


What are the best practices for memory management in C (smart pointers, RAII)?
What are the best practices for memory management in C (smart pointers, RAII)?
Best Practices for Memory Management in C
Effective memory management is crucial for writing robust and efficient C applications. The core principles revolve around two key concepts: smart pointers and Resource Acquisition Is Initialization (RAII).
Smart Pointers: Smart pointers are classes that act like pointers but automatically manage the memory lifecycle of the objects they point to. They encapsulate the delete
operation, preventing memory leaks. The standard library provides several smart pointer types:
-
std::unique_ptr
: Represents exclusive ownership of an object. Only oneunique_ptr
can point to a given object at a time. It automatically deletes the object when it goes out of scope. It's ideal for situations where only one owner is needed. It doesn't support copying, only moving. -
std::shared_ptr
: Represents shared ownership of an object. Multipleshared_ptr
objects can point to the same object. The object is deleted only when the lastshared_ptr
pointing to it goes out of scope. It uses reference counting to track ownership. It's suitable for scenarios where multiple parts of your code need to access the same object. -
std::weak_ptr
: A non-owning pointer that doesn't affect the object's lifetime. It's used to break circular dependencies betweenshared_ptr
objects and to check if a shared object still exists. You need to explicitly calllock()
to get ashared_ptr
from aweak_ptr
, which will return a null pointer if the object has been deleted.
RAII (Resource Acquisition Is Initialization): This principle dictates that resources (memory, files, network connections, etc.) should be acquired in the constructor of a class and released in its destructor. This ensures that resources are automatically released even in the event of exceptions. Smart pointers are a prime example of RAII in action. By using smart pointers, you ensure that memory is automatically managed without manual delete
calls, significantly reducing the risk of memory leaks. Applying RAII to other resources follows the same principle: acquire in the constructor, release in the destructor.
By consistently applying smart pointers and RAII, you drastically improve the reliability and maintainability of your C code, reducing the likelihood of memory-related bugs.
How can I avoid memory leaks and dangling pointers when using smart pointers in C ?
Avoiding Memory Leaks and Dangling Pointers with Smart Pointers
Memory leaks and dangling pointers are common issues in C , but smart pointers significantly mitigate these risks. However, careful usage is still required:
Memory Leaks: Memory leaks occur when dynamically allocated memory is not freed. With smart pointers, memory leaks are rare but can still happen in specific situations:
-
Circular Dependencies: If two or more
shared_ptr
objects point to each other, creating a circular dependency, neither object will be deleted even when they are no longer needed. This is wherestd::weak_ptr
comes into play.weak_ptr
breaks the cycle. -
Raw Pointers within Smart Pointers: If you create a
shared_ptr
from a raw pointer, ensure that the raw pointer itself doesn't continue to be used after theshared_ptr
is created. Otherwise, you might inadvertently extend the lifespan of the object beyond what's intended.
Dangling Pointers: A dangling pointer points to memory that has already been freed. Smart pointers generally prevent dangling pointers because they automatically manage the deletion of the pointed-to object. However, problems can arise if:
-
Using
reset()
improperly: Thereset()
method ofunique_ptr
andshared_ptr
releases the object. If you have another pointer to the same object, usingreset()
can lead to a dangling pointer if that other pointer isn't also reset. -
Incorrect use of
get()
: Theget()
method of smart pointers returns a raw pointer. If you use this raw pointer after the smart pointer goes out of scope, you create a dangling pointer. Minimize the use ofget()
, and if you must use it, ensure the raw pointer is only used within the smart pointer's lifetime.
By adhering to these guidelines and using smart pointers correctly, you can greatly reduce the risk of memory leaks and dangling pointers in your C applications.
What are the common pitfalls to watch out for when implementing Resource Acquisition Is Initialization (RAII) in C ?
Common Pitfalls of RAII Implementation
While RAII is a powerful technique, several pitfalls can arise during its implementation:
-
Exceptions during resource acquisition: If an exception occurs during the constructor (resource acquisition), the destructor might not be called, leading to resource leaks. Consider using RAII for smaller, self-contained operations to minimize the risk. If complex resource acquisition is necessary, consider using exception handling techniques to ensure proper resource release, such as nested RAII objects or
std::unique_ptr
with custom deleters. -
Ignoring exceptions in destructors: Destructors should generally avoid throwing exceptions. If a destructor throws an exception, it can lead to unpredictable behavior, particularly when used in complex scenarios involving multiple objects. Handle exceptions gracefully or use techniques like
std::uncaught_exception
to check for pre-existing exceptions to avoid masking errors. - Incorrect copy semantics: If your class manages resources, you need to carefully consider copy semantics. A simple copy constructor or assignment operator might lead to double-deletion errors or other issues. Consider using the copy-and-swap idiom or explicitly deleting the copy constructor and assignment operator if copying is not allowed.
- Resource leaks in complex scenarios: When managing multiple resources or interacting with external libraries, ensuring proper resource release can become complex. Use smaller, well-defined RAII classes to manage individual resources and compose them to manage complex scenarios.
- Not using RAII consistently: The power of RAII comes from its consistent application. Inconsistent use can lead to a mix of manual and automatic resource management, increasing the risk of errors.
By paying attention to these pitfalls and implementing robust exception handling, you can avoid many of the common issues associated with RAII.
What are the performance implications of different smart pointer types in C and when should I choose one over another?
Performance Implications of Smart Pointer Types
The performance of different smart pointer types varies, influencing the choice based on specific needs:
-
unique_ptr
: Generally has the lowest overhead among the three standard smart pointers because it only involves a single pointer. It avoids the cost of reference counting, making it the most performant option when only one owner is required. -
shared_ptr
: Involves a higher overhead due to reference counting. Eachshared_ptr
object maintains a control block that tracks the number of shared pointers pointing to the managed object. This increases memory consumption and incurs some performance penalty compared tounique_ptr
. However, it's crucial for shared ownership scenarios. Consider usingshared_ptr
when multiple parts of your code need to access the same object. -
weak_ptr
: Has minimal overhead because it doesn't participate in reference counting. It primarily serves as a way to check for object existence without affecting its lifetime. It only adds a small amount of overhead compared to raw pointers.
Choosing the Right Smart Pointer:
-
Use
unique_ptr
when: You need exclusive ownership of an object and only one part of your code needs to access it. This is the default choice for most situations unless shared ownership is explicitly required. It offers the best performance. -
Use
shared_ptr
when: Multiple parts of your code need to share ownership of an object. It handles the complexity of reference counting, ensuring proper memory management even with multiple owners. Be mindful of potential performance overhead and the possibility of circular dependencies. -
Use
weak_ptr
when: You need to observe the existence of an object without affecting its lifetime, typically to break circular dependencies betweenshared_ptr
s or to safely access a potentially deleted object.
The performance difference between smart pointers can be negligible in many cases. However, in performance-critical sections of your code, unique_ptr
generally provides the best performance. Choose the smart pointer type that best suits your ownership and access requirements, prioritizing correctness and maintainability over minor performance differences unless performance is a truly critical constraint.
The above is the detailed content of What are the best practices for memory management in C (smart pointers, RAII)?. For more information, please follow other related articles on the PHP Chinese website!
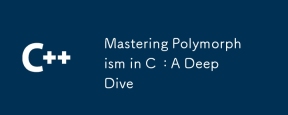
Mastering polymorphisms in C can significantly improve code flexibility and maintainability. 1) Polymorphism allows different types of objects to be treated as objects of the same base type. 2) Implement runtime polymorphism through inheritance and virtual functions. 3) Polymorphism supports code extension without modifying existing classes. 4) Using CRTP to implement compile-time polymorphism can improve performance. 5) Smart pointers help resource management. 6) The base class should have a virtual destructor. 7) Performance optimization requires code analysis first.
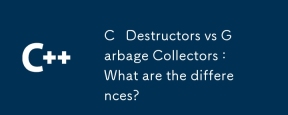
C destructorsprovideprecisecontroloverresourcemanagement,whilegarbagecollectorsautomatememorymanagementbutintroduceunpredictability.C destructors:1)Allowcustomcleanupactionswhenobjectsaredestroyed,2)Releaseresourcesimmediatelywhenobjectsgooutofscop
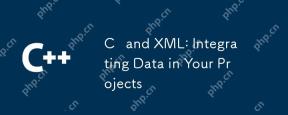
Integrating XML in a C project can be achieved through the following steps: 1) parse and generate XML files using pugixml or TinyXML library, 2) select DOM or SAX methods for parsing, 3) handle nested nodes and multi-level properties, 4) optimize performance using debugging techniques and best practices.
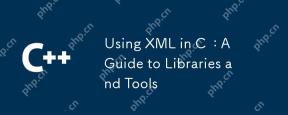
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
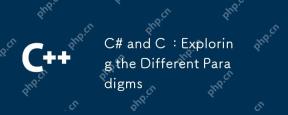
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
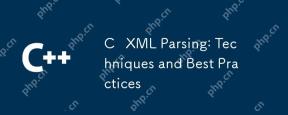
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
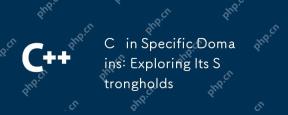
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
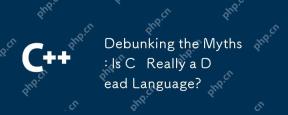
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
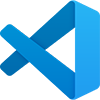
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Notepad++7.3.1
Easy-to-use and free code editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
