How do I use triggers in SQL to automate actions in response to data changes?
This article explains how to use SQL triggers to automate database actions. It details trigger creation, body specification (including examples in PostgreSQL), and best practices for efficiency and error handling. The article also highlights using
How to Use Triggers in SQL to Automate Actions in Response to Data Changes?
SQL triggers are procedural code that automatically execute in response to specific events on a particular table or view in a database. These events can be INSERT, UPDATE, or DELETE operations. Triggers allow you to automate actions, ensuring data consistency and integrity without requiring manual intervention. Here's a breakdown of how to use them:
1. Defining the Trigger: You begin by creating the trigger using a CREATE TRIGGER statement. This statement specifies the trigger's name, the table or view it's associated with, the event that activates it (INSERT, UPDATE, DELETE, or a combination), and the timing (BEFORE or AFTER the event).
2. Specifying the Trigger Body: The core of the trigger is its body, which contains the SQL code to be executed. This code can perform various actions, such as:
- Performing calculations: Updating other tables based on changes in the triggered table.
- Enforcing constraints: Checking for data validity and rejecting invalid updates.
- Auditing changes: Logging changes to a separate audit table.
- Sending notifications: Triggering emails or other alerts based on data modifications.
3. Example (PostgreSQL):
Let's say you want to automatically update a "last_updated" timestamp whenever a row in a "products" table is updated. Here's how you might create a trigger in PostgreSQL:
CREATE OR REPLACE FUNCTION update_last_updated() RETURNS TRIGGER AS $$ BEGIN NEW.last_updated = NOW(); RETURN NEW; END; $$ LANGUAGE plpgsql; CREATE TRIGGER update_product_timestamp BEFORE UPDATE ON products FOR EACH ROW EXECUTE PROCEDURE update_last_updated();
This code first creates a function update_last_updated()
that updates the last_updated
column. Then, it creates a trigger update_product_timestamp
that executes this function before each UPDATE operation on the products
table.
4. Different Database Systems: The syntax for creating triggers varies slightly across different database systems (MySQL, SQL Server, Oracle, etc.). Consult your database system's documentation for the specific syntax.
Best Practices for Designing and Implementing Efficient SQL Triggers
Efficient SQL trigger design is crucial for database performance. Here are some best practices:
- Minimize Trigger Complexity: Keep the trigger code concise and focused. Avoid complex logic or lengthy calculations within the trigger body. Large, complex triggers can significantly impact database performance. Break down complex tasks into smaller, modular procedures called by the trigger.
- Use the Appropriate Timing: Choose between BEFORE and AFTER triggers carefully. BEFORE triggers allow you to modify the data before it's inserted or updated, while AFTER triggers act after the data change has already occurred. Choose the timing that best suits your needs and minimizes the risk of cascading effects.
- Index Relevant Columns: Ensure that the columns used in the trigger's WHERE clause and the tables it accesses are properly indexed. This can dramatically improve the trigger's performance, especially when dealing with large datasets.
- Avoid Recursive Triggers: Recursive triggers (a trigger calling itself) can lead to infinite loops and system crashes. Design your triggers to avoid such scenarios.
- Use Stored Procedures: Encapsulate complex logic within stored procedures and call these procedures from the trigger. This promotes code reusability and maintainability.
- Test Thoroughly: Rigorously test your triggers to ensure they function correctly and don't introduce unexpected behavior or performance issues.
- Error Handling: Include proper error handling mechanisms within your triggers to gracefully handle exceptions and prevent unexpected failures. Log errors for debugging purposes.
Can SQL Triggers Be Used to Enforce Data Integrity and Business Rules Within a Database?
Yes, SQL triggers are extremely valuable for enforcing data integrity and business rules. They provide a powerful mechanism to ensure that data meets specific constraints and adheres to predefined rules before it's stored in the database. Here's how:
- Data Validation: Triggers can validate data before it's inserted or updated. They can check for data types, ranges, and relationships between different tables. If data doesn't meet the specified criteria, the trigger can reject the change.
- Referential Integrity: Triggers can enforce referential integrity by ensuring that foreign key constraints are satisfied. For example, a trigger can prevent the deletion of a record in a parent table if there are related records in a child table.
- Business Rule Enforcement: Triggers can enforce complex business rules that are difficult or impossible to express through standard constraints. For example, a trigger might prevent an order from being processed if the customer's credit limit is exceeded.
- Auditing: Triggers can be used to log changes to the database, providing an audit trail of data modifications. This is crucial for tracking data changes and ensuring accountability.
How Do I Troubleshoot and Debug Issues with SQL Triggers That Aren't Functioning Correctly?
Debugging SQL triggers can be challenging. Here's a systematic approach:
- Check Trigger Syntax: Ensure the trigger's syntax is correct according to your database system's documentation. Even small errors can prevent the trigger from functioning.
- Examine Trigger Log: Many database systems provide logging mechanisms that record trigger executions. Review the logs to identify errors or unexpected behavior.
-
Use PRINT or RAISERROR Statements: (Depending on your database system) Insert
PRINT
(SQL Server) orRAISERROR
(SQL Server) statements into your trigger code to output intermediate values and track the trigger's execution flow. This helps pinpoint the source of the problem. - Step Through the Code: If possible, use a debugger to step through the trigger's code line by line. This allows you to inspect variables and understand the execution path.
- Simplify the Trigger: If the trigger is complex, try simplifying it to isolate the problematic part. This makes it easier to identify and fix the issue.
- Check for Conflicts: Multiple triggers on the same table can sometimes conflict with each other. Check for potential conflicts and adjust the trigger's order of execution if necessary.
- Review Database Constraints: Ensure that other constraints (e.g., CHECK constraints, UNIQUE constraints) don't conflict with the trigger's logic.
- Test with Smaller Datasets: Test the trigger with smaller, simpler datasets to isolate the problem. If the trigger works correctly with small datasets but fails with larger ones, it may indicate a performance issue.
The above is the detailed content of How do I use triggers in SQL to automate actions in response to data changes?. For more information, please follow other related articles on the PHP Chinese website!

SQL is suitable for beginners because it is simple in syntax, powerful in function, and widely used in database systems. 1.SQL is used to manage relational databases and organize data through tables. 2. Basic operations include creating, inserting, querying, updating and deleting data. 3. Advanced usage such as JOIN, subquery and window functions enhance data analysis capabilities. 4. Common errors include syntax, logic and performance issues, which can be solved through inspection and optimization. 5. Performance optimization suggestions include using indexes, avoiding SELECT*, using EXPLAIN to analyze queries, normalizing databases, and improving code readability.

In practical applications, SQL is mainly used for data query and analysis, data integration and reporting, data cleaning and preprocessing, advanced usage and optimization, as well as handling complex queries and avoiding common errors. 1) Data query and analysis can be used to find the most sales product; 2) Data integration and reporting generate customer purchase reports through JOIN operations; 3) Data cleaning and preprocessing can delete abnormal age records; 4) Advanced usage and optimization include using window functions and creating indexes; 5) CTE and JOIN can be used to handle complex queries to avoid common errors such as SQL injection.

SQL is a standard language for managing relational databases, while MySQL is a specific database management system. SQL provides a unified syntax and is suitable for a variety of databases; MySQL is lightweight and open source, with stable performance but has bottlenecks in big data processing.

The SQL learning curve is steep, but it can be mastered through practice and understanding the core concepts. 1. Basic operations include SELECT, INSERT, UPDATE, DELETE. 2. Query execution is divided into three steps: analysis, optimization and execution. 3. Basic usage is such as querying employee information, and advanced usage is such as using JOIN connection table. 4. Common errors include not using alias and SQL injection, and parameterized query is required to prevent it. 5. Performance optimization is achieved by selecting necessary columns and maintaining code readability.

SQL commands are divided into five categories in MySQL: DQL, DDL, DML, DCL and TCL, and are used to define, operate and control database data. MySQL processes SQL commands through lexical analysis, syntax analysis, optimization and execution, and uses index and query optimizers to improve performance. Examples of usage include SELECT for data queries and JOIN for multi-table operations. Common errors include syntax, logic, and performance issues, and optimization strategies include using indexes, optimizing queries, and choosing the right storage engine.

Advanced query skills in SQL include subqueries, window functions, CTEs and complex JOINs, which can handle complex data analysis requirements. 1) Subquery is used to find the employees with the highest salary in each department. 2) Window functions and CTE are used to analyze employee salary growth trends. 3) Performance optimization strategies include index optimization, query rewriting and using partition tables.

MySQL is an open source relational database management system that provides standard SQL functions and extensions. 1) MySQL supports standard SQL operations such as CREATE, INSERT, UPDATE, DELETE, and extends the LIMIT clause. 2) It uses storage engines such as InnoDB and MyISAM, which are suitable for different scenarios. 3) Users can efficiently use MySQL through advanced functions such as creating tables, inserting data, and using stored procedures.

SQLmakesdatamanagementaccessibletoallbyprovidingasimpleyetpowerfultoolsetforqueryingandmanagingdatabases.1)Itworkswithrelationaldatabases,allowinguserstospecifywhattheywanttodowiththedata.2)SQL'sstrengthliesinfiltering,sorting,andjoiningdataacrosstab


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
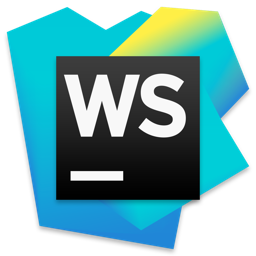
WebStorm Mac version
Useful JavaScript development tools
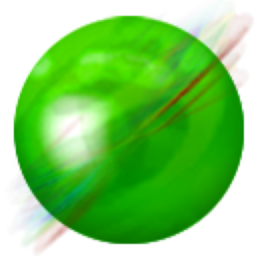
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.