


How to Handle Concurrency Safely in Workerman to Prevent Data Corruption
Workerman, being a high-performance asynchronous framework, inherently handles concurrency through its event-driven architecture. However, this doesn't automatically eliminate the risk of data corruption. To ensure data integrity, you need to carefully manage shared resources and implement appropriate synchronization mechanisms. The primary approach is to avoid sharing mutable state between different processes or threads as much as possible. If sharing is unavoidable, you must employ locking mechanisms.
Workerman excels at handling concurrent requests through its non-blocking I/O model, assigning each request to a separate worker process or thread. This minimizes the risk of race conditions compared to synchronous, multi-threaded applications. However, if you access shared resources like databases, files, or in-memory caches from multiple workers, data corruption can still occur. The solution is to treat these shared resources as critical sections and protect them using locks. For example, if you're updating a database counter, you need to ensure atomicity, often achieved through database transactions or appropriate locking at the database level. If using a shared in-memory cache, employ appropriate locking mechanisms provided by the caching library (e.g., Redis's atomic operations). Avoid using global variables or shared memory directly without proper synchronization.
Best Practices for Ensuring Data Integrity When Using Workerman's Multi-Process or Multi-Thread Capabilities
Maintaining data integrity in a multi-process or multi-threaded Workerman application requires a layered approach. The following best practices significantly reduce the risk of data corruption:
- Minimize Shared Resources: The fewer shared resources, the less chance of conflicts. Design your application to keep data localized within individual worker processes or threads whenever possible. Use message queues or other inter-process communication (IPC) mechanisms to exchange data between workers instead of sharing mutable data structures.
- Use Atomic Operations: When accessing shared resources, utilize atomic operations whenever possible. This ensures that operations are indivisible and prevent partial updates. Many databases and caching systems provide atomic increment/decrement, compare-and-swap, and other atomic operations.
- Implement Proper Locking: If atomic operations aren't sufficient, use locking mechanisms to protect critical sections. Workerman doesn't provide built-in locking mechanisms; you'll need to leverage external libraries or OS-level primitives (like mutexes or semaphores) depending on whether you're using multi-processing or multi-threading. Choose appropriate lock types based on your needs (e.g., mutexes for mutual exclusion, semaphores for controlling access to a limited resource). Always remember to release locks promptly to avoid deadlocks.
- Database Transactions: For database interactions, utilize transactions to ensure atomicity and consistency. Transactions group multiple database operations into a single unit of work, ensuring that either all operations succeed or none do.
- Careful Error Handling: Implement robust error handling to catch and recover from exceptions that might leave shared resources in an inconsistent state. Rollback transactions if errors occur within a critical section.
- Regular Testing: Thoroughly test your application under concurrent load to identify potential data integrity issues early on. Use load testing tools to simulate a large number of concurrent requests and monitor for data inconsistencies.
How to Implement Locking Mechanisms in My Workerman Application to Avoid Race Conditions
Workerman itself doesn't provide built-in locking mechanisms. The choice of locking mechanism depends on whether you're using multi-processing or multi-threading.
Multi-Processing: For multi-processing, you'll typically use inter-process communication (IPC) mechanisms like files, message queues (e.g., Redis, RabbitMQ), or shared memory with appropriate locking primitives provided by your operating system (e.g., POSIX semaphores, file locks). File locks offer a relatively simple approach for protecting shared files, while message queues provide more robust and scalable solutions for inter-process communication and synchronization.
Multi-Threading: In multi-threading scenarios, you'd generally use mutexes (mutual exclusion locks) or other synchronization primitives provided by your programming language's threading library (e.g., threading.Lock
in Python). Mutexes prevent multiple threads from simultaneously accessing a shared resource. Be mindful of potential deadlocks, which occur when two or more threads are blocked indefinitely, waiting for each other to release locks.
Example (Python with threading.Lock
):
import threading lock = threading.Lock() shared_resource = 0 def increment_counter(): global shared_resource with lock: # Acquire the lock shared_resource = 1 # Multiple threads calling increment_counter() will safely increment the counter.
Remember to choose the appropriate locking strategy for your application's architecture and scale requirements. Overuse of locks can introduce performance bottlenecks, so carefully identify the critical sections that require protection.
Common Pitfalls to Avoid When Managing Concurrent Requests in a Workerman-Based Application to Prevent Data Inconsistencies
Several common pitfalls can lead to data inconsistencies in concurrent Workerman applications:
- Ignoring Shared Resource Conflicts: Failing to recognize and address potential conflicts when multiple workers access the same resources (databases, files, caches) is a primary source of data corruption. Always assume that concurrent access is a possibility and implement appropriate synchronization mechanisms.
- Incorrect Locking Implementation: Improper use of locking mechanisms, such as deadlocks (where threads are blocked indefinitely), incorrect lock ordering, or failing to release locks, can lead to data inconsistencies and application crashes.
- Race Conditions: Failing to protect critical sections can result in race conditions, where the final outcome depends on the unpredictable order of execution of concurrent operations. This often manifests as data corruption or unexpected behavior.
- Unhandled Exceptions: Exceptions occurring within critical sections without proper rollback or error handling can leave shared resources in an inconsistent state. Implement robust error handling and transaction management.
- Insufficient Testing: Inadequate testing under concurrent load can mask subtle data integrity issues that only appear under high traffic conditions. Conduct thorough testing with realistic load scenarios to identify and address potential problems.
- Ignoring Data Consistency Guarantees: Not understanding or utilizing the data consistency guarantees provided by your database or caching system can lead to data inconsistencies. Utilize transactions, atomic operations, and appropriate locking mechanisms provided by these systems.
By diligently following these guidelines and best practices, you can significantly improve the reliability and data integrity of your Workerman-based application, even under heavy concurrent load.
The above is the detailed content of How do I handle concurrency safely in Workerman to prevent data corruption?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
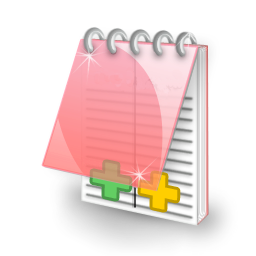
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Linux new version
SublimeText3 Linux latest version
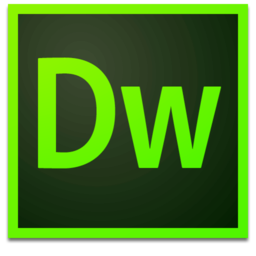
Dreamweaver Mac version
Visual web development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
