cryptographic operations, including encryption and decryption. The core classes involved are Cipher
, SecretKey
, SecretKeyFactory
, and KeyGenerator
. Here's a breakdown of how to use them for symmetric encryption (using AES):
1. Key Generation:
First, you need to generate a secret key. This key is crucial for both encryption and decryption. The following code snippet demonstrates how to generate a 256-bit AES key:
import javax.crypto.Cipher; import javax.crypto.KeyGenerator; import javax.crypto.SecretKey; import javax.crypto.spec.SecretKeySpec; import java.security.NoSuchAlgorithmException; import java.security.SecureRandom; import java.util.Base64; public class AESEncryption { public static void main(String[] args) throws NoSuchAlgorithmException { // Generate a 256-bit AES key KeyGenerator keyGenerator = KeyGenerator.getInstance("AES"); keyGenerator.init(256, new SecureRandom()); SecretKey secretKey = keyGenerator.generateKey(); // ... (rest of the code for encryption and decryption) ... } }
2. Encryption:
Once you have the key, you can use the Cipher
class to encrypt your data. The following code shows how to encrypt a string using AES in CBC mode with PKCS5Padding:
import javax.crypto.Cipher; import javax.crypto.NoSuchPaddingException; import javax.crypto.SecretKey; import javax.crypto.spec.IvParameterSpec; import java.security.InvalidAlgorithmParameterException; import java.security.InvalidKeyException; import java.security.NoSuchAlgorithmException; import java.util.Base64; import java.util.Arrays; // ... (previous code for key generation) ... byte[] iv = new byte[16]; // Initialization Vector (IV) - must be randomly generated new SecureRandom().nextBytes(iv); IvParameterSpec ivParameterSpec = new IvParameterSpec(iv); Cipher cipher = Cipher.getInstance("AES/CBC/PKCS5Padding"); cipher.init(Cipher.ENCRYPT_MODE, secretKey, ivParameterSpec); byte[] encryptedBytes = cipher.doFinal("This is my secret message".getBytes()); String encryptedString = Base64.getEncoder().encodeToString(iv) Base64.getEncoder().encodeToString(encryptedBytes); //Combine IV and encrypted data for later decryption System.out.println("Encrypted: " encryptedString); } }
3. Decryption:
Decryption is similar to encryption, but you use Cipher.DECRYPT_MODE
. Remember to use the same key, IV, and algorithm parameters:
// ... (previous code for key generation and encryption) ... String[] parts = encryptedString.split("\\s "); // Split the string into IV and encrypted data byte[] decodedIv = Base64.getDecoder().decode(parts[0]); byte[] decodedEncryptedBytes = Base64.getDecoder().decode(parts[1]); IvParameterSpec ivParameterSpecDec = new IvParameterSpec(decodedIv); Cipher decipher = Cipher.getInstance("AES/CBC/PKCS5Padding"); decipher.init(Cipher.DECRYPT_MODE, secretKey, ivParameterSpecDec); byte[] decryptedBytes = decipher.doFinal(decodedEncryptedBytes); System.out.println("Decrypted: " new String(decryptedBytes)); } }
Remember to handle exceptions appropriately in a production environment. This example provides a basic illustration. For more complex scenarios, consider using keystores and other security best practices.
What are the Best Practices for Secure Key Management When Using Java Cryptography?
Secure key management is paramount in cryptography. Compromised keys render your encryption useless. Here are some best practices:
-
Use strong key generation: Employ algorithms like AES with sufficient key lengths (at least 256 bits). Use a cryptographically secure random number generator (CSPRNG) like
SecureRandom
. - Key storage: Never hardcode keys directly into your application. Use a secure keystore provided by the Java Cryptography Architecture (JCA) or a dedicated hardware security module (HSM). Keystores provide mechanisms for password protection and key management.
- Key rotation: Regularly rotate your keys to limit the impact of a potential compromise. Implement a scheduled key rotation process.
- Access control: Restrict access to keys based on the principle of least privilege. Only authorized personnel or systems should have access to keys.
- Key destruction: When a key is no longer needed, securely destroy it. Overwriting the key data multiple times is a common approach, but HSMs offer more robust key destruction mechanisms.
- Avoid key reuse: Never reuse the same key for multiple purposes or across different applications.
- Use a Key Management System (KMS): For enterprise-level applications, consider using a dedicated KMS that offers advanced features like key lifecycle management, auditing, and integration with other security systems.
Which Java Cryptographic Algorithms Are Most Suitable for Different Security Needs?
The choice of algorithm depends on your specific security needs and constraints. Here's a brief overview:
-
Symmetric Encryption (for confidentiality):
- AES (Advanced Encryption Standard): Widely considered the most secure and efficient symmetric algorithm for most applications. Use 256-bit keys for maximum security.
- ChaCha20: A modern stream cipher offering strong security and performance, especially on systems with limited resources.
-
Asymmetric Encryption (for confidentiality and digital signatures):
- RSA: A widely used algorithm for digital signatures and key exchange. However, it's computationally more expensive than symmetric algorithms. Use key sizes of at least 2048 bits.
- ECC (Elliptic Curve Cryptography): Provides comparable security to RSA with smaller key sizes, making it more efficient for resource-constrained environments.
-
Hashing (for integrity and authentication):
- SHA-256/SHA-512: Secure hash algorithms providing collision resistance. SHA-512 offers slightly higher security but is computationally more expensive.
- HMAC (Hash-based Message Authentication Code): Provides message authentication and integrity. Combine with a strong hash function like SHA-256 or SHA-512.
-
Digital Signatures (for authentication and non-repudiation):
- RSA and ECDSA (Elliptic Curve Digital Signature Algorithm): Both are widely used for creating digital signatures. ECDSA is generally more efficient than RSA.
Remember to always use the strongest algorithm that your system can efficiently handle and keep up-to-date with the latest security advisories.
Are There Any Common Pitfalls to Avoid When Implementing Encryption and Decryption in Java?
Several common pitfalls can weaken the security of your encryption implementation:
- Incorrect IV handling: Using a non-random or reused IV with block ciphers like AES in CBC mode significantly reduces security. Always generate a cryptographically secure random IV for each encryption operation.
- Weak or hardcoded keys: Never hardcode keys directly in your code. Use a secure keystore and follow key management best practices.
- Improper padding: Using incorrect or insecure padding schemes can lead to vulnerabilities like padding oracle attacks. Use well-established padding schemes like PKCS5Padding or PKCS7Padding.
- Algorithm misuse: Choosing an inappropriate algorithm or using it incorrectly can severely compromise security. Carefully consider the security requirements of your application and select the appropriate algorithm and mode of operation.
- Insufficient key length: Using key lengths that are too short makes your encryption vulnerable to brute-force attacks. Always use the recommended key lengths for chosen algorithms.
- Ignoring exception handling: Properly handling exceptions is crucial for secure and robust cryptography. Failure to handle exceptions can lead to vulnerabilities or data loss.
- Improper data sanitization: Failing to sanitize data before encryption can lead to injection attacks. Sanitize data appropriately before encrypting.
-
Insecure random number generation: Using a weak random number generator can weaken the security of your keys and IVs. Always use a CSPRNG like
SecureRandom
.
By carefully considering these pitfalls and following best practices, you can significantly improve the security of your Java cryptography implementations. Remember that security is an ongoing process, and staying updated with the latest security advisories and best practices is crucial.
The above is the detailed content of How do I use Java's cryptographic APIs for encryption and decryption?. For more information, please follow other related articles on the PHP Chinese website!

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
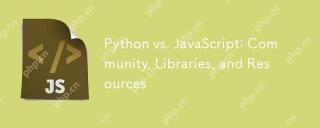
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
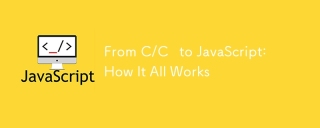
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
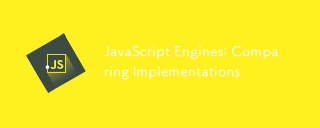
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
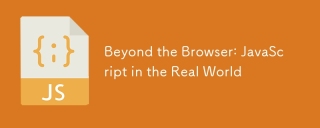
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.
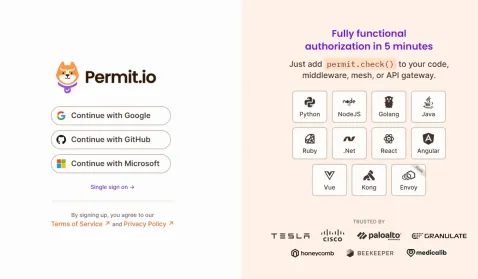
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
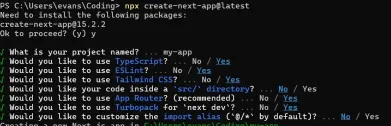
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
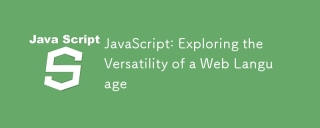
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
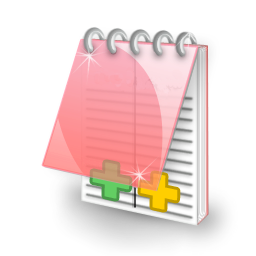
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
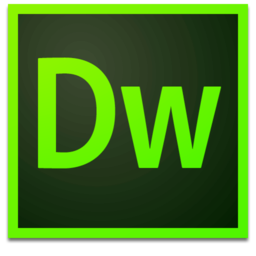
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor