How to Build a Distributed Task Queue System with Swoole and RabbitMQ?
Building a Distributed Task Queue with Swoole and RabbitMQ
Building a distributed task queue system using Swoole and RabbitMQ involves leveraging the strengths of both technologies. Swoole, a high-performance asynchronous PHP framework, handles the task processing and worker management, while RabbitMQ acts as the robust message broker, ensuring reliable message delivery and queuing. The architecture generally consists of these components:
- RabbitMQ Server: This acts as the central message broker. Tasks are published as messages to RabbitMQ exchanges.
- Swoole Workers: Multiple Swoole worker processes consume messages from RabbitMQ queues. Each worker independently processes a task. The number of workers can be adjusted to match the system's load.
- Task Publisher: This component publishes tasks (serialized as messages) to the appropriate RabbitMQ exchange. This could be a separate Swoole server, a different application, or even a scheduled job.
- Message Queues: RabbitMQ queues hold the tasks awaiting processing by the Swoole workers. Multiple queues can be used for different task types or priorities, allowing for better organization and management.
Implementation Details:
-
PHP AMQP Library: You'll need a PHP AMQP library (like
php-amqplib
) to interact with RabbitMQ from your Swoole workers. -
Swoole's
process
andcoroutine
features: Swoole'sprocess
allows creating multiple worker processes, whilecoroutine
enables asynchronous operations within each worker, preventing blocking and maximizing throughput. - Serialization: Tasks should be serialized (e.g., using JSON) before being published to RabbitMQ and deserialized by the workers.
- Error Handling: Implement robust error handling within the Swoole workers to catch exceptions and handle failed tasks appropriately (e.g., moving them to a dead-letter queue).
- Queue Management: Configure RabbitMQ queues and exchanges appropriately (e.g., setting durability, prefetch count, and using appropriate routing keys).
A basic example would involve a publisher sending messages to a queue, and several Swoole workers consuming messages from that queue, processing the tasks, and acknowledging message consumption to RabbitMQ.
What are the key advantages of using Swoole and RabbitMQ together for a distributed task queue?
Key Advantages of Swoole and RabbitMQ Combination
The combination of Swoole and RabbitMQ offers several key advantages for building a distributed task queue:
- High Performance: Swoole's asynchronous nature and event-driven architecture significantly improve performance compared to traditional synchronous PHP applications. RabbitMQ is also known for its high throughput and reliability. This combination allows for processing a large number of tasks concurrently.
- Scalability: Both Swoole and RabbitMQ are highly scalable. You can easily add more Swoole worker processes to handle increasing workloads, and RabbitMQ can be clustered for high availability and increased capacity.
- Reliability: RabbitMQ ensures message persistence and delivery guarantees, preventing task loss even in case of worker failures. Properly configured, the system can achieve high reliability.
- Decoupling: The message queue acts as a decoupling layer between the task publisher and the workers. This allows independent scaling and evolution of both components without affecting each other.
- Fault Tolerance: If a Swoole worker crashes, RabbitMQ retains the unprocessed tasks, allowing other workers to pick them up. This enhances the overall system resilience.
- Flexibility: RabbitMQ's features like message routing, exchanges, and queues offer flexibility in managing different task types and priorities.
How can I handle failures and ensure reliability in a distributed task queue built with Swoole and RabbitMQ?
Handling Failures and Ensuring Reliability
Reliability in a distributed task queue is crucial. Here's how to handle failures and ensure reliability when using Swoole and RabbitMQ:
- RabbitMQ's Durability: Configure RabbitMQ queues and exchanges to be durable. This ensures messages are persisted to disk, preventing data loss even if the RabbitMQ server restarts.
- Message Acknowledgements: Swoole workers should acknowledge messages only after successful task completion. If a worker crashes before acknowledging, RabbitMQ will redeliver the message to another worker. Use negative acknowledgements to explicitly reject messages in case of irrecoverable errors.
- Dead-Letter Queues (DLQs): Configure RabbitMQ to use DLQs. Messages that fail processing multiple times can be moved to a DLQ for later investigation and manual intervention.
- Retry Mechanisms: Implement retry logic within Swoole workers. If a task fails, retry it after a short delay, potentially with exponential backoff to avoid overwhelming the system.
- Monitoring and Alerting: Monitor both Swoole and RabbitMQ for errors and performance issues. Set up alerting mechanisms to notify you of critical problems.
- Transaction Management: For critical tasks, consider using RabbitMQ transactions to ensure atomicity – either all actions within a transaction succeed, or none do.
- Worker Health Checks: Implement health checks within Swoole workers to detect and automatically restart failing workers.
- Error Logging: Thorough logging of errors and exceptions in both Swoole and RabbitMQ is vital for debugging and troubleshooting.
What are the best practices for scaling a Swoole and RabbitMQ based distributed task queue system?
Best Practices for Scaling
Scaling a Swoole and RabbitMQ based system involves scaling both components independently:
- Scaling Swoole Workers: Increase the number of Swoole worker processes to handle increased workload. Monitor CPU and memory usage to determine the optimal number of workers. Consider using a process manager like Supervisor to manage and restart workers.
- Scaling RabbitMQ: For increased throughput and availability, cluster RabbitMQ servers. This distributes the workload across multiple servers and provides redundancy.
- Queue Management: Use multiple queues for different task types or priorities to improve throughput and prevent bottlenecks.
- Horizontal Scaling: Distribute tasks across multiple instances of your Swoole application. This requires a load balancer to distribute incoming tasks across the instances.
- Message Size Optimization: Keep message sizes as small as possible to reduce network overhead and improve throughput.
- Efficient Task Processing: Optimize the task processing logic within Swoole workers to minimize processing time.
- Database Scaling: If your tasks involve database interactions, ensure your database is also scaled appropriately. Consider using connection pooling to efficiently manage database connections.
- Caching: Utilize caching mechanisms (e.g., Redis) to reduce database load and improve response times.
- Monitoring and Performance Tuning: Continuously monitor the performance of both Swoole and RabbitMQ. Use profiling tools to identify bottlenecks and optimize your application. Regularly review queue lengths and worker performance metrics.
By following these best practices, you can build a highly scalable and reliable distributed task queue system using Swoole and RabbitMQ. Remember that thorough testing and monitoring are essential for ensuring the system's stability and performance under various load conditions.
The above is the detailed content of How to Build a Distributed Task Queue System with Swoole and RabbitMQ?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 English version
Recommended: Win version, supports code prompts!

SublimeText3 Chinese version
Chinese version, very easy to use
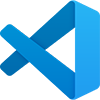
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software