How to Use Vuex Plugins to Extend Its Functionality?
Vuex plugins offer a powerful mechanism to extend the core functionality of Vuex without modifying its internal structure. They provide a clean and organized way to add features like logging, persistence, or custom middleware. To use a plugin, you simply pass it to the plugins
option when creating your Vuex store.
Let's illustrate with a simple example of a plugin that logs all mutations:
// myPlugin.js export default function myPlugin (store) { store.subscribe((mutation, state) => { console.log('mutation:', mutation.type) console.log('payload:', mutation.payload) console.log('state:', state) }) } // main.js import Vue from 'vue' import Vuex from 'vuex' import myPlugin from './myPlugin' Vue.use(Vuex) const store = new Vuex.Store({ state: { count: 0 }, mutations: { increment (state) { state.count } }, plugins: [myPlugin] })
In this example, myPlugin.js
exports a function that takes the store instance as an argument. Inside this function, we use store.subscribe
to listen for mutations and log relevant information to the console. In main.js
, we import the plugin and add it to the plugins
array when creating the store. Now, every time a mutation is committed, the console will display details about the mutation and the current state. This is a fundamental pattern for creating and using Vuex plugins. More complex plugins can incorporate asynchronous operations, interact with external services, or implement more sophisticated logic within the subscribe
function or other store methods provided by the store
object.
What Are Some Common Use Cases for Vuex Plugins?
Vuex plugins are invaluable for several common scenarios:
- Logging and Debugging: As demonstrated above, plugins are ideal for logging mutations, actions, or state changes. This is crucial during development and debugging to track data flow and identify potential issues.
- State Persistence: Plugins can seamlessly integrate with local storage (localStorage or sessionStorage) or other persistence mechanisms (like IndexedDB or a backend API) to automatically save and restore the application's state. This allows for preserving user settings or application data across sessions.
- Middleware: Plugins can act as middleware, intercepting actions or mutations before they are processed. This enables features like authorization checks, request throttling, or optimistic updates.
- External API Interaction: Plugins can handle interactions with external APIs, fetching data and updating the store accordingly. This keeps your store logic cleaner and more focused on state management.
- Custom Error Handling: A plugin can centralize error handling logic, providing a consistent approach for managing errors throughout the application. This can include logging errors, displaying user-friendly messages, or implementing retry mechanisms.
- Code Splitting: For larger applications, plugins can facilitate code splitting, improving initial load times by loading only the necessary plugin functionality when needed.
Can I Create My Own Custom Vuex Plugin?
Absolutely! Creating custom Vuex plugins is straightforward. The key is to understand the plugin's structure: a function that receives the store instance as an argument. Within this function, you can leverage the various methods provided by the store object (like subscribe
, dispatch
, commit
, replaceState
, watch
, registerModule
, unregisterModule
) to integrate your custom logic.
Remember that a well-designed plugin should be modular, reusable, and have minimal dependencies to ensure maintainability and ease of integration into different projects. Consider using clear and descriptive names for your plugins and their exported functions.
How Do Vuex Plugins Interact With Other Parts of a Vue Application?
Vuex plugins primarily interact with other parts of a Vue application through the Vuex store itself. They don't directly interact with Vue components or other modules in a way that bypasses the store. Instead, plugins enhance the store's capabilities, allowing you to extend its functionality in ways that benefit all parts of your application that use the store.
For instance, a plugin that persists the store's state will automatically save and load the state without requiring any explicit interaction from components. Similarly, a plugin that adds logging will automatically log events without requiring components to explicitly call logging functions. The interaction happens indirectly, through the store's events and methods that the plugin accesses. This maintains a clear separation of concerns, making your application more organized and maintainable. Components still interact with the store using $store.dispatch
, $store.commit
, $store.state
, etc., but the plugins augment the underlying behavior of these actions.
The above is the detailed content of How do I use Vuex plugins to extend its functionality?. For more information, please follow other related articles on the PHP Chinese website!

Vue.js is a progressive JavaScript framework released by You Yuxi in 2014 to build a user interface. Its core advantages include: 1. Responsive data binding, automatic update view of data changes; 2. Component development, the UI can be split into independent and reusable components.

Netflix uses React as its front-end framework. 1) React's componentized development model and strong ecosystem are the main reasons why Netflix chose it. 2) Through componentization, Netflix splits complex interfaces into manageable chunks such as video players, recommendation lists and user comments. 3) React's virtual DOM and component life cycle optimizes rendering efficiency and user interaction management.

Netflix's choice in front-end technology mainly focuses on three aspects: performance optimization, scalability and user experience. 1. Performance optimization: Netflix chose React as the main framework and developed tools such as SpeedCurve and Boomerang to monitor and optimize the user experience. 2. Scalability: They adopt a micro front-end architecture, splitting applications into independent modules, improving development efficiency and system scalability. 3. User experience: Netflix uses the Material-UI component library to continuously optimize the interface through A/B testing and user feedback to ensure consistency and aesthetics.

Netflixusesacustomframeworkcalled"Gibbon"builtonReact,notReactorVuedirectly.1)TeamExperience:Choosebasedonfamiliarity.2)ProjectComplexity:Vueforsimplerprojects,Reactforcomplexones.3)CustomizationNeeds:Reactoffersmoreflexibility.4)Ecosystema

Netflix mainly considers performance, scalability, development efficiency, ecosystem, technical debt and maintenance costs in framework selection. 1. Performance and scalability: Java and SpringBoot are selected to efficiently process massive data and high concurrent requests. 2. Development efficiency and ecosystem: Use React to improve front-end development efficiency and utilize its rich ecosystem. 3. Technical debt and maintenance costs: Choose Node.js to build microservices to reduce maintenance costs and technical debt.

Netflix mainly uses React as the front-end framework, supplemented by Vue for specific functions. 1) React's componentization and virtual DOM improve the performance and development efficiency of Netflix applications. 2) Vue is used in Netflix's internal tools and small projects, and its flexibility and ease of use are key.

Vue.js is a progressive JavaScript framework suitable for building complex user interfaces. 1) Its core concepts include responsive data, componentization and virtual DOM. 2) In practical applications, it can be demonstrated by building Todo applications and integrating VueRouter. 3) When debugging, it is recommended to use VueDevtools and console.log. 4) Performance optimization can be achieved through v-if/v-show, list rendering optimization, asynchronous loading of components, etc.

Vue.js is suitable for small to medium-sized projects, while React is more suitable for large and complex applications. 1. Vue.js' responsive system automatically updates the DOM through dependency tracking, making it easy to manage data changes. 2.React adopts a one-way data flow, and data flows from the parent component to the child component, providing a clear data flow and an easy-to-debug structure.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
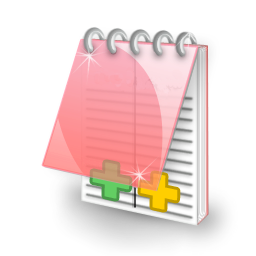
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
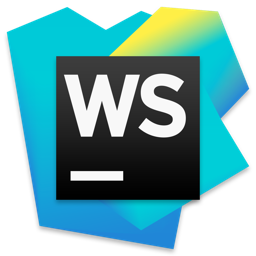
WebStorm Mac version
Useful JavaScript development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!

Zend Studio 13.0.1
Powerful PHP integrated development environment