


What is template metaprogramming in C and how can I use it for compile-time computations?
What is template metaprogramming in C and how can I use it for compile-time computations?
Template metaprogramming (TMP) in C is a powerful technique that allows you to perform computations during the compilation process, rather than at runtime. This is achieved by leveraging C 's template system to generate code at compile time. Instead of writing code that executes at runtime, you write code that the compiler executes to generate specialized code for different types. This generated code is then used during the program's execution.
The core idea is to use templates not just for generic programming (writing code that works with multiple types), but also for controlling the structure and behavior of the code itself at compile time. This is done through template recursion, template specialization, and other template features.
How to use it for compile-time computations:
Let's consider a simple example: calculating the factorial of a number at compile time. We can achieve this using template recursion:
template <int N> struct Factorial { static const int value = N * Factorial<N - 1>::value; }; template <> struct Factorial<0> { static const int value = 1; }; int main() { constexpr int factorial_5 = Factorial<5>::value; // Computed at compile time // ... use factorial_5 ... return 0; }
Here, Factorial<n></n>
recursively calculates the factorial. The base case (Factorial
) stops the recursion. The constexpr
keyword ensures that the computation happens at compile time. The compiler generates the code for factorial_5
(which will be 120) during compilation. This avoids the runtime overhead of calculating the factorial. More complex computations can be achieved using similar techniques, combining template recursion with other template features like partial specialization.
What are the advantages and disadvantages of using template metaprogramming in C ?
Advantages:
- Compile-time computations: This is the primary advantage. Computations are performed during compilation, eliminating runtime overhead and potentially improving performance.
- Code generation: TMP allows for the generation of highly optimized code tailored to specific types and situations. This can lead to significant performance improvements compared to runtime polymorphism.
- Increased type safety: Many errors that would occur at runtime in regular code can be caught at compile time using TMP. This improves the overall robustness of the code.
- Improved code readability (sometimes): For certain algorithms, expressing them using TMP can lead to more concise and elegant code, compared to equivalent runtime implementations.
Disadvantages:
- Increased compilation time: Compile times can significantly increase, especially for complex TMP implementations. This can severely hinder development productivity.
- Difficult to debug: Debugging TMP code can be challenging, as the actual code execution happens during compilation, and traditional debugging tools may not be as effective. Error messages can also be cryptic and hard to interpret.
- Complexity: TMP can be conceptually complex, requiring a deep understanding of C templates and metaprogramming techniques. It's not suitable for all situations and can make the code harder to maintain and understand for less experienced developers.
- Compiler limitations: The capabilities of TMP are dependent on the compiler's support for template metaprogramming features. Some compilers may have limitations or handle TMP differently, leading to portability issues.
Can template metaprogramming improve the performance of my C code, and if so, how?
Yes, template metaprogramming can significantly improve the performance of C code in certain situations. The primary way it achieves this is by moving computations from runtime to compile time.
How it improves performance:
- Eliminating runtime overhead: By pre-calculating values or generating specialized code at compile time, TMP eliminates the need for these calculations during program execution. This can result in substantial performance gains, particularly for computationally intensive operations performed repeatedly.
- Code specialization: TMP allows the generation of highly optimized code tailored to specific types. This can lead to better utilization of CPU instructions and data structures.
- Static polymorphism: TMP can replace runtime polymorphism (e.g., virtual functions) with compile-time polymorphism, eliminating the overhead associated with virtual function calls. This is particularly beneficial in performance-critical sections of the code.
However, it's crucial to note that TMP doesn't always improve performance. The overhead of increased compilation time and the complexity of the generated code can sometimes outweigh the performance benefits. TMP should be used strategically, where the performance gains justify the added complexity.
How does template metaprogramming differ from runtime computation in C , and when should I choose one over the other?
The fundamental difference lies in when the computation occurs:
- Template metaprogramming: Computations are performed by the compiler during the compilation phase. The results are baked into the generated code.
- Runtime computation: Computations are performed by the CPU during the program's execution.
When to choose TMP:
- Performance-critical sections: When a computation is performed repeatedly and the runtime overhead is significant, TMP can provide substantial performance improvements.
- Compile-time constants: When values are known at compile time, calculating them using TMP can eliminate runtime calculations.
- Code generation: When you need to generate specialized code based on types or other compile-time information, TMP is the ideal solution.
- Type safety: When compile-time error checking is crucial, TMP can help detect errors early in the development process.
When to choose runtime computation:
- Dynamic data: When the data involved in the computation is only known at runtime, TMP is not applicable.
- Complexity and maintainability: If the computation is complex and TMP would significantly increase the compilation time or make the code harder to maintain, runtime computation is preferable.
- Flexibility: Runtime computation offers greater flexibility, as the code can adapt to changing conditions during program execution.
- Debugging ease: Runtime computations are generally much easier to debug than template metaprogramming.
In summary, the choice between TMP and runtime computation is a trade-off between compile-time efficiency and development complexity. Use TMP when the performance benefits significantly outweigh the increased development complexity and compilation time. Otherwise, stick to runtime computation for simplicity and maintainability.
The above is the detailed content of What is template metaprogramming in C and how can I use it for compile-time computations?. For more information, please follow other related articles on the PHP Chinese website!
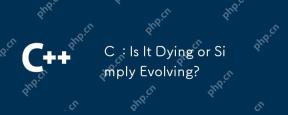
C isnotdying;it'sevolving.1)C remainsrelevantduetoitsversatilityandefficiencyinperformance-criticalapplications.2)Thelanguageiscontinuouslyupdated,withC 20introducingfeatureslikemodulesandcoroutinestoimproveusabilityandperformance.3)Despitechallen
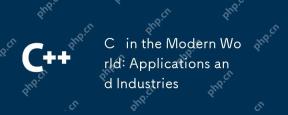
C is widely used and important in the modern world. 1) In game development, C is widely used for its high performance and polymorphism, such as UnrealEngine and Unity. 2) In financial trading systems, C's low latency and high throughput make it the first choice, suitable for high-frequency trading and real-time data analysis.
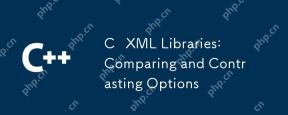
There are four commonly used XML libraries in C: TinyXML-2, PugiXML, Xerces-C, and RapidXML. 1.TinyXML-2 is suitable for environments with limited resources, lightweight but limited functions. 2. PugiXML is fast and supports XPath query, suitable for complex XML structures. 3.Xerces-C is powerful, supports DOM and SAX resolution, and is suitable for complex processing. 4. RapidXML focuses on performance and parses extremely fast, but does not support XPath queries.
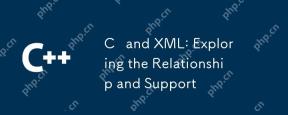
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
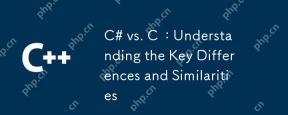
The main differences between C# and C are syntax, performance and application scenarios. 1) The C# syntax is more concise, supports garbage collection, and is suitable for .NET framework development. 2) C has higher performance and requires manual memory management, which is often used in system programming and game development.
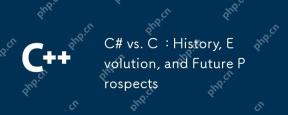
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
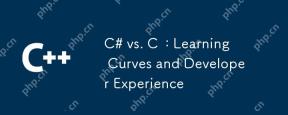
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
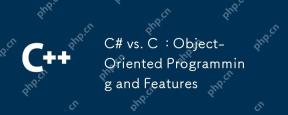
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
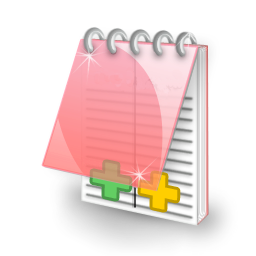
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
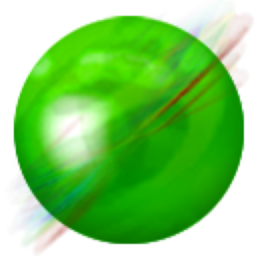
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
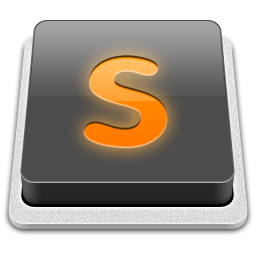
SublimeText3 Mac version
God-level code editing software (SublimeText3)