


How do I handle user authentication and authorization in Java web applications?
Handling User Authentication and Authorization in Java Web Applications
User authentication and authorization are critical aspects of securing any Java web application. Authentication verifies the identity of a user, confirming that they are who they claim to be. Authorization, on the other hand, determines what resources a user is allowed to access after successful authentication. In Java web applications, this typically involves several steps:
- Receiving Credentials: The process begins when a user attempts to log in, providing credentials like a username and password (or other authentication factors like multi-factor authentication). This usually happens through an HTML form submitted to a servlet or controller.
-
Authentication: The application then needs to verify these credentials. This often involves:
- Database Lookup: Comparing the provided credentials against a database of registered users. It's crucial to store passwords securely, using hashing algorithms like bcrypt or Argon2 to prevent them from being easily compromised.
- Third-Party Authentication: Leveraging services like OAuth 2.0 or OpenID Connect to authenticate users through existing accounts (e.g., Google, Facebook). This simplifies the authentication process and enhances security.
- Token-Based Authentication (JWT): Generating JSON Web Tokens (JWTs) after successful authentication. These tokens contain user information and are used for subsequent requests, eliminating the need for repeated authentication.
-
Authorization: Once authenticated, the application needs to determine what actions the user is permitted to perform. This can involve:
- Role-Based Access Control (RBAC): Assigning users to roles (e.g., administrator, editor, user) and defining permissions for each role.
- Attribute-Based Access Control (ABAC): More granular control based on various attributes of the user, resource, and environment.
- Access Control Lists (ACLs): Explicitly defining which users or groups have access to specific resources.
- Session Management: Maintaining user sessions after successful authentication. This typically involves using HTTP sessions or tokens to track the user's activity and access rights. Proper session management is crucial to prevent session hijacking.
- Secure Coding Practices: Implementing robust input validation to prevent SQL injection and cross-site scripting (XSS) attacks is essential. Regular security audits and penetration testing can further improve the application's security posture.
Best-Suited Security Frameworks for Java Web Apps
Several robust frameworks simplify the implementation of authentication and authorization in Java web applications:
- Spring Security: This is arguably the most popular framework, offering a comprehensive set of features including authentication, authorization, session management, and protection against various web vulnerabilities. It supports various authentication mechanisms (database, LDAP, OAuth 2.0, etc.) and provides flexible authorization options (RBAC, ABAC).
- Keycloak: An open-source Identity and Access Management (IAM) solution that can be integrated with Java applications. It provides features like user management, authentication, authorization, and single sign-on (SSO). It's particularly useful for larger applications requiring centralized identity management.
- Shiro (Apache Shiro): Another powerful framework that offers authentication, authorization, session management, and cryptography features. It's known for its simplicity and ease of use, especially for smaller to medium-sized applications.
Integrating a Robust Authentication System
Integrating a robust authentication system into an existing Java web application depends on the chosen framework and the application's architecture. Generally, the process involves:
-
Adding the Framework Dependency: Include the necessary dependencies (e.g., Spring Security, Keycloak) in your project's
pom.xml
(for Maven) orbuild.gradle
(for Gradle). -
Configuring the Framework: Configure the framework according to your specific requirements, such as defining user roles, authentication providers, and authorization rules. This typically involves modifying configuration files (e.g.,
application.properties
or XML configuration files) or using annotations. -
Integrating with Existing Code: Modify your controllers and servlets to incorporate authentication and authorization checks. This might involve adding annotations (e.g.,
@PreAuthorize
in Spring Security) or using API calls provided by the chosen framework. - Testing Thoroughly: Rigorously test the integration to ensure that authentication and authorization work correctly and that all functionalities are secure.
Common Vulnerabilities and Mitigation Strategies
Several common vulnerabilities can compromise Java authentication and authorization:
- SQL Injection: Malicious users can inject SQL code into input fields to manipulate database queries, potentially gaining unauthorized access. Mitigation: Use parameterized queries or prepared statements to prevent SQL injection.
- Cross-Site Scripting (XSS): Attackers can inject malicious scripts into web pages to steal user data or perform other harmful actions. Mitigation: Encode user inputs properly and implement robust output encoding.
- Session Hijacking: Attackers can steal a user's session ID to impersonate them. Mitigation: Use secure session management techniques, including HTTPS, secure cookies (HttpOnly and Secure flags), and regular session timeout.
- Brute-Force Attacks: Attackers can attempt to guess usernames and passwords repeatedly. Mitigation: Implement rate limiting, account lockout mechanisms, and strong password policies.
- Broken Authentication: Weaknesses in the authentication process can allow attackers to bypass authentication or gain unauthorized access. Mitigation: Use strong password hashing algorithms, implement multi-factor authentication, and regularly audit the authentication system for vulnerabilities.
Addressing these vulnerabilities requires careful planning, secure coding practices, and the use of appropriate security frameworks and tools. Regular security audits and penetration testing are crucial to identify and address potential weaknesses.
The above is the detailed content of How do I handle user authentication and authorization in Java web applications?. For more information, please follow other related articles on the PHP Chinese website!
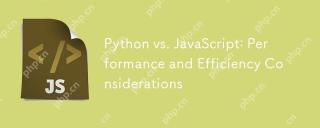
The differences in performance and efficiency between Python and JavaScript are mainly reflected in: 1) As an interpreted language, Python runs slowly but has high development efficiency and is suitable for rapid prototype development; 2) JavaScript is limited to single thread in the browser, but multi-threading and asynchronous I/O can be used to improve performance in Node.js, and both have advantages in actual projects.
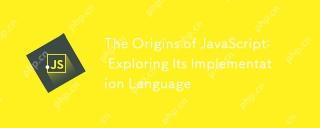
JavaScript originated in 1995 and was created by Brandon Ike, and realized the language into C. 1.C language provides high performance and system-level programming capabilities for JavaScript. 2. JavaScript's memory management and performance optimization rely on C language. 3. The cross-platform feature of C language helps JavaScript run efficiently on different operating systems.
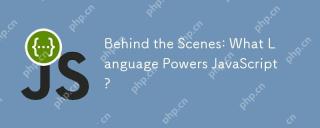
JavaScript runs in browsers and Node.js environments and relies on the JavaScript engine to parse and execute code. 1) Generate abstract syntax tree (AST) in the parsing stage; 2) convert AST into bytecode or machine code in the compilation stage; 3) execute the compiled code in the execution stage.
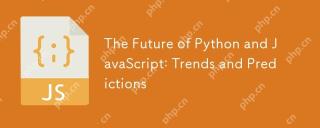
The future trends of Python and JavaScript include: 1. Python will consolidate its position in the fields of scientific computing and AI, 2. JavaScript will promote the development of web technology, 3. Cross-platform development will become a hot topic, and 4. Performance optimization will be the focus. Both will continue to expand application scenarios in their respective fields and make more breakthroughs in performance.
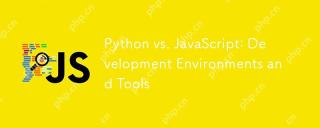
Both Python and JavaScript's choices in development environments are important. 1) Python's development environment includes PyCharm, JupyterNotebook and Anaconda, which are suitable for data science and rapid prototyping. 2) The development environment of JavaScript includes Node.js, VSCode and Webpack, which are suitable for front-end and back-end development. Choosing the right tools according to project needs can improve development efficiency and project success rate.
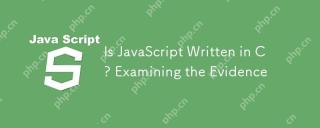
Yes, the engine core of JavaScript is written in C. 1) The C language provides efficient performance and underlying control, which is suitable for the development of JavaScript engine. 2) Taking the V8 engine as an example, its core is written in C, combining the efficiency and object-oriented characteristics of C. 3) The working principle of the JavaScript engine includes parsing, compiling and execution, and the C language plays a key role in these processes.
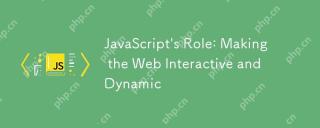
JavaScript is at the heart of modern websites because it enhances the interactivity and dynamicity of web pages. 1) It allows to change content without refreshing the page, 2) manipulate web pages through DOMAPI, 3) support complex interactive effects such as animation and drag-and-drop, 4) optimize performance and best practices to improve user experience.
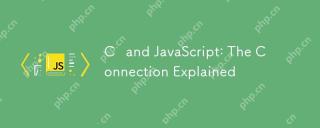
C and JavaScript achieve interoperability through WebAssembly. 1) C code is compiled into WebAssembly module and introduced into JavaScript environment to enhance computing power. 2) In game development, C handles physics engines and graphics rendering, and JavaScript is responsible for game logic and user interface.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
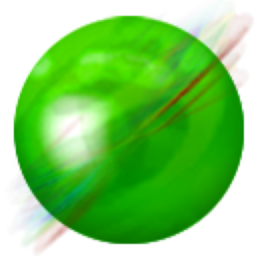
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
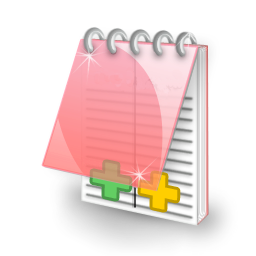
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Atom editor mac version download
The most popular open source editor
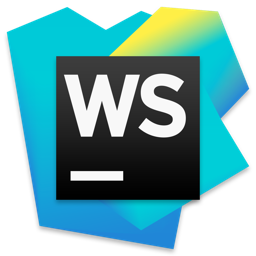
WebStorm Mac version
Useful JavaScript development tools
