This article explains using Redis hashes for efficient structured data storage and retrieval. It details commands like HSET, HGET, and HMGET, and best practices for large datasets including data modeling, indexing, and batch operations. The articl
How to Use Redis Hashes for Storing and Retrieving Structured Data
Redis hashes provide a convenient way to store structured data within a single key. A hash is essentially a key-value store where the key is a string (the field name) and the value can be any of Redis' supported data types (strings, numbers, etc.). This allows you to represent complex objects efficiently.
To store data, you use the HSET
command. For example, to store information about a product:
HSET product:123 name "Awesome Widget" price 19.99 description "A fantastic widget!"
This creates a hash with the key product:123
. It sets the fields name
, price
, and description
with their respective values.
Retrieving data is equally straightforward. HGET
retrieves a single field:
HGET product:123 price
This would return 19.99
. HGETALL
retrieves all fields and values:
HGETALL product:123
This would return all the data associated with product:123
. You can also use HMGET
to retrieve multiple fields at once:
HMGET product:123 name price
This improves efficiency compared to multiple HGET
calls. Incrementing numeric values is also easy with HINCRBY
:
HINCRBY product:123 quantity 1
Best Practices for Efficiently Using Redis Hashes with Large Datasets
Efficiently using Redis hashes with large datasets requires careful consideration. Here are some best practices:
- Data Modeling: Avoid excessively large hashes. If a hash becomes too large (many fields), consider breaking it down into smaller, more focused hashes or using other Redis data structures like JSON or sorted sets. Large hashes can lead to performance bottlenecks.
- Field Naming Conventions: Use consistent and descriptive field names to improve readability and maintainability.
- Indexing: While Redis hashes don't directly support indexing, you can use other Redis data structures (like sorted sets) in conjunction with hashes to create indexes for faster searching. For example, if you need to quickly find products by price, you could store product IDs in a sorted set ordered by price, with the product details stored in separate hashes.
-
Batch Operations: Use commands like
HMSET
(for setting multiple fields at once) andHMGET
(for getting multiple fields at once) to reduce the number of round trips to the Redis server. This significantly improves performance. -
Data Expiration: If data has a limited lifespan, use
EXPIRE
to set an expiration time for the hash key, preventing unnecessary data accumulation. - Redis Cluster: For extremely large datasets, consider using a Redis Cluster to distribute the data across multiple nodes, improving scalability and performance.
Using Redis Hashes for Implementing a User Profile System
Yes, Redis hashes are well-suited for implementing a user profile system. You can use a user ID as the key and store various profile attributes as fields within the hash.
For example:
<code>HSET user:1234 username "johndoe" email "john.doe@example.com" location "New York" last_login 1678886400</code>
Here, user:1234
is the key, and username
, email
, location
, and last_login
are fields. You can easily update individual fields using HSET
or HINCRBY
(for numeric fields like login count). Retrieving the entire profile is done with HGETALL user:1234
. This approach is efficient for accessing and updating individual profile attributes. For more complex scenarios, consider using JSON within the hash for nested data.
Handling Potential Conflicts or Collisions When Using Redis Hashes
Redis hashes themselves don't inherently have collisions in the sense of hash table collisions. The key is unique, and the fields within the hash are also unique within that key. However, collisions can arise from poor data modeling or naming conventions.
- Unique Key Generation: Ensure your keys (e.g., user IDs, product IDs) are globally unique to prevent overwriting data. Use UUIDs or other reliable unique identifiers if necessary.
- Careful Field Naming: Avoid ambiguous or overlapping field names within a single hash. Clearly defined field names prevent confusion and accidental data overwriting.
-
Atomic Operations: Redis provides atomic operations like
HSET
,HINCRBY
, etc., which guarantee that operations are performed without interruption, preventing race conditions and data corruption. Use these operations to ensure data consistency, especially in concurrent environments. -
Transactions: For more complex scenarios involving multiple operations on different keys, use Redis transactions (
MULTI
,EXEC
) to ensure atomicity across multiple commands. This helps maintain data integrity in situations where multiple clients might access and modify data concurrently.
The above is the detailed content of How do I use Redis hashes for storing and retrieving structured data?. For more information, please follow other related articles on the PHP Chinese website!
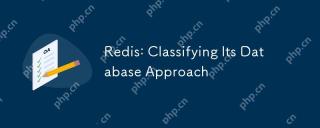
Redis's database methods include in-memory databases and key-value storage. 1) Redis stores data in memory, and reads and writes fast. 2) It uses key-value pairs to store data, supports complex data structures such as lists, collections, hash tables and ordered collections, suitable for caches and NoSQL databases.
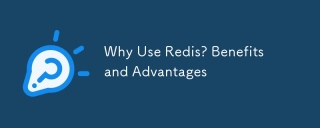
Redis is a powerful database solution because it provides fast performance, rich data structures, high availability and scalability, persistence capabilities, and a wide range of ecosystem support. 1) Extremely fast performance: Redis's data is stored in memory and has extremely fast read and write speeds, suitable for high concurrency and low latency applications. 2) Rich data structure: supports multiple data types, such as lists, collections, etc., which are suitable for a variety of scenarios. 3) High availability and scalability: supports master-slave replication and cluster mode to achieve high availability and horizontal scalability. 4) Persistence and data security: Data persistence is achieved through RDB and AOF to ensure data integrity and reliability. 5) Wide ecosystem and community support: with a huge ecosystem and active community,
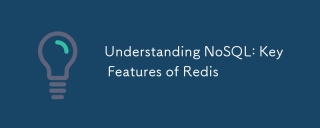
Key features of Redis include speed, flexibility and rich data structure support. 1) Speed: Redis is an in-memory database, and read and write operations are almost instantaneous, suitable for cache and session management. 2) Flexibility: Supports multiple data structures, such as strings, lists, collections, etc., which are suitable for complex data processing. 3) Data structure support: provides strings, lists, collections, hash tables, etc., which are suitable for different business needs.
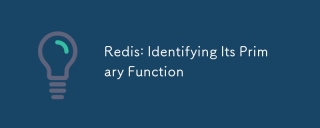
The core function of Redis is a high-performance in-memory data storage and processing system. 1) High-speed data access: Redis stores data in memory and provides microsecond-level read and write speed. 2) Rich data structure: supports strings, lists, collections, etc., and adapts to a variety of application scenarios. 3) Persistence: Persist data to disk through RDB and AOF. 4) Publish subscription: Can be used in message queues or real-time communication systems.

Redis supports a variety of data structures, including: 1. String, suitable for storing single-value data; 2. List, suitable for queues and stacks; 3. Set, used for storing non-duplicate data; 4. Ordered Set, suitable for ranking lists and priority queues; 5. Hash table, suitable for storing object or structured data.
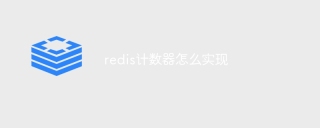
Redis counter is a mechanism that uses Redis key-value pair storage to implement counting operations, including the following steps: creating counter keys, increasing counts, decreasing counts, resetting counts, and obtaining counts. The advantages of Redis counters include fast speed, high concurrency, durability and simplicity and ease of use. It can be used in scenarios such as user access counting, real-time metric tracking, game scores and rankings, and order processing counting.

Use the Redis command line tool (redis-cli) to manage and operate Redis through the following steps: Connect to the server, specify the address and port. Send commands to the server using the command name and parameters. Use the HELP command to view help information for a specific command. Use the QUIT command to exit the command line tool.
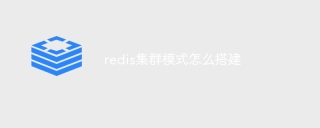
Redis cluster mode deploys Redis instances to multiple servers through sharding, improving scalability and availability. The construction steps are as follows: Create odd Redis instances with different ports; Create 3 sentinel instances, monitor Redis instances and failover; configure sentinel configuration files, add monitoring Redis instance information and failover settings; configure Redis instance configuration files, enable cluster mode and specify the cluster information file path; create nodes.conf file, containing information of each Redis instance; start the cluster, execute the create command to create a cluster and specify the number of replicas; log in to the cluster to execute the CLUSTER INFO command to verify the cluster status; make


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
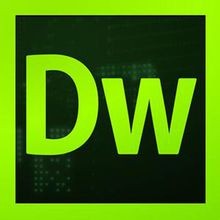
Dreamweaver CS6
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 Linux new version
SublimeText3 Linux latest version

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
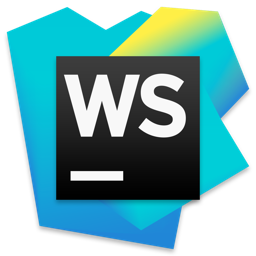
WebStorm Mac version
Useful JavaScript development tools