How to Use Yii's Themes to Customize the Look and Feel of Your Application
Yii's theming system allows for significant customization of your application's appearance without modifying core code. It achieves this through the separation of presentation (views and assets) from application logic. The core process involves creating theme directories containing view files and assets that override the default application views and assets. Yii will automatically locate and use these themed versions if they exist.
To utilize themes, you first need to create a theme directory under the themes
directory within your application's web
directory. For example, a theme named "mytheme" would be located at web/themes/mytheme
. Inside this directory, you'll need to replicate the directory structure of your application's views, placing your customized views within. For instance, if you want to customize the index
view of your site
controller, you would create a file at web/themes/mytheme/views/site/index.php
. Similarly, assets (CSS, JavaScript, images) should be placed in a subdirectory named assets
within your theme directory. You can then reference these assets within your themed views using Yii's asset manager. Finally, you need to set the theme
property in your application configuration (config/web.php
):
return [ // ... other configurations ... 'theme' => 'mytheme', ];
This tells Yii to use the "mytheme" for rendering views. Any view files found within the theme directory will override their counterparts in the application's default views. Remember that the asset publishing path must be correctly configured to allow your theme's assets to be accessible by the browser.
Dynamically Switching Between Themes in a Yii Application
Yes, you can dynamically switch between themes in a Yii application. The most straightforward method involves modifying the application's theme
property during runtime. This can be done based on user preferences, device detection, or any other dynamic criteria.
One approach is to use a session variable to store the currently selected theme. A user might select their preferred theme via a settings page, and this selection would be stored in the session. Then, within your application's initialization (e.g., in the beforeRequest
event of your application component), you check the session for the theme preference and set the application's theme
property accordingly.
public function beforeRequest($event) { $theme = Yii::$app->session->get('theme', 'default'); // 'default' is the fallback theme Yii::$app->theme = $theme; }
This example assumes you have a session component configured in your application. The get('theme', 'default')
part retrieves the theme from the session, falling back to 'default' if not found. Alternatively, you can use cookies or database storage to persist the user's theme selection. The key is to change the Yii::$app->theme
property dynamically before the view is rendered. Remember to ensure that the theme you are switching to actually exists.
Creating a Custom Theme for Your Yii Application From Scratch
Creating a custom theme from scratch involves several steps. First, create the theme directory as described earlier (web/themes/mynewtheme
). Within this directory, create the necessary subdirectories to mirror your application's view structure. You'll then populate these directories with your custom view files (.php). These files should contain your HTML, CSS, and JavaScript code to define the theme's visual style.
For example, if you have a view located at views/site/index.php
, you would create a corresponding file at web/themes/mynewtheme/views/site/index.php
containing the themed version of that view. Remember to use Yii's view helpers and widgets to maintain consistency and leverage Yii's features.
Next, create an assets
subdirectory within your theme directory to store your theme's CSS, JavaScript, and image files. You can register these assets within your themed views using Yii's asset manager:
use yii\web\View; use yii\helpers\Html; $this->registerCssFile('@web/themes/mynewtheme/assets/style.css'); $this->registerJsFile('@web/themes/mynewtheme/assets/script.js');
Remember to replace @web
with your actual web root alias if it differs. Finally, configure your application to use the new theme by setting the theme
property in your application configuration as shown in the first answer. Thoroughly testing your new theme is crucial to ensure it functions correctly across different parts of your application.
Best Practices for Managing Multiple Themes in a Yii Project
Managing multiple themes efficiently requires a structured approach. Consider these best practices:
- Consistent Directory Structure: Maintain a consistent directory structure for all your themes. This makes it easier to locate files and maintain consistency across themes.
- Theme Inheritance: Utilize theme inheritance where appropriate. Create a base theme with common styles and layouts, and then extend this base theme for individual themes, reducing redundancy.
- Version Control: Use a version control system (like Git) to track changes to your themes, allowing for easy rollback and collaboration.
- Asset Management: Use a robust asset management strategy, perhaps utilizing a CSS preprocessor (like Sass or Less) and a JavaScript module bundler (like Webpack) to manage dependencies and minimize asset sizes.
- Configuration: Use configuration files (e.g., JSON or YAML) to store theme-specific settings, making it easier to manage and modify these settings without altering code.
- Modular Design: Break down your themes into smaller, reusable components (layouts, widgets, views) to promote reusability and maintainability.
- Theme Selection Mechanism: Implement a clear and user-friendly mechanism for selecting themes, whether through a settings page, user roles, or other criteria.
By following these best practices, you can effectively manage multiple themes in your Yii project, ensuring maintainability, scalability, and a streamlined development workflow.
The above is the detailed content of How do I use Yii's themes to customize the look and feel of my application?. For more information, please follow other related articles on the PHP Chinese website!
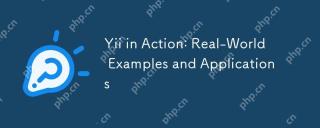
The Yii framework is suitable for developing web applications of all sizes, and its advantages lie in its high performance and rich feature set. 1) Yii adopts an MVC architecture, and its core components include ActiveRecord, Widget and Gii tools. 2) Through the request processing process, Yii efficiently handles HTTP requests. 3) Basic usage shows a simple example of creating controllers and views. 4) Advanced usage demonstrates the flexibility of database operations through ActiveRecord. 5) Debugging skills include using the debug toolbar and logging system. 6) Performance optimization It is recommended to use cache and database query optimization, follow coding specifications and dependency injection to improve code quality.
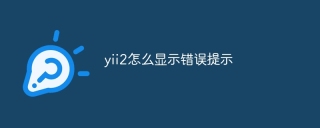
In Yii2, there are two main ways to display error prompts. One is to use Yii::$app->errorHandler->exception() to automatically catch and display errors when an exception occurs. The other is to use $this->addError(), which displays an error when model validation fails and can be accessed in the view through $model->getErrors(). In the view, you can use if ($errors = $model->getErrors())
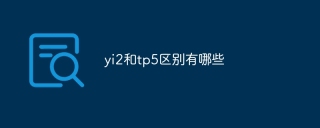
With the continuous development of PHP framework technology, Yi2 and TP5 have attracted much attention as the two mainstream frameworks. They are all known for their outstanding performance, rich functionality and robustness, but they have some differences and advantages and disadvantages. Understanding these differences is crucial for developers to choose frameworks.
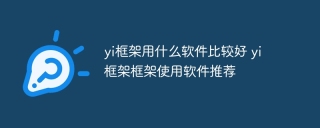
Abstract of the first paragraph of the article: When choosing software to develop Yi framework applications, multiple factors need to be considered. While native mobile application development tools such as XCode and Android Studio can provide strong control and flexibility, cross-platform frameworks such as React Native and Flutter are becoming increasingly popular with the benefits of being able to deploy to multiple platforms at once. For developers new to mobile development, low-code or no-code platforms such as AppSheet and Glide can quickly and easily build applications. Additionally, cloud service providers such as AWS Amplify and Firebase provide comprehensive tools
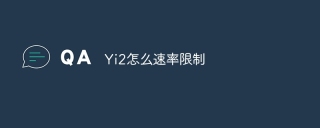
The Yi2 Rate Limiting Guide provides users with a comprehensive guide to how to control the data transfer rate in Yi2 applications. By implementing rate limits, users can optimize application performance, prevent excessive bandwidth consumption and ensure stable and reliable connections. This guide will introduce step-by-step how to configure the rate limit settings of Yi2, covering a variety of platforms and scenarios to meet the different needs of users.
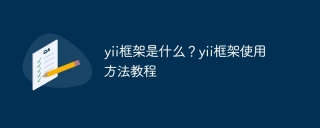
Article Summary: Yii Framework is an efficient and flexible PHP framework for creating dynamic and scalable web applications. It is known for its high performance, lightweight and easy to use features. This article will provide a comprehensive tutorial on the Yii framework, covering everything from installation to configuration to development of applications. This guide is designed to help beginners and experienced developers take advantage of the power of Yii to build reliable and maintainable web solutions.
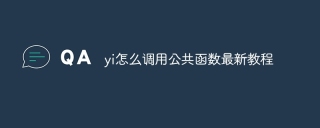
This article introduces the latest tutorial on calling public functions, which is implemented in Easy Language (Yi) language. For beginners, easy-to-language programming languages are easy to learn, and this article provides a detailed step-by-step guide to help users master how to call public functions in Yi applications. By following this tutorial, users will learn how to define, load, and call common functions, thereby enhancing their code reusability and flexibility.
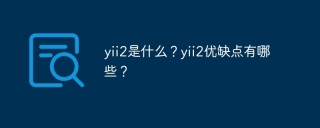
Yii2 is a powerful PHP framework that has been widely praised by developers. With its high performance, scalability and user-friendly interface, it becomes ideal for building large, complex web applications. However, like any framework, Yii2 has some advantages and disadvantages to consider.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
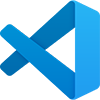
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
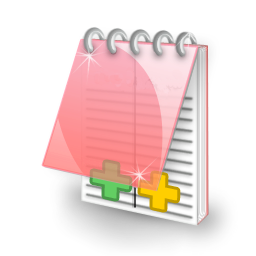
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Chinese version
Chinese version, very easy to use